C++ Basics
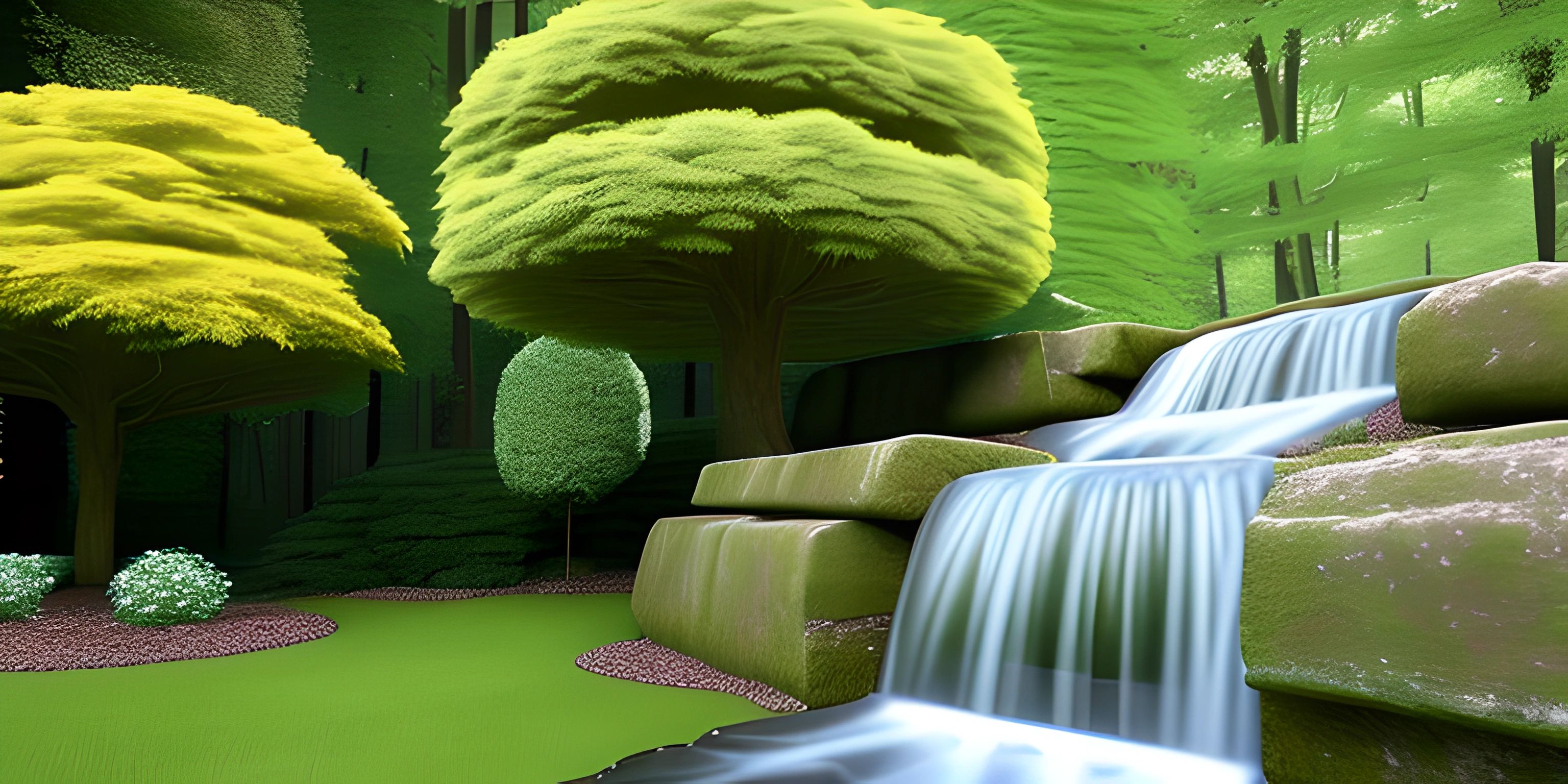
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
C++ is a powerful and versatile programming language, widely used in various domains such as game development, high-performance computing, and embedded systems. As an extension of the C programming language, C++ adds features like classes and objects, making it a popular choice for both beginners and experienced programmers. So buckle up and let's dive into the world of C++!
History
C++ was created by Bjarne Stroustrup in 1985. The language was originally called "C with Classes" as it was designed to add object-oriented programming capabilities to the C language. As the language evolved, it was renamed to C++ to signify the incremental improvements it brought to the table.
Syntax
Similar to C, the syntax of C++ is simple and consistent. Here's a basic "Hello, World!" example to give you a taste:
#include <iostream> int main() { std::cout << "Hello, World!" << std::endl; return 0; }
Let's break down the elements of this simple program:
#include <iostream>
: This line is a preprocessor directive that tells the compiler to include theiostream
header file, which is necessary for input and output operations in C++.int main()
: This line declares themain()
function, which is the entry point of every C++ program. Theint
beforemain()
indicates that the function returns an integer value.{}
: Curly braces are used to define the scope of functions, classes, and other constructs in C++.std::cout << "Hello, World!" << std::endl;
: This line is responsible for printing "Hello, World!" to the console.std::cout
stands for "console output" andstd::endl
is used to insert a newline character.return 0;
: This line indicates the successful termination of themain()
function and returns the value0
to the operating system.
Variables and Data Types
C++ has a strong type system, meaning each variable must have a specific data type. Some common data types in C++ are int
(integer), float
(floating-point number), double
(double-precision floating-point number), and char
(character). To declare a variable, you must specify its type followed by its name:
int age = 25; float average = 80.5; char letter = 'A';
Control Structures
C++ provides various control structures to manage the flow of your program, such as if
, else
, while
, and for
. Here's a simple example demonstrating the use of an if
statement:
int age = 18; if (age >= 18) { std::cout << "You are eligible to vote!" << std::endl; } else { std::cout << "You are not eligible to vote yet." << std::endl; }
Functions
Functions are reusable blocks of code that perform a specific task. They can accept input (parameters) and return output (return value). Here's an example of a simple function that calculates the sum of two numbers:
int add(int a, int b) { int sum = a + b; return sum; } int main() { int result = add(5, 10); std::cout << "The sum is: " << result << std::endl; return 0; }
Classes and Objects
C++ is an object-oriented programming language, and it supports classes and objects. A class is a blueprint for creating objects, and it defines properties (also known as attributes) and behaviors (methods) that the objects can have. Here's a simple example of a class called Person
:
class Person { public: std::string name; int age; void sayHello() { std::cout << "Hello, my name is " << name << " and I am " << age << " years old." << std::endl; } }; int main() { Person person1; person1.name = "Alice"; person1.age = 30; person1.sayHello(); return 0; }
With this introduction, you're now ready to explore the world of C++ programming. There's much more to learn, so don't hesitate to dive deeper and expand your skills! Happy coding!
FAQ
What is C++ and what is its purpose?
C++ is a general-purpose programming language that was created by Bjarne Stroustrup in 1985 as an extension of the C programming language. Its main purpose is to enable system programming and resource-constrained applications due to its low-level memory manipulation capabilities. It is widely used in game development, embedded systems, and high-performance applications.
How is C++ different from C?
While C++ is an extension of C, it introduces several new features and enhancements, including:
- Object-oriented programming (OOP) with classes and objects
- Stronger type checking
- Better support for code reuse through inheritance and polymorphism
- Support for exception handling
- Support for templates, allowing generic programming
- The Standard Template Library (STL), which provides a rich set of reusable data structures and algorithms
How do I write a simple "Hello, World!" program in C++?
To write a "Hello, World!" program in C++, you would use the following code:
#include <iostream> int main() { std::cout << "Hello, World!" << std::endl; return 0; }
This program includes the <iostream>
header file which provides the std::cout
object and the std::endl
manipulator for output. The main
function is the entry point of the program, and std::cout << "Hello, World!" << std::endl;
prints the message "Hello, World!" followed by a newline character. The program then returns 0, indicating successful execution.
What is the importance of the main() function in C++?
The main()
function serves as the entry point of a C++ program. When a program is executed, the operating system calls the main()
function, and the program starts executing from there. The main()
function must have a specific signature, typically int main()
or int main(int argc, char* argv[])
. The first version takes no arguments, while the second version takes command-line arguments. The main()
function should return an integer, usually 0 for successful execution or a non-zero value for an error.
What are some common data types in C++?
C++ supports several basic data types, including:
int
: Integer valuesfloat
: Single-precision floating-point valuesdouble
: Double-precision floating-point valueschar
: Single characters or small integersbool
: Boolean values (true or false) C++ also allows you to create custom data types using structures, classes, and unions.