How Assemblers Work: Converting Assembly Language to Machine Code
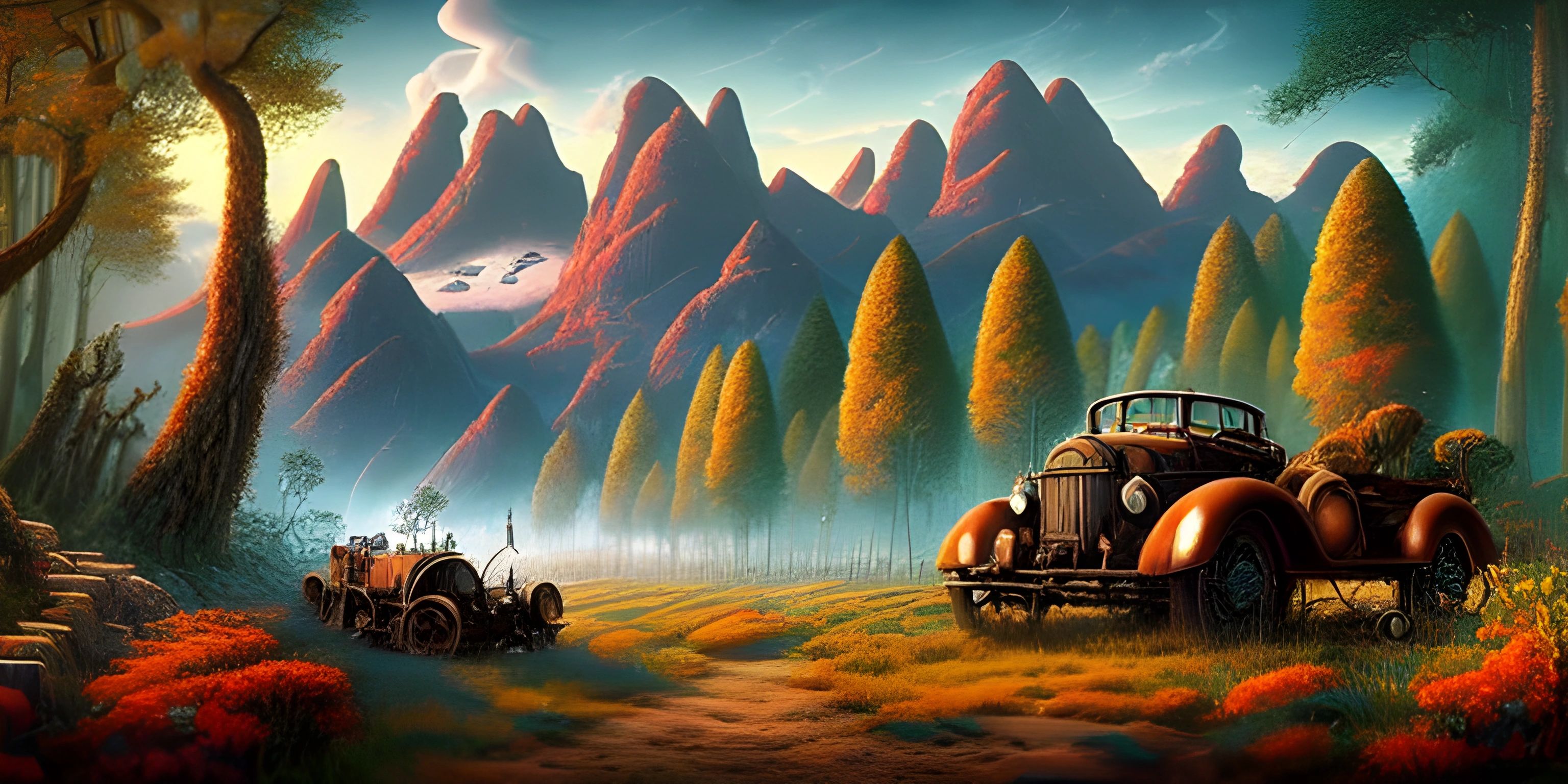
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
When it comes to programming, most of us are used to high-level languages like Python or JavaScript, where we write code that is relatively easy to read and understand. But what about those languages that are closer to the hardware, like assembly language? That's where assemblers come in. Let's take a deep dive into the world of assemblers and explore how they convert assembly language into machine code.
What is Assembly Language?
Assembly language is a low-level programming language that is one step above machine code. It uses mnemonic codes and labels to represent machine-level instructions, making it slightly more human-readable. Each assembly language instruction corresponds directly to a machine code instruction, which is executed by the CPU.
For example, here’s a simple assembly language snippet for an x86 processor:
MOV AX, 1 ; Move the value 1 into the AX register ADD AX, 2 ; Add 2 to the value in the AX register
The above code moves the value 1 into the AX register and then adds 2 to it. In machine code, these instructions would be represented by binary numbers, which are not as easy for humans to interpret.
What is an Assembler?
An assembler is a specialized program that translates assembly language into machine code. Think of it as a translator that speaks both human (well, almost human) and machine languages fluently. The assembler reads the assembly code, translates each line into its corresponding machine code, and then outputs a binary file that the computer can execute.
The Assembly Process
The process of translating assembly language into machine code involves several steps:
- Lexical Analysis: The assembler reads the assembly code and breaks it down into tokens. Tokens are the smallest units of meaning in the code, such as keywords, labels, and operators.
- Syntax Analysis: The assembler checks the tokens for grammatical correctness. It ensures that the code follows the syntax rules of the assembly language.
- Semantic Analysis: The assembler evaluates the meaning of the code. This includes resolving labels and variables to their memory addresses.
- Code Generation: The assembler translates the assembly instructions into machine code instructions.
- Optimization: Some assemblers include an optimization step, where they improve the performance of the generated machine code.
- Output: The assembler produces the final machine code file, which can be executed by the computer.
Assemblers vs. Compilers
You might be wondering how assemblers differ from compilers. While both translate code from one language to another, compilers work with high-level languages like C++ or Java and convert them into assembly language or directly into machine code. Assemblers, on the other hand, work exclusively with assembly language and translate it into machine code.
Types of Assemblers
Assemblers come in different flavors, each tailored to specific types of processors and architectures. Here are a few common types:
Single-pass Assemblers
Single-pass assemblers read the source code once and generate the machine code in a single pass. They are fast but have limitations, such as not being able to resolve forward references (labels that are used before they are defined).
Multi-pass Assemblers
Multi-pass assemblers read the source code multiple times. The first pass collects information, such as labels and addresses, and the subsequent passes generate the machine code. This allows them to resolve forward references and produce more optimized code.
Cross Assemblers
Cross assemblers run on one type of processor but generate code for a different type. They are used in cross-platform development and embedded systems programming.
Practical Example: Assembling x86 Code
Let's assemble a simple x86 assembly code example to see how an assembler works in practice. We’ll use the NASM (Netwide Assembler), a popular assembler for x86 architecture.
Source Code
Here's a simple NASM assembly program that prints "Hello, World!" to the console:
section .data hello db 'Hello, World!',0 section .text global _start _start: ; Write Hello, World! to the console mov eax, 4 ; syscall number for sys_write mov ebx, 1 ; file descriptor 1 (stdout) mov ecx, hello ; pointer to the hello string mov edx, 13 ; length of the string int 0x80 ; call the kernel ; Exit program mov eax, 1 ; syscall number for sys_exit xor ebx, ebx ; return code 0 int 0x80 ; call the kernel
Assembling the Code
To assemble this code using NASM, we would run the following command:
nasm -f elf64 hello.asm
This converts the assembly code into an object file (hello.o
). To link the object file and create an executable, we would use a linker like ld
:
ld -s -o hello hello.o
Finally, we can run our program:
./hello
This should print "Hello, World!" to the console. The assembler has successfully translated our assembly code into executable machine code.
Debugging Assembly Code
Debugging assembly code can be challenging due to its low-level nature. Tools like GDB (GNU Debugger) can be invaluable for stepping through the code and inspecting registers and memory. To debug the hello
program, we could use the following commands:
gdb ./hello
Inside GDB, we can set breakpoints, step through instructions, and examine the state of the CPU and memory.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust - A Language You'll Love (psst, it's free!).
FAQ
What is assembly language?
Assembly language is a low-level programming language that uses mnemonic codes and labels to represent machine-level instructions. Each assembly instruction corresponds directly to a machine code instruction.
What is the role of an assembler?
An assembler translates assembly language into machine code. It reads the assembly code, translates each instruction into its corresponding machine code, and outputs a binary file that the computer can execute.
How do single-pass and multi-pass assemblers differ?
Single-pass assemblers read the source code once and generate machine code in one pass, making them fast but limited in resolving forward references. Multi-pass assemblers read the code multiple times, allowing them to resolve forward references and produce more optimized code.
Can assemblers optimize machine code?
Yes, some assemblers include an optimization step to improve the performance of the generated machine code. However, the extent of optimization is generally less compared to high-level language compilers.
What are cross assemblers used for?
Cross assemblers generate code for a different type of processor than the one they run on. They are commonly used in cross-platform development and embedded systems programming.