Testing and Quality Assurance
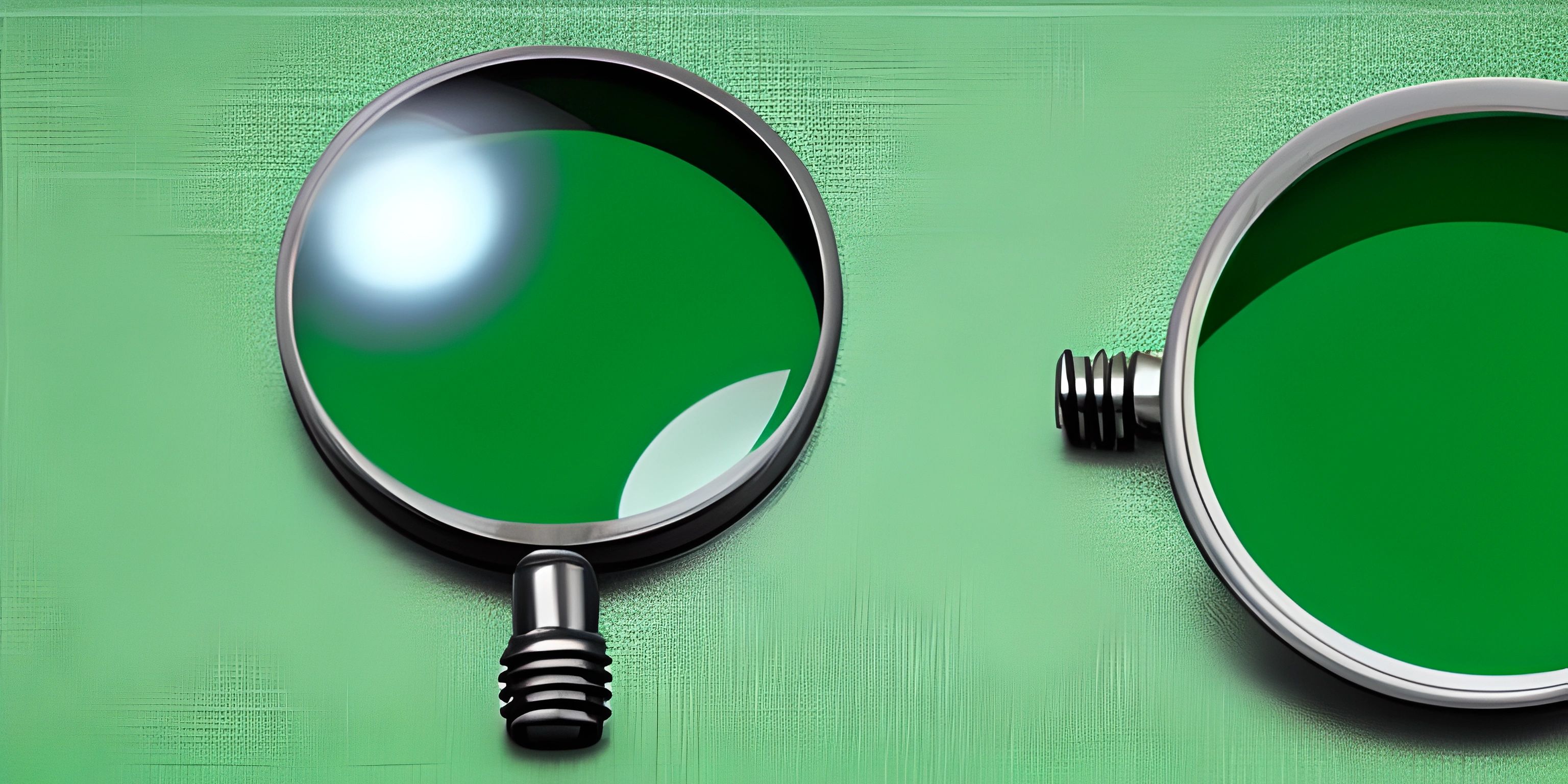
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Reliability and maintainability are essential aspects of writing good code. By implementing various testing techniques and quality assurance practices, you can ensure that your code performs well and is easy to maintain. This article will guide you through these techniques and practices, helping you improve the quality of your code.
What is Testing and Quality Assurance?
Testing is the process of evaluating a system or its components to ensure that it meets the specified requirements. Quality assurance (QA) is a set of activities focused on ensuring that the product meets the quality standards and end-user requirements. Together, testing and QA help ensure that your code is reliable, maintainable, and meets its intended purpose.
Types of Testing
There are several types of testing that can be applied to your code to ensure its quality:
- Unit Testing: Testing individual components or units of your code in isolation. This helps identify issues at the earliest stage of development.
function add(a, b) { return a + b; } // Unit test for the add function describe("add", function() { it("adds two numbers correctly", function() { expect(add(1, 2)).toBe(3); }); });
-
Integration Testing: Testing the interaction between multiple components or units of your code. This helps identify issues in the way components work together.
-
System Testing: Testing the entire system as a whole. This helps ensure that all components work together as expected and the system meets the specified requirements.
-
Acceptance Testing: Testing the system from a user's perspective. This helps ensure that the system meets the end-user requirements and is fit for its intended purpose.
Quality Assurance Practices
In addition to testing, there are several quality assurance practices that can help improve the reliability and maintainability of your code:
-
Code Reviews: Having your code reviewed by other developers can help identify issues, improve code quality, and ensure adherence to coding standards.
-
Coding Standards: Establishing and following coding standards for your project can help maintain consistency and readability, making it easier for others to understand and maintain your code.
-
Continuous Integration and Continuous Deployment (CI/CD): Implementing CI/CD practices can help catch issues early and ensure that your code is always in a releasable state.
-
Documentation: Providing clear documentation for your code can help others understand its purpose and how to use it, making it easier to maintain and extend.
-
Refactoring: Regularly reviewing and refactoring your code can help improve its structure, readability, and maintainability.
By incorporating these testing techniques and quality assurance practices into your development process, you can ensure that your code is reliable, maintainable, and meets the needs of its users.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Advanced Data Types (psst, it's free!).
FAQ
What is the main goal of testing and quality assurance in software development?
The main goal of testing and quality assurance in software development is to ensure that the code is reliable, maintainable, and performs as expected. This is achieved by implementing various testing techniques and quality assurance practices to identify and resolve issues, improve the overall quality of the code, and prevent defects from occurring in the future.
Can you briefly explain some common testing techniques in software development?
Sure! Here are a few common testing techniques used in software development:
- Unit testing: Testing individual components or units of code in isolation to ensure they function correctly.
- Integration testing: Testing how different components of the system work together to verify that the overall system functions as expected.
- System testing: Testing the complete system, including hardware, software, and all interactions, to ensure it meets the specified requirements.
- Acceptance testing: Ensuring the system meets the needs and expectations of the end-users and stakeholders.
How can quality assurance practices help improve code reliability and maintainability?
Quality assurance practices play a significant role in improving code reliability and maintainability. Some common practices include:
- Establishing coding standards and guidelines to ensure consistent code quality.
- Conducting regular code reviews to identify and resolve issues, share knowledge, and encourage collaboration.
- Implementing continuous integration and continuous delivery (CI/CD) processes to automate testing, building, and deploying code, ensuring quicker identification of problems and faster delivery of reliable software.
- Monitoring and measuring code quality metrics, like code coverage, complexity, and maintainability, to track improvements and set goals for future enhancements.
What is the importance of automated testing in the quality assurance process?
Automated testing is essential in the quality assurance process for several reasons:
- It speeds up the testing process, allowing developers to test their code more frequently and quickly identify and fix defects.
- It ensures consistency and repeatability in testing, reducing the risk of human errors and oversight.
- It allows developers to focus their efforts on more complex tasks and problem-solving, as the automated tests take care of routine checks.
- It enables continuous integration and continuous delivery, making it easier to maintain high-quality code in a fast-paced development environment.
How can developers strike a balance between thorough testing and efficient development?
Striking a balance between thorough testing and efficient development involves the following strategies:
- Prioritize testing efforts based on risk, focusing on critical components and functionalities first.
- Use a combination of manual and automated testing to maximize efficiency and coverage.
- Implement testing practices throughout the development process, ensuring that testing is not an afterthought or a separate phase.
- Encourage collaboration between developers, testers, and other stakeholders to foster a shared understanding of quality expectations and a culture of continuous improvement.