Greedy Algorithms
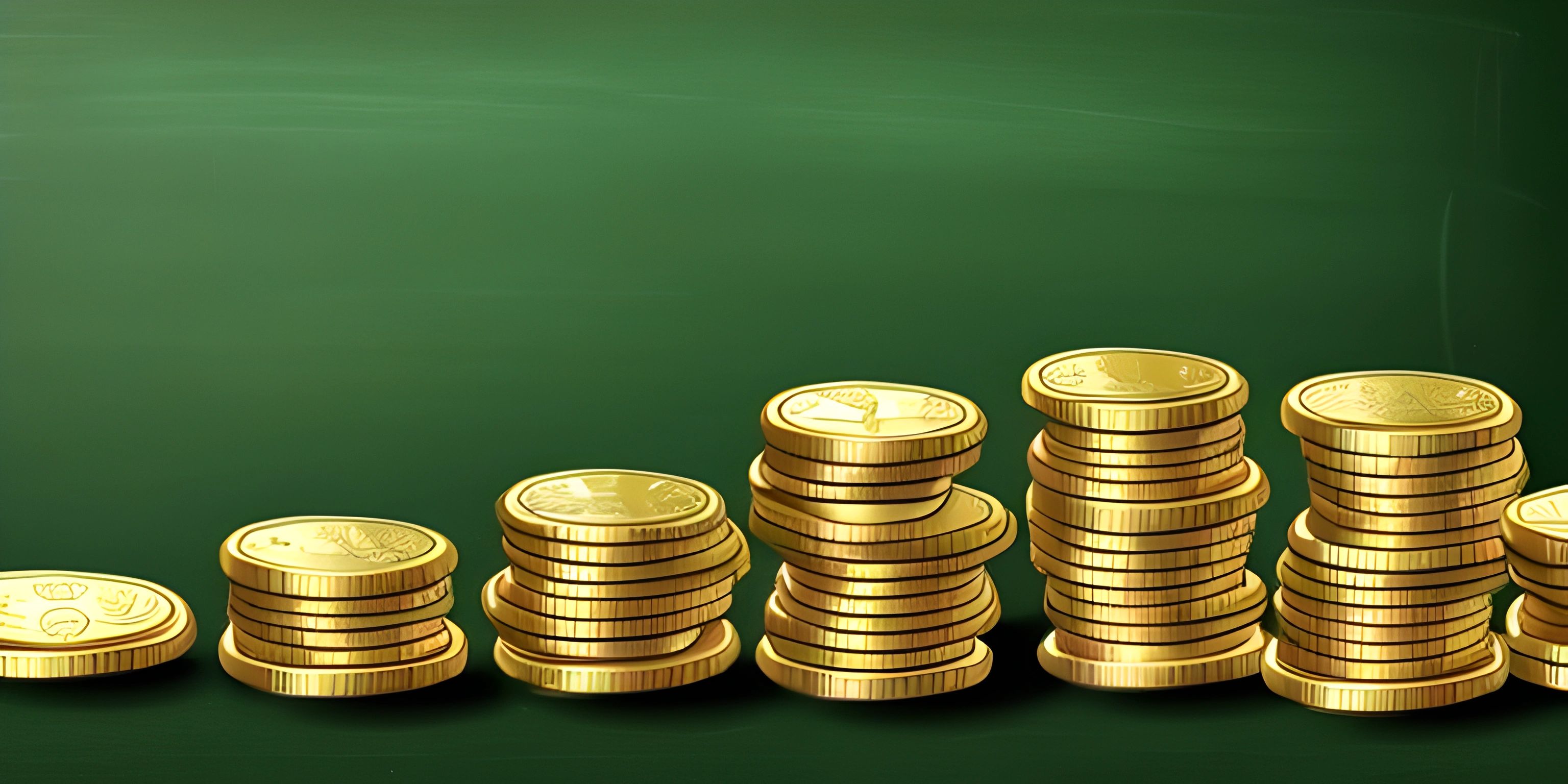
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Sometimes, the best way to solve a problem is to take a bite at a time. Greedy algorithms do exactly that! They take the best possible step at each stage, always choosing what seems to be the most beneficial at the time, without looking ahead or considering the overall problem. This strategy can lead to efficient and straightforward solutions for specific problem types.
What are Greedy Algorithms?
A greedy algorithm is an approach to solving optimization problems by making a locally optimal choice at each step, hoping that these choices will lead to a globally optimal solution. Greedy algorithms are often simple to implement and can offer quick results, especially when finding an exact solution is infeasible or unnecessary.
Examples of Greedy Algorithms
There are some well-known examples of greedy algorithms that showcase their effectiveness and adaptability to various situations:
-
Coin Change Problem: Given a set of coin denominations and a target amount, find the minimum number of coins needed to make the target amount. The greedy approach starts by selecting the largest denomination that is less than or equal to the target and continues to add coins until the target amount is reached.
-
Huffman Coding: Used in data compression, Huffman coding is a greedy algorithm that constructs an optimal prefix-free binary code for a set of symbols, given their frequency of occurrence.
-
Kruskal's Algorithm: This algorithm finds the minimum spanning tree of an undirected graph. It does so by sorting the edges by weight and iteratively adding them to the tree, as long as they don't form a cycle.
Pros and Cons
While greedy algorithms can be incredibly efficient and practical for certain problems, they also have their limitations:
Pros:
- Easy to implement
- Can be fast and efficient
- Suitable for real-time applications, where results are needed quickly
Cons:
- Not always optimal, as making locally optimal choices may lead to suboptimal solutions
- Can struggle with problems that require looking ahead, such as chess or more complex graph problems
Approaching Problems with Greedy Algorithms
When tackling a problem, it's essential to identify which algorithmic strategy will be most effective. To determine if a greedy approach is suitable, consider the following questions:
- Can the problem be broken down into smaller subproblems?
- Does a locally optimal choice always lead to a globally optimal solution?
- Can the problem be solved quickly and efficiently without considering the entire search space?
If the answer to these questions is yes, a greedy algorithm might be a good fit! But remember, it's always essential to double-check the results and make sure that the solution is indeed optimal.
Implementing a Greedy Algorithm
Let's look at a simple example of implementing a greedy algorithm to solve the coin change problem. We will use Python to demonstrate the solution:
def greedy_coin_change(denominations, target): denominations.sort(reverse=True) change = [] for coin in denominations: while target >= coin: target -= coin change.append(coin) return change denominations = [1, 5, 10, 25] target = 36 result = greedy_coin_change(denominations, target) print(result)
This code snippet will output [25, 10, 1]
, which indicates that we can make 36 with one 25-cent coin, one 10-cent coin, and one 1-cent coin.
However, keep in mind that greedy algorithms don't always guarantee an optimal solution. It's crucial to analyze the problem and determine if the greedy approach is suitable before diving in headfirst.
In conclusion, greedy algorithms can be a powerful tool in your problem-solving arsenal. Just remember, like a kid in a candy store, sometimes the best choice is to take it one step at a time!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Why Program? (psst, it's free!).