Angular Directives
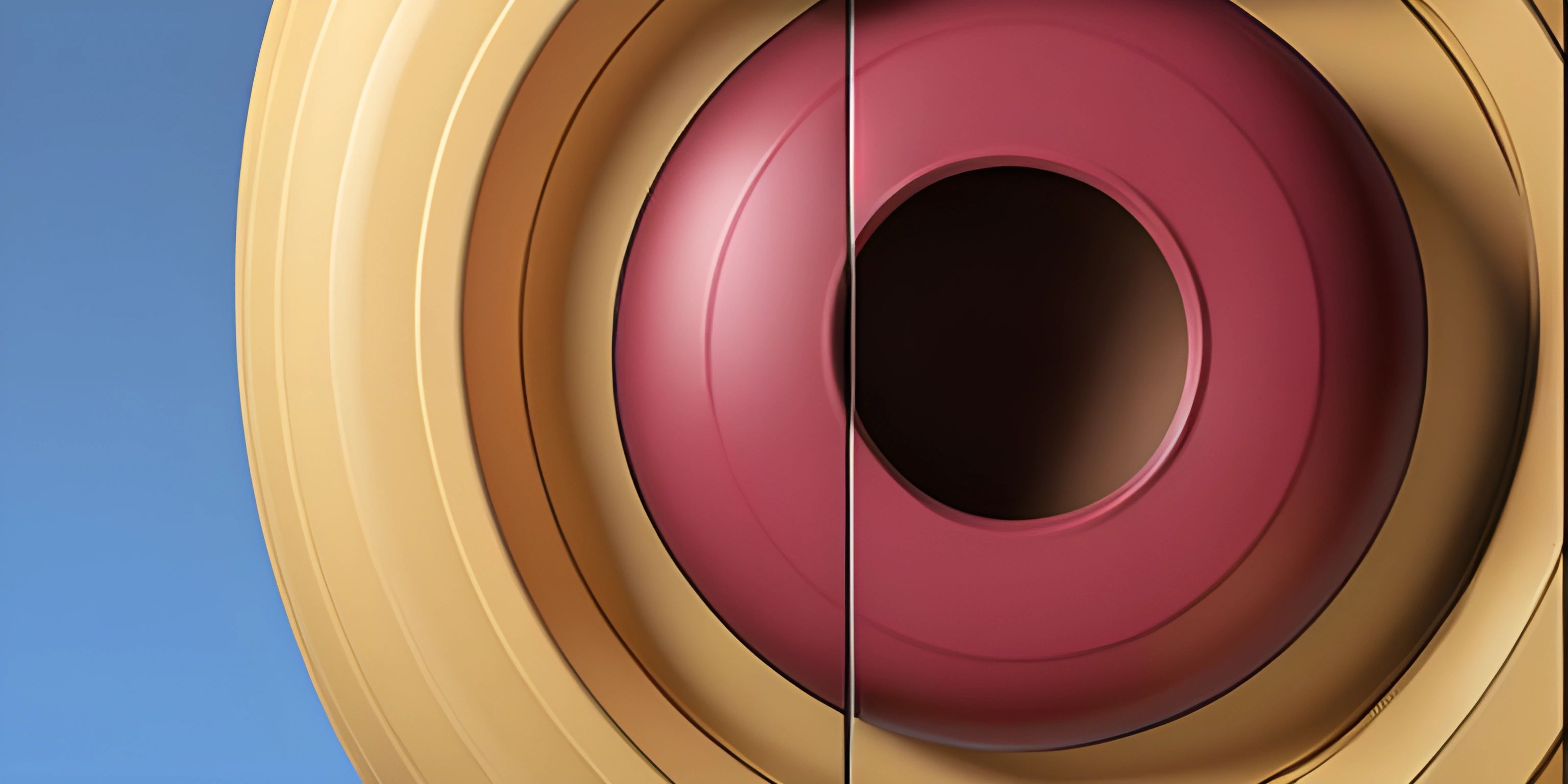
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Angular is a powerful web development framework, and one of its key features is directives. Directives are a way to manipulate the DOM (Document Object Model) and enhance its functionality. They're basically markers on a DOM element that tell Angular to attach a specific behavior or transform the DOM element and its children.
Built-In Directives
Angular comes with a set of pre-built directives that you can use right out of the box. Let's take a look at some of the most common ones:
ngIf
*ngIf
is a structural directive that conditionally adds or removes elements from the DOM based on a given expression. Here's an example:
<div *ngIf="isLoggedIn"> Welcome back, user! </div>
In this example, the div
element will only be added to the DOM if isLoggedIn
is true.
ngFor
*ngFor
is another structural directive that's used to loop through a collection and create DOM elements for each item. Here's an example:
<ul> <li *ngFor="let item of items">{{ item }}</li> </ul>
In this case, a li
element will be created for each item in the items
array, and the content of the li
element will be the item's value.
ngClass
[ngClass]
is an attribute directive that dynamically adds or removes CSS classes from a DOM element based on an expression. Here's a simple example:
<button [ngClass]="{ 'active': isActive }">Toggle</button>
The active
CSS class will be added to the button
element if isActive
is true, and removed if it's false.
Custom Directives
In addition to the built-in directives, you can also create your own custom directives in Angular. To create a custom directive, you need to define a class with the @Directive
decorator. Here's an example of a simple custom directive:
import { Directive, ElementRef } from '@angular/core'; @Directive({ selector: '[appHighlight]' }) export class HighlightDirective { constructor(private elementRef: ElementRef) { elementRef.nativeElement.style.backgroundColor = 'yellow'; } }
In this example, we're creating a directive called appHighlight
that sets the background color of the element it's applied to, to yellow. To use this directive, you simply add appHighlight
as an attribute to any DOM element:
<p appHighlight>Hello, Angular!</p>
The paragraph element will now have a yellow background.
Conclusion
Directives are a powerful feature in Angular that allows you to manipulate the DOM and add functionality to your web applications. By understanding how to use built-in directives and create your own custom directives, you can greatly enhance the capabilities of your Angular projects.
FAQ
What are Angular directives?
Angular directives are markers on DOM elements that tell Angular to attach specific behaviors or transform the DOM element and its children. They're used to manipulate the DOM and enhance functionality in Angular applications.
What are some built-in Angular directives?
Some common built-in Angular directives include *ngIf
(conditionally adds or removes elements from the DOM), *ngFor
(loops through a collection and creates DOM elements for each item), and [ngClass]
(dynamically adds or removes CSS classes from a DOM element based on an expression).
How do you create a custom Angular directive?
To create a custom Angular directive, you need to define a class with the @Directive
decorator and provide a selector. Inside the class, you can manipulate the DOM element by injecting ElementRef and using its nativeElement property. After creating the custom directive, you can use it as an attribute on any DOM element.