Introduction to NASM Assembler
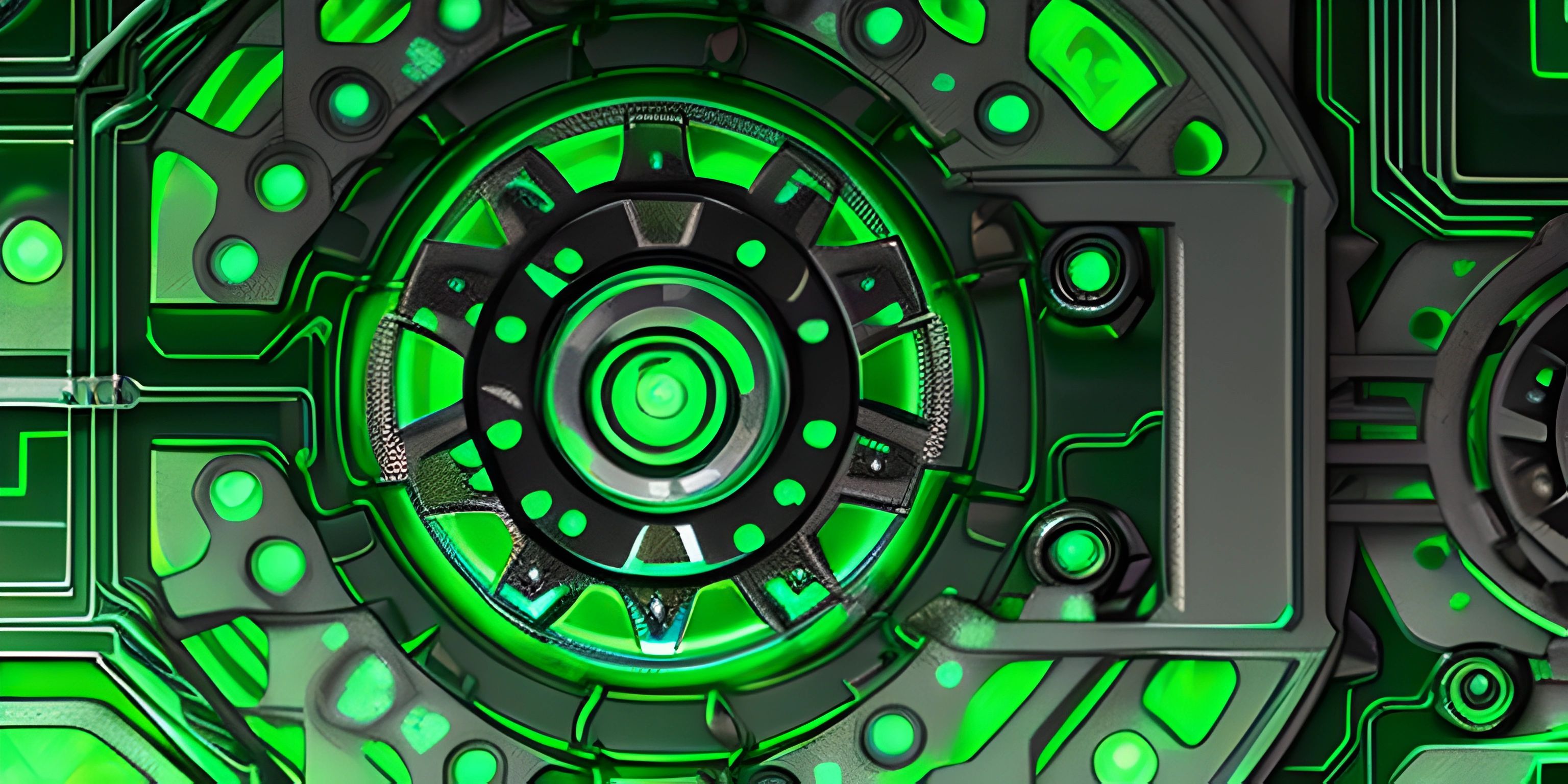
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
When diving into the world of low-level programming, assembly language is the go-to choice for many developers. One popular assembler for x86 and x86-64 processors is the Netwide Assembler (NASM). With its unique features and powerful capabilities, NASM has earned its place in the hearts of assembly language enthusiasts.
What is NASM?
NASM is an open-source assembler that converts assembly language code into machine code for x86 and x86-64 processors. It's widely used for tasks such as operating system development, driver creation, and reverse engineering. NASM provides a simple syntax that closely resembles Intel's assembly language while offering compatibility with a variety of output formats, such as ELF, COFF, and Mach-O.
Why use NASM?
Several factors contribute to the popularity of NASM among assembly language programmers:
- Intel-like syntax: NASM uses a syntax similar to Intel's assembly language, making it easier to learn and understand for those already familiar with Intel's convention.
- Multiple output formats: NASM supports a wide range of output formats, giving developers the flexibility to choose the most suitable option for their projects.
- Cross-platform support: NASM works on multiple platforms, including Windows, macOS, and Linux, ensuring a consistent development experience regardless of the operating system.
- Macro support: NASM includes a powerful macro processor to simplify repetitive tasks and streamline the development process.
- Detailed documentation: NASM offers comprehensive documentation, providing clear explanations and examples for its features and syntax.
Setting up NASM
Before we can start writing NASM assembly code, we need to install the assembler on our system. Here are the installation steps for different platforms:
Windows
- Download the latest NASM release for Windows from the official website.
- Extract the contents of the downloaded ZIP file to a folder on your system.
- Add the extracted folder to your system's PATH environment variable.
macOS
Use Homebrew to install NASM:
brew install nasm
Linux
Use your distribution's package manager to install NASM. For example, on Ubuntu or Debian-based systems, run:
sudo apt-get install nasm
On Fedora or CentOS, use:
sudo dnf install nasm
Writing your first NASM program
Now that NASM is installed, let's write a simple "Hello, World!" program to demonstrate its usage.
Create a new file called hello.asm
and add the following code:
section .data hello db "Hello, World!", 0 section .text global _start _start: ; Write the string to stdout mov eax, 4 mov ebx, 1 lea ecx, [hello] mov edx, 13 int 0x80 ; Exit the program mov eax, 1 xor ebx, ebx int 0x80
To assemble and link the program, run the following commands:
nasm -f elf64 hello.asm ld -o hello hello.o
Now you can execute the hello
binary to see the "Hello, World!" message printed to the console.
Conclusion
NASM is a powerful and versatile assembler that simplifies assembly language programming for x86 and x86-64 processors. With its easy-to-understand syntax, cross-platform support, and robust feature set, NASM is an excellent choice for low-level programming tasks. Whether you're developing an operating system or writing a device driver, NASM has you covered.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust - A Language You'll Love (psst, it's free!).
FAQ
What is NASM Assembler and what is it used for?
NASM (Netwide Assembler) is an assembler and disassembler for x86 and x86-64 processors. It's used for writing low-level machine code programs in assembly language, which can be executed directly by the computer's hardware. NASM is popular for its flexibility, simplicity, and powerful macro support, making it an ideal choice for programmers working on system-level code or other projects requiring fine-grained control over hardware.
How is NASM different from other assemblers?
NASM stands out from other assemblers for a few reasons:
- It has a consistent syntax that's easy to learn and understand.
- It supports a wide range of output formats, such as ELF, COFF, and Mach-O, making it suitable for various platforms and operating systems.
- NASM's macro support allows for complex code generation and simplification of repetitive tasks.
- It's an open-source project, which means it's constantly improving and benefiting from a large community of users and contributors.
Can you provide a simple example of NASM code?
Sure! Here's a basic example of NASM code that prints "Hello, World!" to the console:
section .data hello db "Hello, World!", 0xA section .text global _start _start: ; Write "Hello, World!" to the console mov eax, 4 mov ebx, 1 lea ecx, [hello] mov edx, 13 int 0x80 ; Exit the program mov eax, 1 xor ebx, ebx int 0x80
This code defines a data section containing the "Hello, World!" string, and a text section with the _start
label, which is the entry point of the program. It uses x86 assembly instructions to write the string to the console and then exit the program.
How do I assemble and link a NASM program?
To assemble and link a NASM program, you'll need to follow these steps:
- First, assemble the source code using NASM. For example, if your source file is named
example.asm
, run the following command:
This will generate an object file namednasm -f elf example.asm
example.o
. - Next, link the object file using the linker, such as
ld
on Linux. For example:
This will create an executable file namedld -m elf_i386 -s -o example example.o
example
. - Finally, run the executable:
This will execute the compiled program and display the output, if any. Remember, these steps may vary slightly depending on your operating system and development environment../example