JavaScript DOM Manipulation
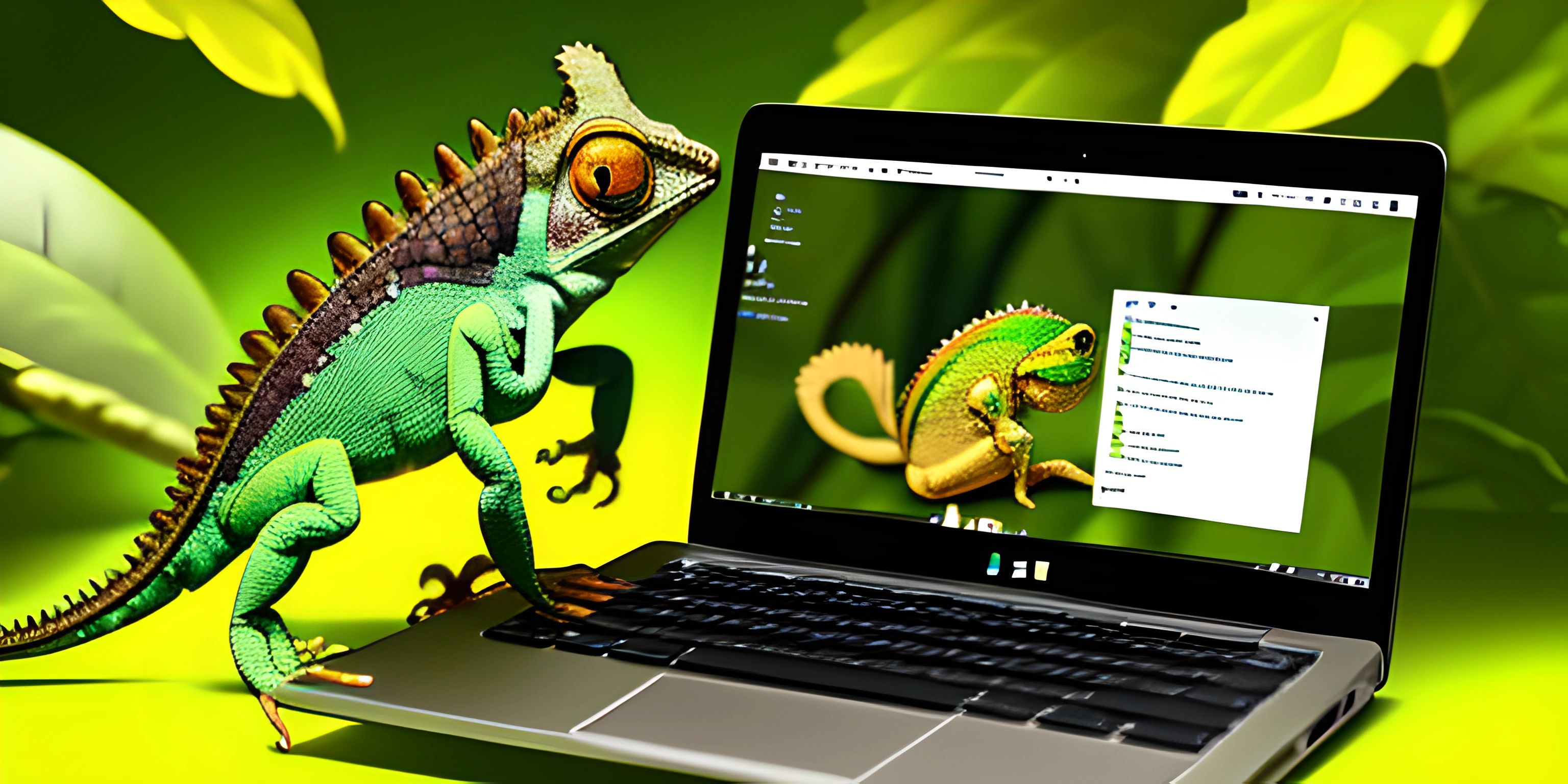
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
When working with web development, interacting with the Document Object Model (DOM) is an essential skill. The DOM represents the structure of a web page, and JavaScript can be used to manipulate it, allowing for dynamic changes to the content and appearance of a page. Buckle up and get ready to dive into the world of DOM manipulation using JavaScript!
Selecting Elements
The first step in DOM manipulation is selecting the elements we want to work with. JavaScript provides several methods to achieve this:
getElementById
To select an element with a specific id
, we can use the getElementById
method:
const myElement = document.getElementById("my-element-id");
querySelector and querySelectorAll
To select elements based on CSS selectors, we can use querySelector
(for a single element) and querySelectorAll
(for multiple elements):
// Selects the first occurrence of the element with the class 'my-class' const singleElement = document.querySelector(".my-class"); // Selects all elements with the class 'my-class' const allElements = document.querySelectorAll(".my-class");
Modifying Content
Once we have the elements we want to work with, we can modify their content or attributes.
InnerHTML and TextContent
To modify an element's content, we can use the innerHTML
or textContent
properties:
const myElement = document.getElementById("my-element-id"); // Change the HTML content myElement.innerHTML = "<strong>Hello, World!</strong>"; // Change the text content (without HTML tags) myElement.textContent = "Hello, World!";
Attributes
To manipulate an element's attributes, we can use the setAttribute
and removeAttribute
methods:
const myElement = document.getElementById("my-element-id"); // Set an attribute myElement.setAttribute("data-custom-attribute", "some-value"); // Remove an attribute myElement.removeAttribute("data-custom-attribute");
Event Handling
To make a web page interactive, we need to handle user events like clicks, mouse movements, and keyboard inputs. JavaScript provides event listeners for this purpose:
addEventListener
To attach an event listener to an element, use the addEventListener
method:
const myButton = document.querySelector("#my-button"); myButton.addEventListener("click", function () { console.log("Button clicked!"); });
removeEventListener
To remove an event listener added with addEventListener
, we need to pass the same function to removeEventListener
. To do this, we can define the function first and then pass it:
const myButton = document.querySelector("#my-button"); function handleClick() { console.log("Button clicked!"); } myButton.addEventListener("click", handleClick); myButton.removeEventListener("click", handleClick);
Now you're well-equipped to start manipulating the DOM using JavaScript! Remember to experiment, practice, and explore the many other methods and features available. Happy coding!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Using JavaScript (psst, it's free!).
FAQ
What is DOM manipulation in JavaScript?
DOM manipulation in JavaScript refers to the process of interacting with the Document Object Model (DOM) using JavaScript code. This includes tasks like selecting elements on the page, modifying their content, attributes, or styles, and handling user interactions through event listeners.
How can I select an element using JavaScript?
To select an element using JavaScript, you can use various methods like getElementById
, getElementsByClassName
, getElementsByTagName
, querySelector
, and querySelectorAll
. For instance, to select an element with the ID "myElement", you would use:
const myElement = document.getElementById("myElement");
How do I modify an element's content or attributes with JavaScript?
To modify an element's content or attributes, you can use properties like innerText
, innerHTML
, textContent
, style
, and setAttribute
. Here's an example that changes the text content and adds a class to an element with the ID "myElement":
const myElement = document.getElementById("myElement"); myElement.textContent = "New text content"; myElement.setAttribute("class", "new-class");
How can I add or remove an element using JavaScript?
To add or remove elements using JavaScript, you can use methods like createElement
, appendChild
, insertBefore
, removeChild
, and replaceChild
. Here's an example that creates a new paragraph element, adds it to the DOM, and later removes it:
// Create a new paragraph element const newParagraph = document.createElement("p"); newParagraph.textContent = "This is a new paragraph."; // Add the new paragraph to the DOM const container = document.getElementById("container"); container.appendChild(newParagraph); // Remove the paragraph from the DOM container.removeChild(newParagraph);
How do I handle user events with JavaScript DOM manipulation?
To handle user events, you can use the addEventListener
and removeEventListener
methods. Here's an example that listens for a click event on a button with the ID "myButton" and calls a function named handleClick
when the button is clicked:
const myButton = document.getElementById("myButton"); myButton.addEventListener("click", handleClick); function handleClick() { alert("Button clicked!"); }