Elixir Testing: Ensuring Code Quality and Reliability
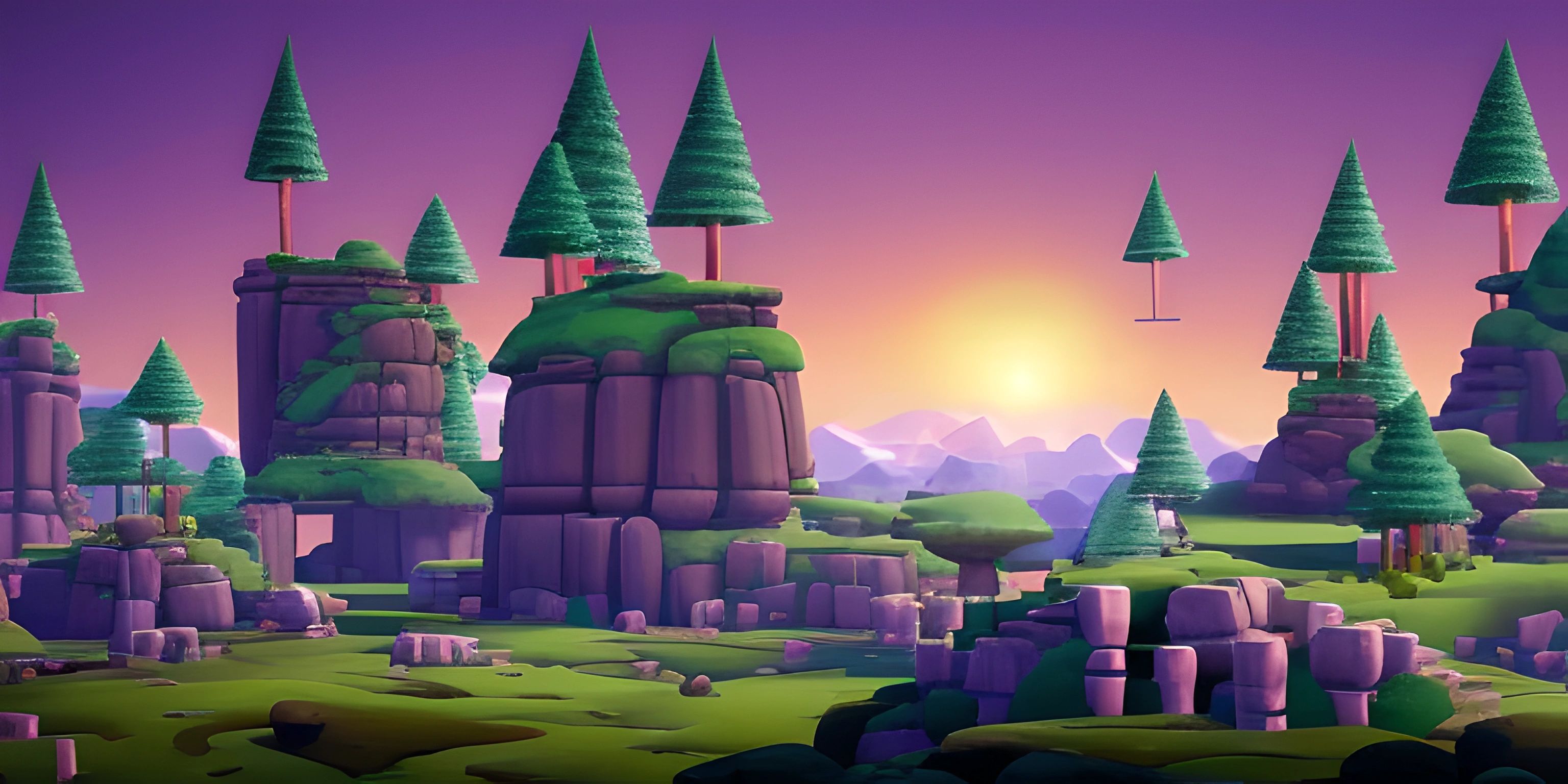
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Elixir, a functional language built on the Erlang VM, has gained popularity for its impressive performance, fault tolerance, and scalability. Just like every other programming language, testing is a crucial component in Elixir to ensure code quality and reliability.
Elixir comes with a built-in testing framework called ExUnit, which makes it easy to get started with testing your code.
Getting Started with ExUnit
To start using ExUnit in your Elixir project, you'll need to include the :ex_unit
module and use the ExUnit.Case
macro to define your test cases.
defmodule MyModuleTest do use ExUnit.Case # Your tests go here end
Now you can write test cases using the test
macro. Let's say you have a module called MyModule
with a greet/1
function that takes a name and returns a greeting message.
defmodule MyModule do def greet(name) do "Hello, #{name}!" end end
You can write a test case for this function like this:
defmodule MyModuleTest do use ExUnit.Case alias MyModule test "greet/1" do assert MyModule.greet("John") == "Hello, John!" end end
To run your tests, simply execute the mix test
command in your terminal.
Test-driven Development in Elixir
Test-driven development (TDD) is a software development methodology where you write tests before implementing the functionality. In Elixir, you can follow TDD by writing ExUnit tests before implementing the actual code.
Here's a TDD example. Suppose you want to create a function add/2
that adds two numbers. Start by writing a test case:
defmodule MyModuleTest do use ExUnit.Case alias MyModule test "add/2" do assert MyModule.add(1, 2) == 3 end end
Running the tests now will result in a failure, as the add/2
function is not yet implemented. Next, implement the add/2
function in your MyModule
:
defmodule MyModule do def add(a, b) do a + b end end
Now, when you run the tests again, the test should pass.
Testing with Fixtures
In some cases, you may need to set up some initial data or state before running your tests. ExUnit provides a feature called setup
that allows you to prepare your test environment.
defmodule MyModuleTest do use ExUnit.Case alias MyModule setup do # Perform setup here {:ok, some_data: "example"} end test "greet/1", context do IO.inspect(context[:some_data]) # "example" assert MyModule.greet("John") == "Hello, John!" end end
The setup
block is executed before each test, and the return value is passed as a map to the test through the context
argument.
Elixir testing with ExUnit is a powerful feature to ensure code quality and reliability. By following TDD practices and making use of fixtures, you can be confident that your Elixir code is robust, maintainable, and ready for production.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: AI Test Playground (psst, it's free!).