Complex Numbers in Programming
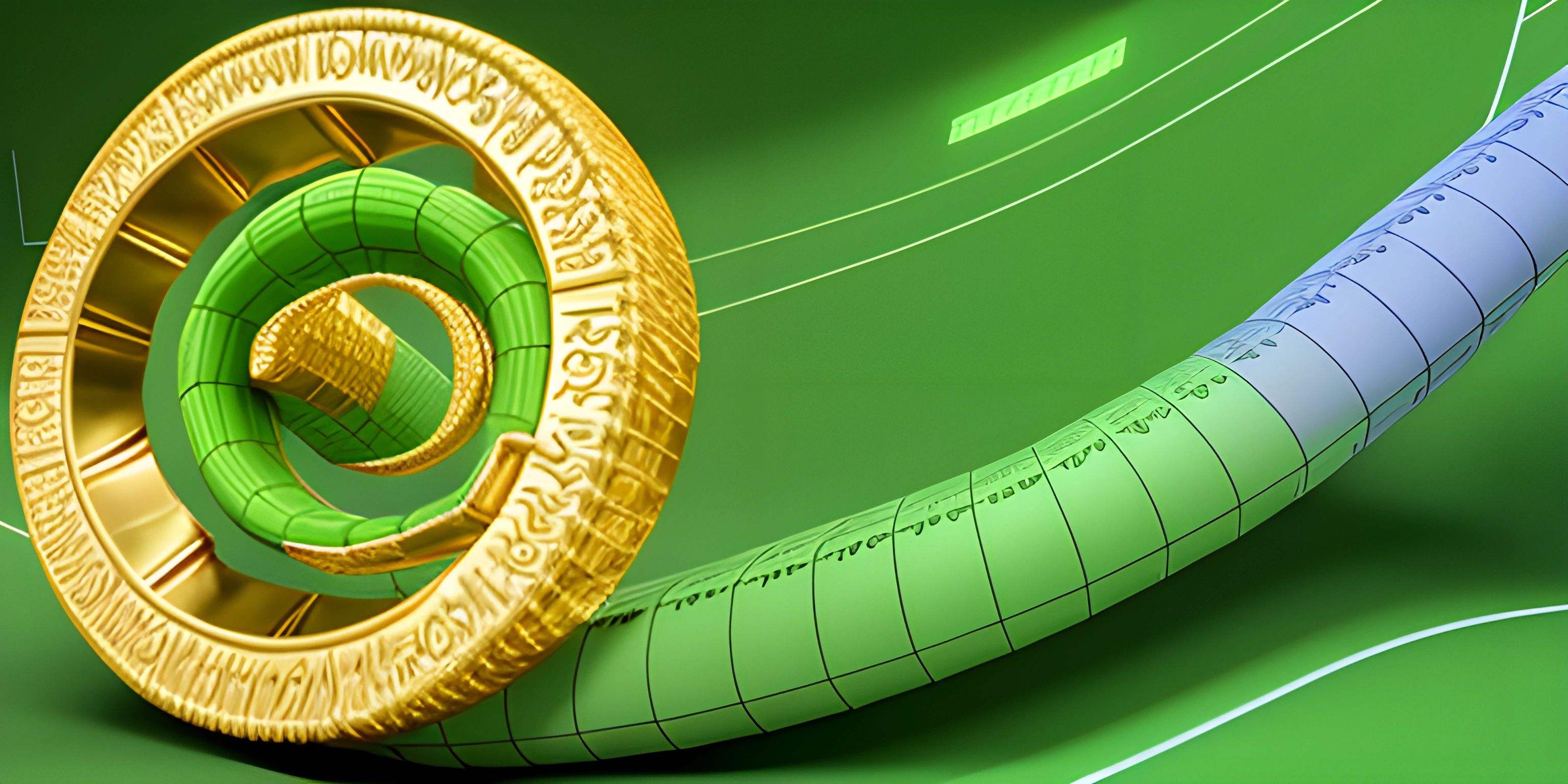
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Complex numbers are like the mysterious creatures of the mathematical world, often misunderstood but full of hidden powers. In programming, they can help us solve problems that would otherwise be impossible (or at least really tricky) to tackle. So, buckle up as we dive into the realm of complex numbers and how they can be used in various programming languages!
What are Complex Numbers?
In mathematics, a complex number is a number that can be expressed in the form a + bi
, where a
and b
are real numbers, and i
is the imaginary unit, which satisfies the equation i² = -1
. In other words, complex numbers extend the realm of real numbers by introducing an imaginary part.
Now that we've got a basic understanding of complex numbers, let's see how we can use them in programming.
Complex Numbers in Python
Python provides built-in support for complex numbers through the complex
data type. To create a complex number, you can use the complex()
function or the j
suffix for the imaginary part:
c1 = complex(3, 4) # 3 + 4j c2 = 3 + 4j # Another way to create a complex number
Python offers a variety of operations and functions for working with complex numbers. Here are some examples:
c1 = 3 + 4j c2 = 2 - 3j # Addition c_sum = c1 + c2 # (5 + 1j) # Multiplication c_product = c1 * c2 # (18 + 1j) # Conjugate c_conjugate = c1.conjugate() # (3 - 4j) # Absolute value (magnitude) c_abs = abs(c1) # 5.0
Complex Numbers in C++
In C++, we can use the complex
library to work with complex numbers. First, we need to include the library and create complex numbers using the complex
class template:
#include <complex> std::complex<double> c1(3, 4); // 3 + 4i std::complex<double> c2(2, -3); // 2 - 3i
C++ provides several functions for performing operations with complex numbers:
#include <complex> #include <iostream> int main() { std::complex<double> c1(3, 4); std::complex<double> c2(2, -3); // Addition std::complex<double> c_sum = c1 + c2; std::cout << "Sum: " << c_sum << std::endl; // (5, 1) // Multiplication std::complex<double> c_product = c1 * c2; std::cout << "Product: " << c_product << std::endl; // (18, 1) // Conjugate std::complex<double> c_conjugate = std::conj(c1); std::cout << "Conjugate: " << c_conjugate << std::endl; // (3, -4) // Absolute value (magnitude) double c_abs = std::abs(c1); std::cout << "Absolute value: " << c_abs << std::endl; // 5.0 return 0; }
Complex Numbers in Other Languages
While Python and C++ provide built-in support for complex numbers, other languages may require external libraries or custom implementations. For instance, in JavaScript, you can use the mathjs
library, and in Java, you can use the Apache Commons Math library.
Conclusion
Complex numbers can be a powerful tool in programming, allowing us to solve problems involving imaginary numbers and perform calculations that would be difficult or impossible using real numbers alone. By understanding how to work with complex numbers in various programming languages, you can unlock new possibilities and enhance your problem-solving skills. So go ahead, embrace the magic of complex numbers, and let your imagination run wild!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Mandelbrot Set (psst, it's free!).
FAQ
What are complex numbers in programming?
Complex numbers are numbers that consist of a real part and an imaginary part. In programming, complex numbers are used to perform mathematical operations that involve imaginary numbers, such as addition, subtraction, multiplication, and division. They are commonly used in scientific, engineering, and graphics applications, where complex mathematical calculations are required.
How do I create a complex number in Python?
In Python, you can create a complex number using the complex()
function, or by using the j
notation for the imaginary part. Here's an example of creating a complex number in Python:
complex_number = 3 + 4j alternatively, complex_number = complex(3, 4)
How do I perform operations with complex numbers in programming languages like C++?
In C++, you can use the <complex>
library to handle complex numbers. This library provides a complex class template that can be used to perform operations on complex numbers. Here's an example of creating and adding two complex numbers in C++:
#include <iostream> #include <complex> int main() { std::complex<double> num1(3, 4); std::complex<double> num2(5, -2); std::complex<double> result = num1 + num2; std::cout << "The sum of the complex numbers is: " << result << std::endl; return 0; }
Can I use complex numbers in JavaScript?
JavaScript does not have native support for complex numbers, but you can use external libraries like complex.js to handle complex numbers in JavaScript. Here's an example of creating and adding two complex numbers using the complex.js library:
const Complex = require("complex.js"); const num1 = new Complex(3, 4); const num2 = new Complex(5, -2); const result = num1.add(num2); console.log("The sum of the complex numbers is: ", result.toString());
Is there a standard library for complex numbers in programming languages?
Many programming languages have built-in support or standard libraries for handling complex numbers. For instance, Python has the built-in complex
type, C++ has the <complex>
library, and Java has the Complex
class in the org.apache.commons.math3.complex
package. However, not all languages have built-in support; in such cases, you can use external libraries or implement your own complex number classes or functions.