C# Introduction
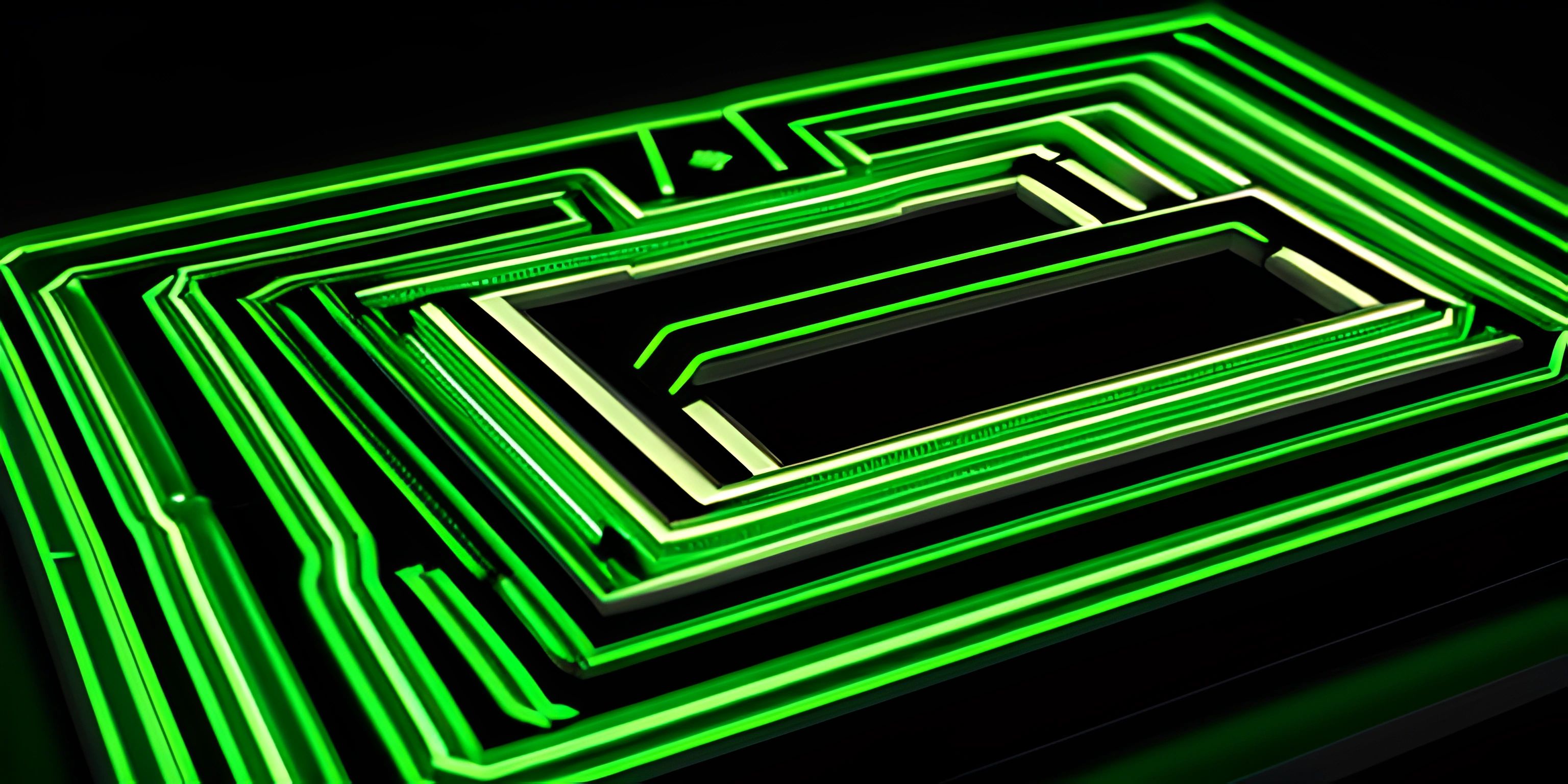
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
C# (pronounced "C Sharp") is a versatile and powerful programming language, used to build a wide range of applications, from desktop apps to web services and even video games. Built on the .NET framework, C# offers a comprehensive suite of features that make it a popular choice for developers around the world.
History and Purpose
C# was developed by Microsoft in 2000 as part of their .NET initiative. The language was designed by Anders Hejlsberg, who also created Turbo Pascal and was a key contributor to the development of Delphi. C# was intended to be a modern, object-oriented language that would be easy to learn and use, while still being powerful and efficient.
One of the main purposes of C# was to provide a simpler, safer, and more consistent programming experience in comparison with languages like C++ and Java. C# borrows some of its syntax and concepts from these languages, but also introduces new features that make it distinct and unique.
Features
C# comes with a variety of features that make it a compelling choice for developers. Some of its key features include:
Object-Oriented
C# is inherently object-oriented, allowing you to create modular, reusable code by defining classes and objects. This design makes it easier to manage complex applications and promotes code reusability.
Type Safety
C# is a strongly typed language, which means that the data types of variables must be explicitly defined. This helps prevent errors and promotes code clarity, as developers can quickly understand the purpose of a variable by looking at its data type.
Garbage Collection
C# features automatic garbage collection, which helps manage memory by automatically releasing objects that are no longer in use. This reduces the chances of memory leaks and makes it easier for developers to manage memory efficiently.
Modern Syntax
C# is known for its clean, modern syntax that is easy to read and understand. This makes it more accessible to new developers and allows experienced programmers to quickly become proficient in the language.
Cross-Platform
With the introduction of .NET Core, C# has become a cross-platform language, allowing you to develop applications that can run on Windows, macOS, and Linux. This greatly expands the potential audience for your applications and makes it easier to target multiple platforms with a single codebase.
Getting Started with C#
Now that you have a basic understanding of what C# is and what it offers, you're ready to start learning the language. Be sure to check out the following resources to help you on your journey:
- C# Variables and Data Types
- C# Control Structures
- C# Classes and Objects
With these resources and some practice, you'll be well on your way to becoming a skilled C# developer!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust - A Language You'll Love (psst, it's free!).
FAQ
What is C# and why is it popular?
C# (pronounced "C Sharp") is a versatile, object-oriented programming language developed by Microsoft. It is popular because of its simplicity, flexibility, and powerful features. C# is widely used for developing various types of applications, including desktop, web, mobile, and video game applications. Its seamless integration with the .NET Framework and modern language features make it a top choice for many developers.
What are some key features of the C# programming language?
Some of the key features of C# include:
- Object-oriented programming (OOP) with support for inheritance, encapsulation, and polymorphism.
- Strong typing and automatic memory management.
- Built-in support for exception handling.
- Integration with the .NET Framework, which provides a vast library of classes and functions.
- Modern language features like LINQ, async/await, and pattern matching.
- Cross-platform compatibility with the .NET Core framework.
How do I get started with C# programming?
To start programming in C#, you'll need an Integrated Development Environment (IDE). The most popular IDE for C# development is Microsoft Visual Studio, which is available in multiple editions, including a free Community edition. After installing Visual Studio, you can create a new C# project, write your code, and compile and run your application within the IDE.
What does a basic C# program look like?
A basic C# program consists of a class with a Main
method, which serves as the entry point of the application. Here's a simple example:
using System; class Program { static void Main(string[] args) { Console.WriteLine("Hello, World!"); } }
This program defines a Program
class with a static Main
method. Inside the method, it uses the Console.WriteLine()
function to print "Hello, World!" to the console.
Can I use C# to develop cross-platform applications?
Yes, you can use C# to develop cross-platform applications using the .NET Core framework. .NET Core is an open-source, cross-platform version of the .NET Framework that allows you to build and run C# applications on multiple platforms, including Windows, macOS, and Linux. To get started, you'll need to install the .NET Core SDK and use a compatible IDE or text editor, such as Visual Studio, Visual Studio Code, or JetBrains Rider.