Creating Custom Artwork Components with Java Swing
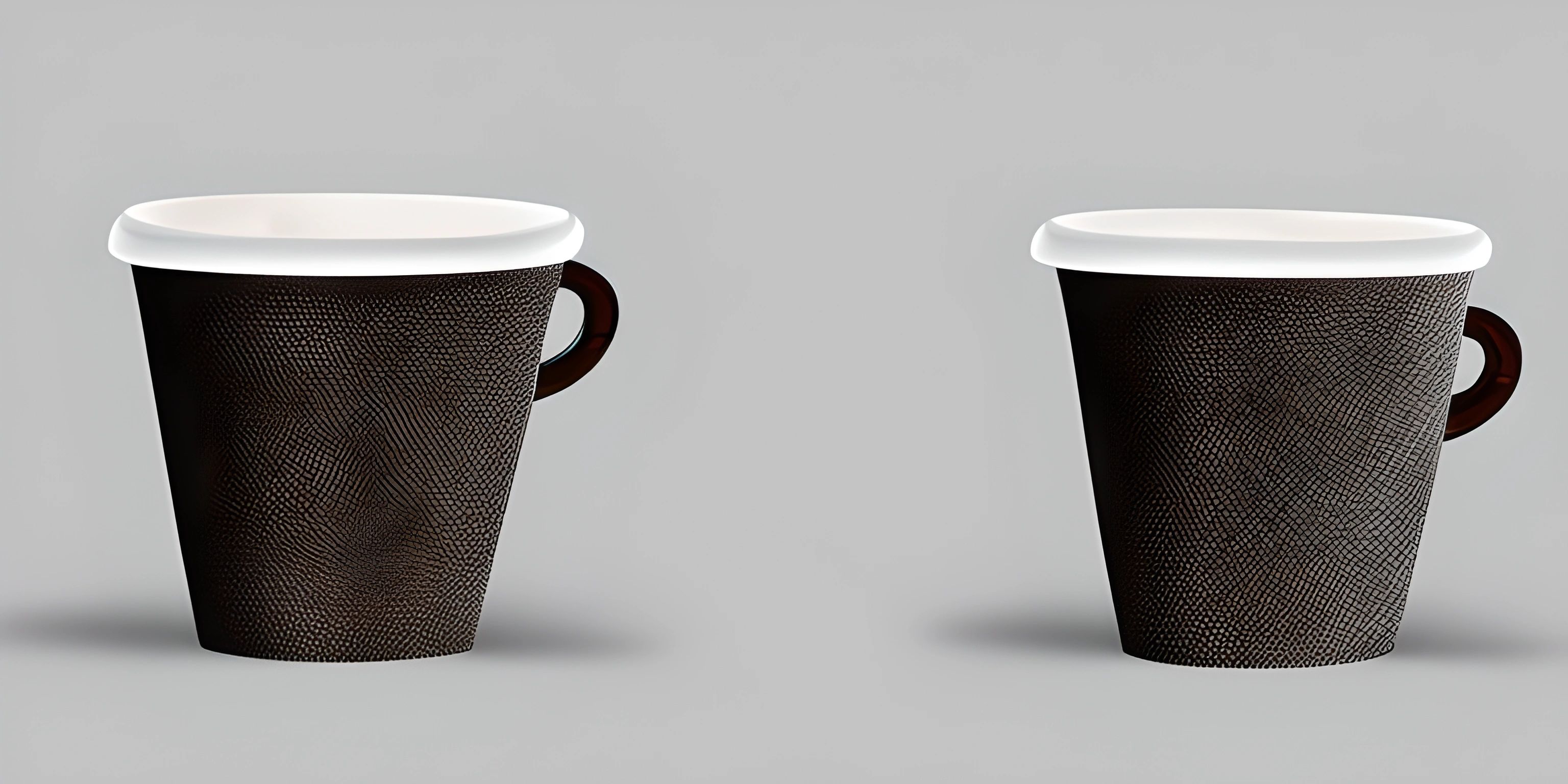
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Java is a popular programming language, and Swing is a powerful library that provides an extensive set of tools for creating graphical user interfaces (GUIs). One great feature of Swing is its ability to create custom artwork components. This opens the door to a world of creativity and flexibility, allowing you to design unique components that fit your specific needs.
Getting Started with Swing
Before diving into custom artwork components, you should familiarize yourself with the Java Swing library. Swing is part of the Java Foundation Classes (JFC) and is built on top of the Abstract Window Toolkit (AWT). It provides a rich set of components and tools for creating GUIs in Java.
To start using Swing in your project, make sure to import the necessary Swing packages:
import javax.swing.*; import java.awt.*;
Custom Artwork Components
A custom artwork component is a Swing component that can draw unique shapes, images, or any other visual element you can imagine. To create a custom component, you will need to extend an existing Swing component, typically JComponent
, and override its paintComponent(Graphics g)
method to draw your desired graphics.
Creating a Custom Component Class
To create a custom component, first extend the JComponent
class:
import javax.swing.*; import java.awt.*; public class CustomArtworkComponent extends JComponent { // Custom component code goes here }
Next, override the paintComponent(Graphics g)
method. This method is responsible for drawing the component on the screen:
import javax.swing.*; import java.awt.*; public class CustomArtworkComponent extends JComponent { @Override protected void paintComponent(Graphics g) { super.paintComponent(g); // Custom drawing code goes here } }
Drawing Graphics
In the paintComponent(Graphics g)
method, you can use the Graphics
object to draw shapes, images, and text. The Graphics
class provides various drawing methods, such as drawLine
, drawRect
, drawOval
, and drawString
.
Here's an example of how to draw a simple smiley face using a custom component:
import javax.swing.*; import java.awt.*; public class SmileyFaceComponent extends JComponent { @Override protected void paintComponent(Graphics g) { super.paintComponent(g); // Draw the face g.setColor(Color.YELLOW); g.fillOval(10, 10, 200, 200); // Draw the eyes g.setColor(Color.BLACK); g.fillOval(60, 60, 40, 40); g.fillOval(160, 60, 40, 40); // Draw the mouth g.drawArc(60, 90, 100, 100, 0, -180); } }
Adding the Custom Component to a Frame
To display your custom component, you will need to add it to a JFrame
. Here's an example of creating a simple Swing application that displays the smiley face custom component:
import javax.swing.*; public class CustomArtworkDemo { public static void main(String[] args) { SwingUtilities.invokeLater(() -> { JFrame frame = new JFrame("Custom Artwork Component"); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.setSize(300, 300); frame.add(new SmileyFaceComponent()); frame.setVisible(true); }); } }
When you run the application, you will see a window displaying your custom smiley face component.
Conclusion
Creating custom artwork components in Java Swing unlocks a world of creativity for your GUI applications. By extending existing Swing components and overriding their painting methods, you can design unique and visually appealing components tailored to your needs. Practice drawing various shapes and images to get a feel for what's possible, and let your imagination run wild!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Web Frameworks (React) (psst, it's free!).
FAQ
What is Java Swing and why should I use it for creating custom artwork components?
Java Swing is a widely-used graphical user interface (GUI) library for creating rich, interactive desktop applications in Java. It provides a comprehensive set of pre-built components and allows you to create custom components for your applications. When it comes to creating custom artwork components, Swing offers several advantages, such as:
- A rich set of drawing tools and functionalities
- Event handling for user interactions
- Support for custom rendering and painting
- Cross-platform compatibility
How do I start creating custom components with Java Swing?
To create custom components in Java Swing, follow these steps:
- Extend the
JComponent
class or any other suitable Swing component class. - Override the
paintComponent(Graphics g)
method to provide custom rendering for your component. - Use the
Graphics
object passed to thepaintComponent
method to draw your custom artwork. - Create an instance of your custom component and add it to a container (e.g.,
JFrame
orJPanel
) to display it in your application. Here's an example of a simple custom component that draws a rectangle:
import javax.swing.*; import java.awt.*; public class CustomComponent extends JComponent { @Override protected void paintComponent(Graphics g) { super.paintComponent(g); g.setColor(Color.BLUE); g.fillRect(50, 50, 100, 100); } public static void main(String[] args) { JFrame frame = new JFrame("Custom Component Example"); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.add(new CustomComponent()); frame.setSize(300, 300); frame.setVisible(true); } }
How can I handle user interactions with my custom artwork components in Java Swing?
Java Swing provides various event listeners and adapters to handle different types of user interactions, such as mouse clicks, keyboard inputs, and focus changes. To handle user interactions with your custom component, follow these steps:
- Implement the appropriate listener interface (e.g.,
MouseListener
,KeyListener
) or extend the corresponding adapter class (e.g.,MouseAdapter
,KeyAdapter
). - Override the relevant event handling methods to define the behavior of your component in response to user actions.
- Register the listener to your custom component using the
addXxxListener
method (e.g.,addMouseListener
,addKeyListener
). Here's an example of handling mouse clicks on a custom component:
import javax.swing.*; import java.awt.*; import java.awt.event.MouseAdapter; import java.awt.event.MouseEvent; public class CustomComponentWithMouseListener extends JComponent { @Override protected void paintComponent(Graphics g) { super.paintComponent(g); g.setColor(Color.BLUE); g.fillRect(50, 50, 100, 100); } public CustomComponentWithMouseListener() { addMouseListener(new MouseAdapter() { @Override public void mouseClicked(MouseEvent e) { System.out.println("Mouse clicked at (" + e.getX() + ", " + e.getY() + ")"); } }); } public static void main(String[] args) { JFrame frame = new JFrame("Custom Component With Mouse Listener"); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.add(new CustomComponentWithMouseListener()); frame.setSize(300, 300); frame.setVisible(true); } }
How can I make my custom artwork components resizable with Java Swing?
To make your custom components resizable with Java Swing, you need to make sure that your component's painting code adapts to the current size of the component. You can achieve this by using the getWidth()
and getHeight()
methods to get the current dimensions of your component and adjust the drawing coordinates and dimensions accordingly.
Here's an example of a custom component that draws a centered square, which resizes with the component:
import javax.swing.*; import java.awt.*; public class ResizableCustomComponent extends JComponent { @Override protected void paintComponent(Graphics g) { super.paintComponent(g); int width = getWidth(); int height = getHeight(); int squareSize = Math.min(width, height) / 2; int x = (width - squareSize) / 2; int y = (height - squareSize) / 2; g.setColor(Color.BLUE); g.fillRect(x, y, squareSize, squareSize); } public static void main(String[] args) { JFrame frame = new JFrame("Resizable Custom Component"); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.add(new ResizableCustomComponent()); frame.setSize(300, 300); frame.setVisible(true); } }