C++ Overview
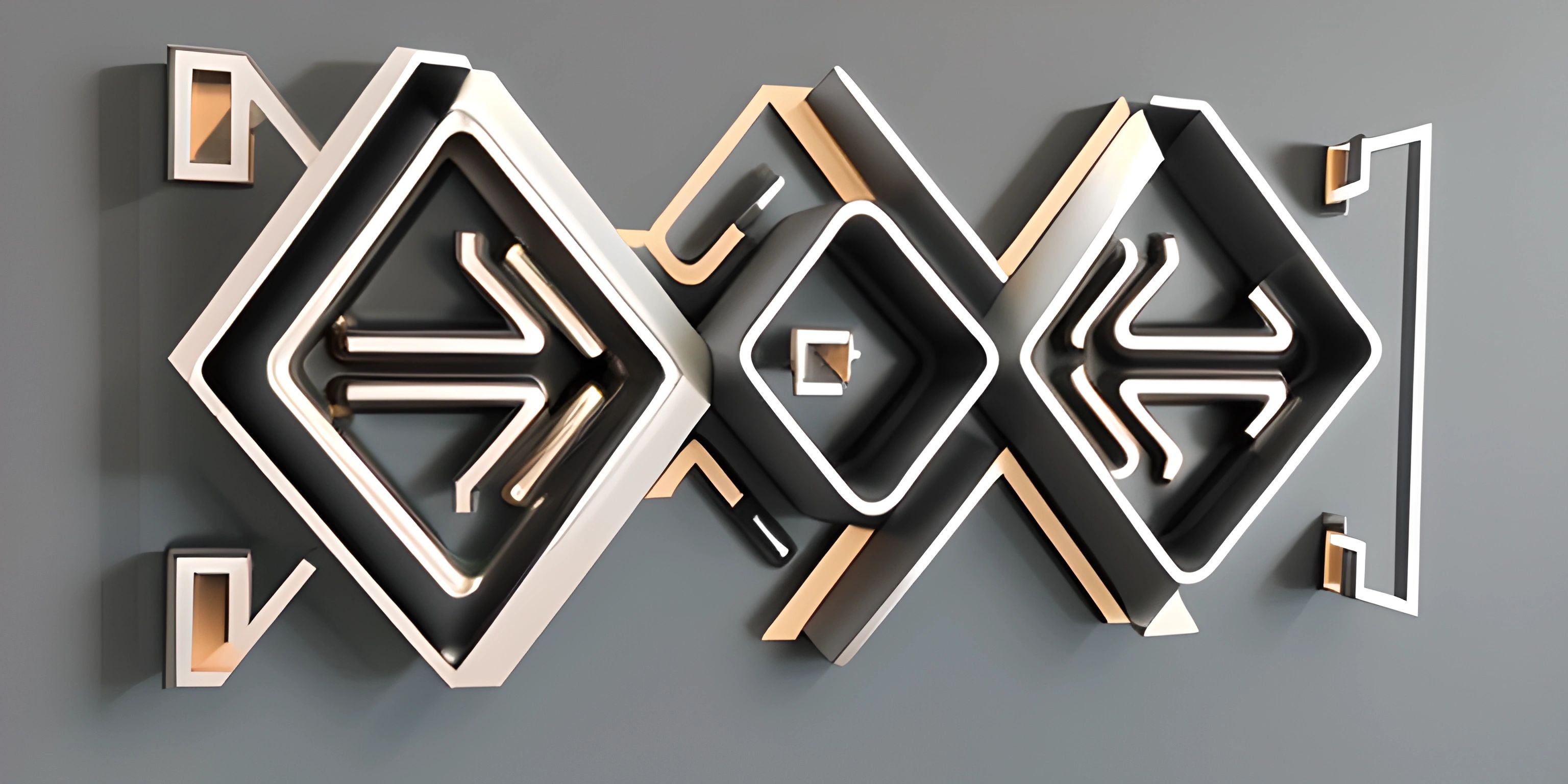
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
C++, pronounced "C plus plus," is a general-purpose programming language that has been around since the 1980s. Created by Bjarne Stroustrup as an extension of the C programming language, it has become a crucial player in the world of modern programming. In this article, we'll explore the significance of C++ and why many developers continue to favor it.
Origins and Evolution of C++
C++ came into existence when Bjarne Stroustrup, in an effort to improve the C language, added object-oriented features, giving birth to a powerful new language called "C with Classes." Later, it was renamed C++ when more features, such as operator overloading, were introduced.
Over the years, C++ has undergone numerous revisions and updates, notably C++98, C++03, C++11, C++14, C++17, and most recently, C++20. Each revision has brought new features and enhancements, making the language more efficient, expressive, and powerful.
Why C++ Matters
C++ is considered one of the most important programming languages for several reasons:
-
Performance: C++ provides low-level access to memory and efficient management of resources, making it suitable for performance-critical applications, such as video games, operating systems, and embedded systems.
-
Object-Oriented Features: The object-oriented capabilities of C++ enable better organization and reusability of code, which enhances modularity and maintainability.
-
Compatibility: C++ maintains a high level of compatibility with its predecessor, C, allowing developers to integrate existing C codebases seamlessly.
-
Wide Adoption: C++ is widely adopted across various industries, and many popular frameworks and libraries have been built using it, such as Qt, Boost, and Unreal Engine.
-
Community: C++ boasts a large community of developers, which translates into extensive support, resources, and tools available to learn and master the language.
Getting Started with C++
To dive into C++, you'll need a compiler and an Integrated Development Environment (IDE). Popular C++ compilers include GCC (GNU Compiler Collection), Clang, and Microsoft Visual C++. Common IDEs for C++ development are Visual Studio, Code::Blocks, and CLion.
Here's a short example of a basic C++ program that prints "Hello, C++!" to the console:
#include <iostream> int main() { std::cout << "Hello, C++!" << std::endl; return 0; }
The #include <iostream>
directive allows us to use input and output (I/O) functions, like std::cout
. The main
function is the entry point of the program, and std::cout
is used to output text to the console.
Exploring C++ Concepts
C++ offers a wide range of features and concepts that make it a versatile and powerful language. Here are some key topics to explore when learning C++:
- Variables and Data Types
- Control Structures
- Functions
- Classes and Objects
- Inheritance and Polymorphism
- Templates
- Error Handling
As you learn more about C++ and explore its many features, you'll discover the reasons behind its enduring popularity and its continued significance in modern programming.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust - A Language You'll Love (psst, it's free!).
FAQ
What is C++ and why is it significant in modern programming?
C++ is a general-purpose, high-level programming language that is an extension of the C language. It was created by Bjarne Stroustrup in 1985 and has since become one of the most widely used languages in the world. C++ is known for its efficiency, strong performance, and flexibility, making it ideal for system programming, game development, and other performance-critical applications. Its significance in modern programming can be attributed to its versatile features, object-oriented programming support, and extensive standard library.
Can you provide a simple example of a C++ program?
Of course! Here's a basic C++ program that prints "Hello, World!" to the console:
#include <iostream> int main() { std::cout << "Hello, World!" << std::endl; return 0; }
This program includes the <iostream>
header file, which provides functionalities for input and output streams. The main()
function serves as the entry point for the program, and the std::cout
object is used to output "Hello, World!" to the console.
What are the main differences between C and C++?
While C++ is an extension of the C language, there are several notable differences between the two:
- Object-oriented programming: C++ supports object-oriented programming (OOP), which allows for better organization, code reusability, and easier maintenance. C is a procedural language and doesn't support OOP.
- Standard Template Library (STL): C++ includes the STL, a powerful library with a variety of containers, algorithms, and iterators that simplify complex programming tasks. C lacks this feature.
- Exception handling: C++ provides built-in support for handling exceptions, making it easier to manage errors during program execution. C doesn't have built-in exception handling and relies on error codes and external libraries.
- Constructors and destructors: C++ supports constructors and destructors for objects, which are special member functions that are automatically called when an object is created or destroyed. C doesn't have this feature.
What are some popular applications of C++?
C++ is widely used in various domains due to its high performance and versatility. Some popular applications include:
- System programming: Operating systems, drivers, and embedded systems often use C++ for its low-level control and efficiency.
- Game development: C++ is popular among game developers for its performance and ability to handle complex, real-time graphics rendering.
- Desktop applications: Many desktop applications, such as Microsoft Office and Adobe Photoshop, are built using C++.
- Web browsers: Major web browsers like Google Chrome and Mozilla Firefox utilize C++ for their core functionalities.
- Machine learning and artificial intelligence: C++ can be used to develop efficient machine learning and AI algorithms, especially in cases where high performance is crucial.
How can I start learning C++?
To get started with C++, follow these steps:
- Install a C++ compiler: Download and install a compiler like GCC, Clang, or Visual Studio, which allows you to compile and run C++ code on your machine.
- Set up an Integrated Development Environment (IDE): Choose an IDE like Visual Studio Code, CLion, or Code::Blocks to write, compile, and debug your C++ code.
- Learn C++ basics: Start with the basics, such as syntax, data types, loops, and functions, and gradually progress to more advanced concepts like object-oriented programming and templates.
- Practice coding: Write and run sample programs to practice various C++ concepts and build your confidence in the language.
- Explore resources: Utilize online tutorials, books, and courses to enhance your understanding of C++ and stay up-to-date with new developments in the language.