Go Introduction
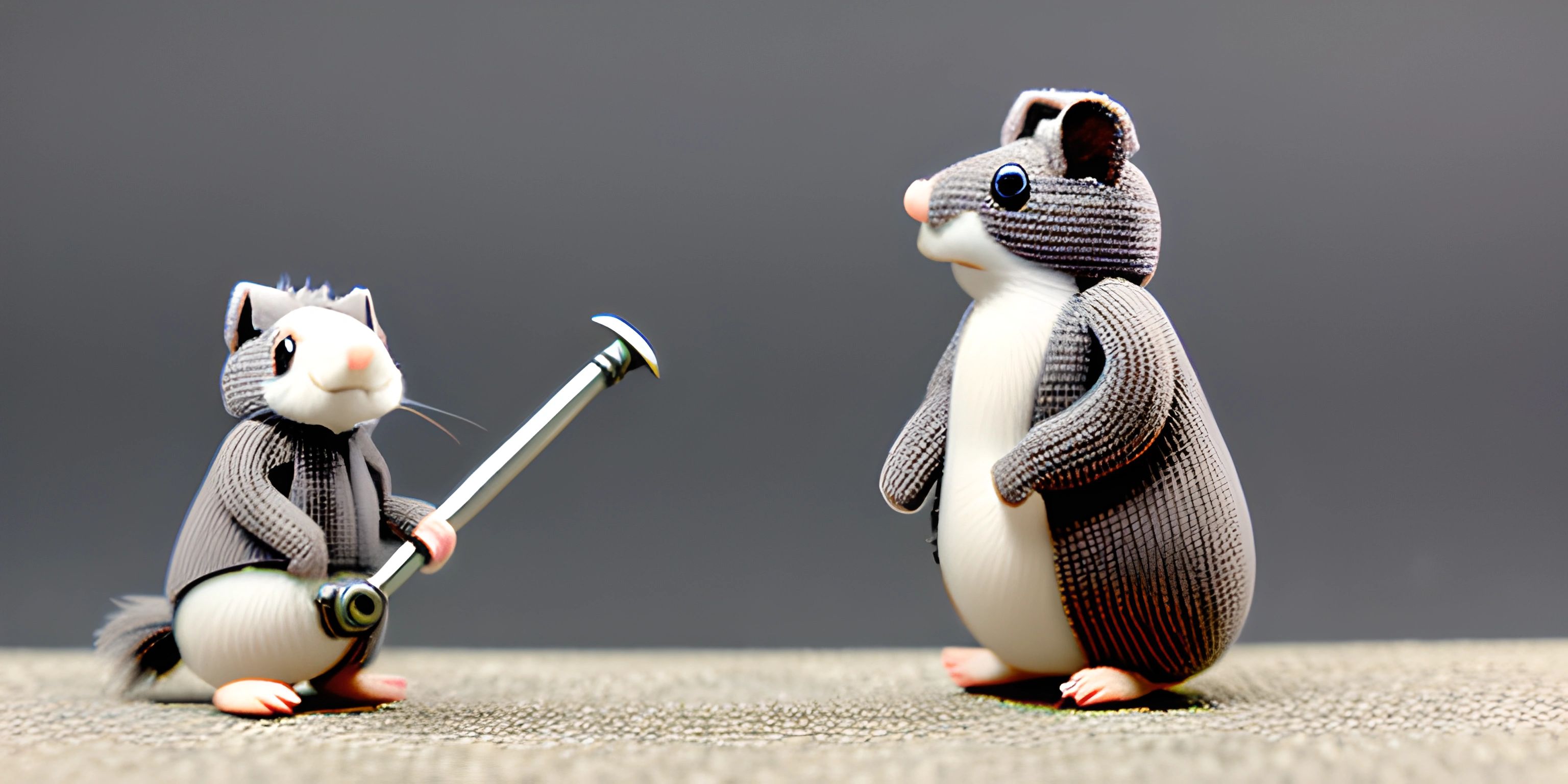
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Go, also known as Golang, is an open-source programming language developed by Google. Designed by Robert Griesemer, Rob Pike, and Ken Thompson, Go was created to address some of the shortcomings of other languages while maintaining their strengths. With a focus on simplicity, efficiency, and strong support for concurrent programming, Go has quickly gained popularity among developers.
Key Features of Go
1. Simplicity
Go was designed with simplicity in mind, making it easy to learn and use. Its syntax is clean, minimalistic, and reminiscent of the C programming language. This allows developers to write clear and concise code, making it easier to maintain and understand.
2. Static Typing
Go is a statically typed language, which means that the data types of variables are determined at compile time. This helps catch errors early and ensures type safety, reducing the chances of runtime errors.
3. Garbage Collection
Go has built-in garbage collection, which automatically manages memory allocation and deallocation. This frees developers from having to manually manage memory, reducing the likelihood of memory leaks and making code simpler and more efficient.
4. Concurrency
One of Go's most powerful features is its support for concurrent programming. Go introduces the concept of goroutines, which are lightweight threads managed by the Go runtime. Goroutines make it easy to write concurrent code, allowing developers to write more efficient and responsive applications.
5. Standard Library
Go comes with a rich standard library that provides a wide range of pre-built functions and packages, covering common tasks such as file I/O, networking, and cryptography. This powerful library helps developers save time and effort by providing reusable code components.
6. Cross-platform Compatibility
Go is cross-platform, which means that you can write your code once and run it on multiple operating systems, including Windows, macOS, Linux, and more. This feature makes Go a great choice for developing applications that need to run on various platforms.
Getting Started with Go
To start using Go, you'll first need to install the Go compiler and tools. Once that's done, you can start experimenting with writing Go code.
A simple "Hello, World!" program in Go would look like this:
package main import "fmt" func main() { fmt.Println("Hello, World!") }
Save this code in a file named hello.go
and run it using the go run
command:
$ go run hello.go
This will compile and run your Go program, displaying "Hello, World!" on the command line.
As you continue to learn Go, you'll discover its many features and benefits, making it a powerful and versatile language for a wide range of applications. So go forth and explore the world of Go!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust - A Language You'll Love (psst, it's free!).
FAQ
What is the Go programming language?
Go, also known as Golang, is an open-source programming language developed by Google. It was created with simplicity, efficiency, and concurrent programming in mind. Go offers strong support for concurrent programming, garbage collection, and offers ease of use with its static typing and clear syntax.
What are the key features of Go?
Some of the key features of Go include:
- Static typing: Go uses static typing, which means the type of a variable is known at compile time, making it easier to catch errors early.
- Simplicity: Go has a clean and minimal syntax, making it easier to learn and read.
- Concurrency: Go has built-in support for concurrent programming using goroutines and channels.
- Garbage collection: Go includes an efficient garbage collector that helps manage memory allocation and deallocation.
- Standard library: Go comes with a robust standard library, providing a wide range of built-in packages for various functionalities.
- Cross-platform: Go compiles to native code for various platforms, including Windows, macOS, and Linux.
Why should I consider using Go for my projects?
You should consider using Go because of its simplicity, performance, and strong support for concurrent programming. Go is known for its excellent performance, similar to languages like C and C++, while providing the ease of use of languages like Python and JavaScript. Its built-in concurrency support makes it an ideal choice for developing scalable web servers, data pipelines, and other applications that require efficient parallelism.
How do I get started with Go?
To get started with Go, first download and install the Go programming language from the official website: https://golang.org/dl/. Once installed, you can start writing Go programs using any text editor or integrated development environment (IDE) of your choice. Popular IDEs for Go development include Visual Studio Code, GoLand, and LiteIDE. Additionally, you can find numerous tutorials, documentation, and resources on the official Go website and the Go community online.
Can you provide a simple example of a Go program?
Absolutely! Here's a basic "Hello, World!" program in Go:
package main import "fmt" func main() { fmt.Println("Hello, World!") }
This program defines a main
function, which is the entry point of the application. Inside the function, we use the fmt
package to print "Hello, World!" to the console.