Exploring High-Level Programming Languages
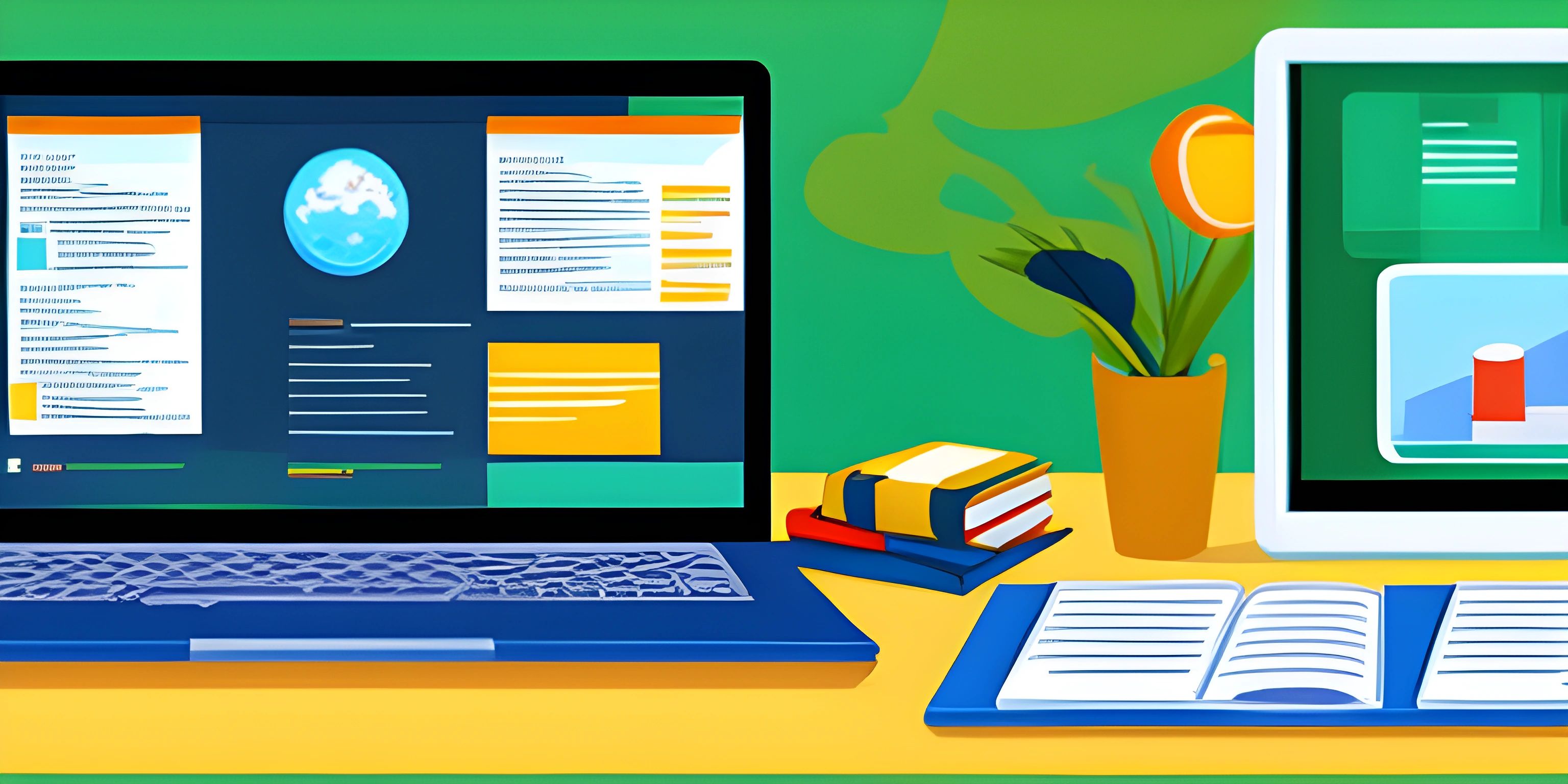
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
If programming languages were animals, high-level languages would be the dolphins of the ocean – friendly, intelligent, and capable of performing impressive tricks. High-level programming languages are designed to be easy for humans to read and write, making them the go-to choice for many developers. But what exactly makes these languages so special?
High-level languages abstract away the nitty-gritty details of machine code, allowing you to focus on solving problems rather than getting bogged down with syntax and hardware-specific quirks. They're like the translators of the coding world, bridging the gap between human logic and computer execution.
Features of High-Level Languages
One of the main attractions of high-level languages is their readability. Code written in high-level languages often resembles human language, making it more intuitive and easier to understand. For instance, compare a simple print statement in Python with its equivalent in assembly language:
Python:
print("Hello, world!")
Assembly:
section .data hello db 'Hello, world!',0 section .text global _start _start: mov eax,4 mov ebx,1 mov ecx,hello mov edx,13 int 0x80 mov eax,1 xor ebx,ebx int 0x80
It's like comparing a friendly chat with a friend to deciphering ancient hieroglyphs!
Abstraction
High-level languages provide a level of abstraction that allows developers to write code without worrying about the underlying hardware. This abstraction makes it easier to write, read, and maintain code. Imagine trying to write a novel where every sentence had to specify the physical actions of the writer's fingers on the keyboard. High-level languages let you focus on the story, not the typing.
Portability
One of the superpowers of high-level languages is their portability. Because they are not tied to a specific type of computer hardware, code written in high-level languages can often run on different types of systems with little to no modification. This portability is akin to a universal translator – write your code once, and it'll be understood across the globe!
Memory Management
Forget about manually allocating and deallocating memory – high-level languages usually handle memory management for you. This feature reduces the chances of memory leaks and other related bugs, allowing developers to concentrate on the logic of their applications. Think of it as having a personal assistant who takes care of all the mundane tasks, so you can focus on the big picture.
Popular High-Level Languages
Let's explore some popular high-level languages and their unique features.
Python
Python is often hailed as the Swiss Army knife of programming languages. Its simplicity and readability make it a favorite among beginners and experts alike. Python's extensive standard library and vibrant community mean you have a wealth of resources at your fingertips for almost any task, from web development to data science.
# Python example: Finding the sum of a list numbers = [1, 2, 3, 4, 5] total = sum(numbers) print("The total is:", total)
JavaScript
JavaScript is the backbone of web development. It allows developers to create interactive and dynamic web pages. With the rise of frameworks like React and Node.js, JavaScript has expanded its reach beyond the browser, making it a versatile and powerful language.
// JavaScript example: Changing the content of a div document.getElementById("myDiv").innerHTML = "Hello, world!";
Java
Java is renowned for its portability thanks to the Java Virtual Machine (JVM). Code written in Java can run on any device that has a JVM, making it a popular choice for building cross-platform applications. Its robustness and performance make it a mainstay in enterprise environments.
// Java example: Printing "Hello, world!" public class HelloWorld { public static void main(String[] args) { System.out.println("Hello, world!"); } }
Advantages of High-Level Languages
Increased Productivity
High-level languages often come with rich libraries and frameworks that speed up development. Instead of reinventing the wheel, you can leverage existing tools to build applications quickly and efficiently. It's like having a well-stocked toolbox – you have the right tool for every job.
Easier Debugging and Maintenance
With their readable syntax and powerful debugging tools, high-level languages make it easier to find and fix bugs. Code maintenance becomes less of a chore and more of a manageable task. Think of it as having a clear roadmap – when you know where you're going, it's easier to stay on course.
Strong Community Support
High-level languages often have large, active communities. This support system means that when you encounter a problem, there's a good chance someone else has faced it before and can help you out. It's like having a team of experts on call, ready to assist whenever you need it.
Rapid Development
The combination of abstraction, rich libraries, and community support means you can bring your ideas to life quickly. Whether you're prototyping a new feature or building a full-fledged application, high-level languages enable rapid development. It's like having a superpower – you can achieve more in less time.
When to Use High-Level Languages
High-level languages are suitable for a wide range of applications, but they're particularly well-suited for:
- Web Development: Languages like JavaScript and Python are staples in web development, enabling the creation of dynamic and interactive web pages.
- Data Science and Machine Learning: Python's extensive libraries, such as NumPy, Pandas, and TensorFlow, make it a go-to language for data analysis and machine learning.
- Automation and Scripting: High-level languages excel at automating repetitive tasks and scripting complex workflows.
- Cross-Platform Development: Languages like Java allow you to build applications that run on multiple platforms without modification.
Conclusion
High-level programming languages have revolutionized the way we write code, making it more accessible, maintainable, and efficient. Their readability, abstraction, and portability have made them indispensable tools in the modern developer's toolbox. Whether you're just starting your coding journey or are a seasoned developer, high-level languages offer a plethora of benefits that can help you achieve your goals faster and with less hassle.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust - A Language You'll Love (psst, it's free!).
FAQ
What is a high-level programming language?
A high-level programming language is a type of programming language that is designed to be easy for humans to read and write. It abstracts away the details of the computer's hardware, allowing developers to focus on solving problems rather than dealing with machine code.
Why are high-level languages more readable?
High-level languages often use syntax that resembles human language and common mathematical notation. This readability makes it easier for developers to understand, write, and maintain code, reducing the likelihood of errors.
What are some popular high-level programming languages?
Some popular high-level programming languages include Python, JavaScript, and Java. Each of these languages has its own unique features and strengths, making them suitable for different types of applications.
How do high-level languages handle memory management?
High-level languages typically include automatic memory management, which means the language runtime automatically allocates and deallocates memory as needed. This reduces the risk of memory leaks and other memory-related bugs.
Can high-level languages be used for system programming?
While high-level languages are primarily designed for application development, some, like Python and Java, can be used for system programming tasks. However, for tasks that require direct hardware manipulation or high performance, low-level languages like C or assembly are often more suitable.