Knapsack Problem
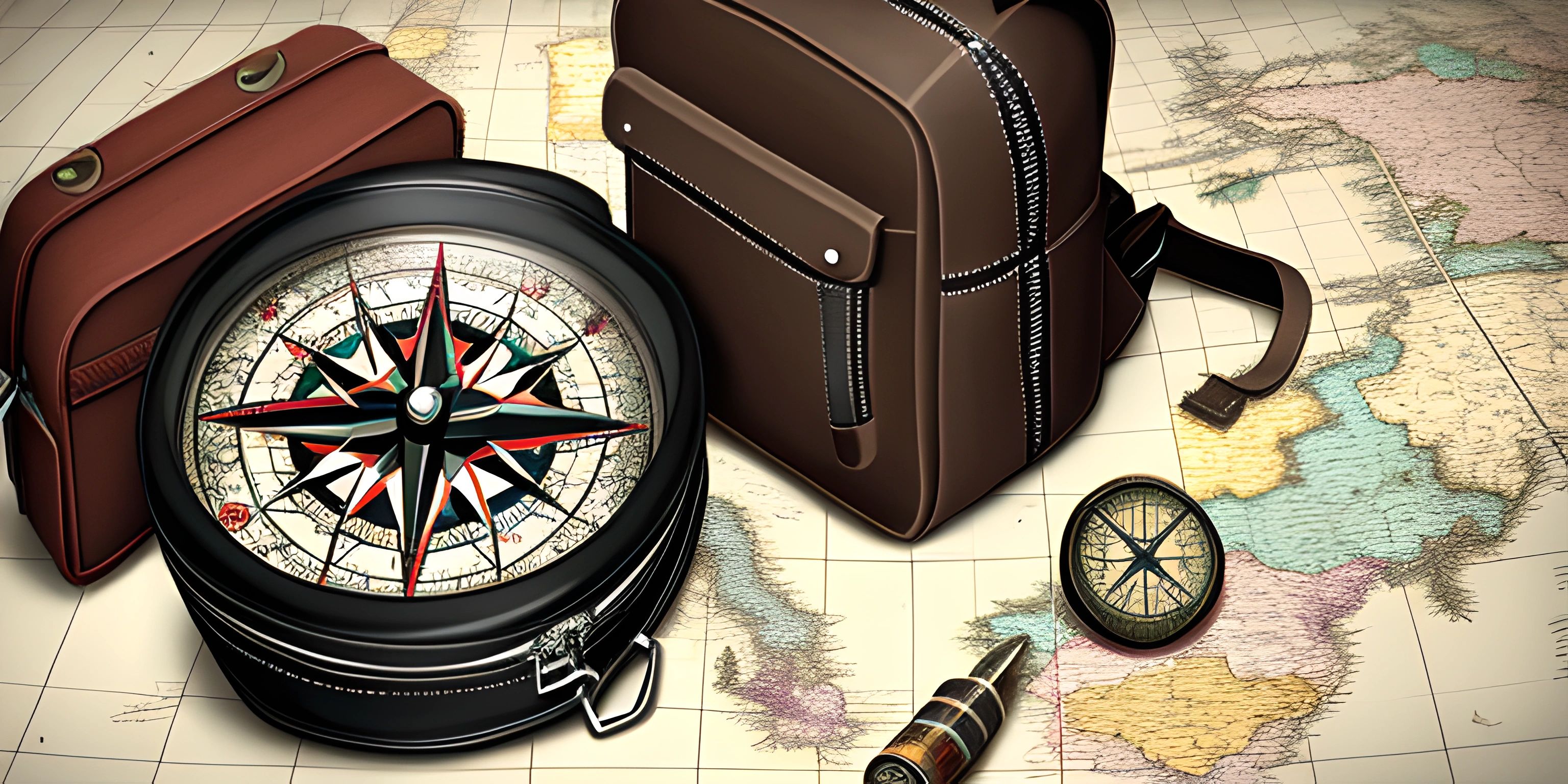
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Once upon a time, a weary traveler needed to pack their knapsack with valuable items, but the knapsack had a weight limit. They wanted to maximize the total value of the items, without exceeding the weight limit. Thus, the knapsack problem was born.
The knapsack problem is a classic optimization problem in computer science. It asks: given a set of items, each with a weight and a value, what is the best combination of items to include in a knapsack with a weight limit? The goal is to maximize the total value of the items, without exceeding the weight limit.
Brute Force Approach
A naive approach to solve the knapsack problem is to generate all possible subsets of items and calculate their total weights and values. Then, we can pick the subset with the highest value that doesn't exceed the weight limit. This method is known as the brute force approach. However, this method is very inefficient, as it has a time complexity of O(2^n), where n is the number of items.
Dynamic Programming
We can do better. Say hello to dynamic programming, a powerful technique that can be used to solve optimization problems by breaking them down into simpler overlapping subproblems. In this case, we can use dynamic programming to solve the knapsack problem more efficiently.
Step-by-Step Solution
Here's a step-by-step guide to solving the knapsack problem using dynamic programming:
- Create a 2D table
dp[i][w]
, wherei
represents the index of items andw
represents the weight capacity of the knapsack. - Fill the table by iterating through all the items and possible weights.
- For each item, compare the value of including it in the knapsack with the value of excluding it.
- Store the maximum value obtained in
dp[i][w]
. - The final solution will be stored in
dp[n][W]
, wheren
is the number of items andW
is the weight limit of the knapsack.
Here's a Python implementation of the dynamic programming solution:
def knapSack(W, wt, val, n): dp = [[0 for w in range(W + 1)] for i in range(n + 1)] for i in range(n + 1): for w in range(W + 1): if i == 0 or w == 0: dp[i][w] = 0 elif wt[i-1] <= w: dp[i][w] = max(val[i-1] + dp[i-1][w-wt[i-1]], dp[i-1][w]) else: dp[i][w] = dp[i-1][w] return dp[n][W] # Example usage val = [60, 100, 120] wt = [10, 20, 30] W = 50 n = len(val) print(knapSack(W, wt, val, n)) # Output: 220
This dynamic programming solution has a time complexity of O(nW), where n
is the number of items and W
is the weight limit of the knapsack. Although it might not seem much better than the brute force approach, the difference in performance becomes significant when dealing with larger input sizes.
Now, our weary traveler can efficiently pack their knapsack with the most valuable items, maximizing their fortune while sticking to the weight limit. And you, dear reader, have learned a powerful technique to solve optimization problems like the knapsack problem. Happy packing!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: What Programming Means (psst, it's free!).