Unit Testing Best Practices
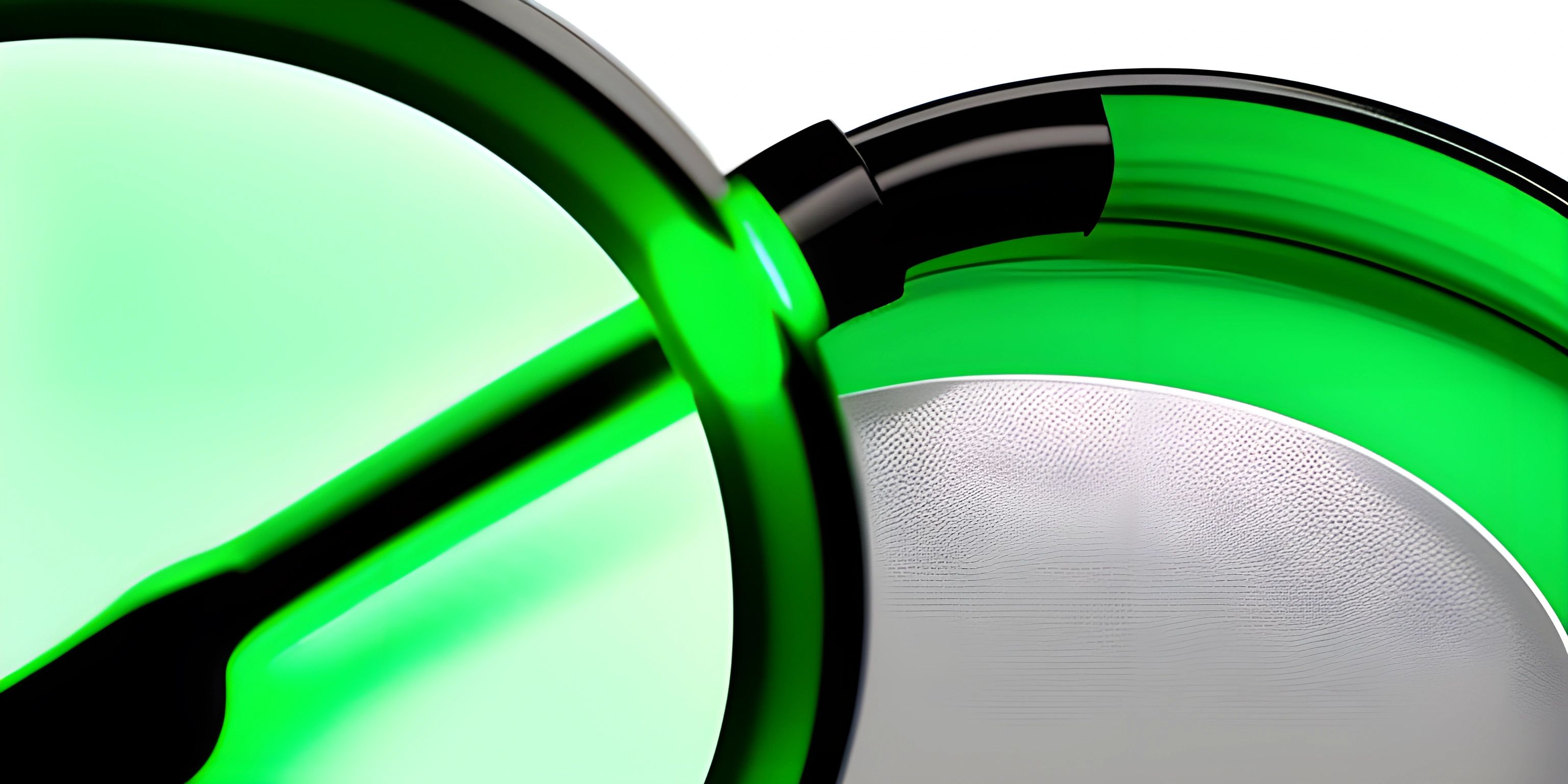
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Unit testing plays a crucial role in software development, ensuring that individual components of our code are working as expected. In this digital age, where even a single bug can cost companies millions of dollars or harm their reputation, it's critical that we follow best practices to create effective and maintainable unit tests.
The Importance of Unit Testing
Unit testing is a way to catch issues early in the development process, before they can escalate into more significant problems. The benefits of unit testing include:
-
Improved code quality: Unit tests help maintain a high level of code quality by making sure each component functions correctly and handles edge cases.
-
Easier debugging: When a bug is discovered, having a suite of unit tests makes it much easier to pinpoint the issue, saving valuable time and resources.
-
Simpler code refactoring: Unit tests provide a safety net when making changes to the code, ensuring that no previously working functionality is broken.
-
Faster development: Writing unit tests can seem time-consuming at first, but they ultimately save time by catching issues early and streamlining the debugging process.
Now that we understand why unit testing is so important, let's dive into some best practices for implementing it in our projects.
Unit Testing Best Practices
1. Write Testable Code
First and foremost, ensure your code is designed to be testable. This involves writing modular and loosely coupled code, adhering to the SOLID principles, and using dependency injection.
2. Use a Consistent Naming Convention
Choose a clear and consistent naming convention for your tests, such as FunctionName_TestCondition_ExpectedResult
. This makes it easier to identify the purpose of each test and quickly locate relevant tests when needed.
3. Keep Tests Simple and Focused
Each test should focus on a single aspect of your code. Avoid writing tests that depend on multiple components or complex setups, as this can make them brittle and difficult to maintain.
4. Test Edge Cases and Error Handling
Don't just test the "happy path" – also test edge cases and error handling. This helps uncover potential issues and ensures that your code can gracefully handle unexpected situations.
5. Use Mocks and Stubs Wisely
When testing components that rely on external dependencies, use mocks and stubs to isolate the code under test. This allows you to focus on testing your component's behavior without worrying about the dependencies' behavior.
6. Regularly Review and Refactor Tests
Just like your codebase, your tests need regular maintenance to stay effective. Periodically review and refactor your tests to ensure they remain clear, concise, and relevant.
7. Run Tests Frequently and Automatically
Integrate unit tests into your development workflow by running them frequently and automatically, ideally as part of a continuous integration process. This helps catch issues as soon as they're introduced, minimizing their impact.
By following these best practices, you'll be well on your way to creating a robust suite of unit tests that improve the quality and maintainability of your code.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: What Programming Means (psst, it's free!).
FAQ
Why is unit testing important in software development?
Unit testing is important because it improves code quality, makes debugging easier, simplifies code refactoring, and leads to faster development. It helps catch issues early in the development process, reducing the chances of more significant problems later on.
What are some best practices for implementing unit tests?
Some best practices include writing testable code, using a consistent naming convention, keeping tests simple and focused, testing edge cases and error handling, using mocks and stubs wisely, regularly reviewing and refactoring tests, and running tests frequently and automatically.
How can unit testing save time in the development process?
While writing unit tests may seem time-consuming initially, they save time in the long run by catching issues early, streamlining the debugging process, and providing a safety net when making changes to the code. This results in faster development and higher-quality software.