Rust Syntax
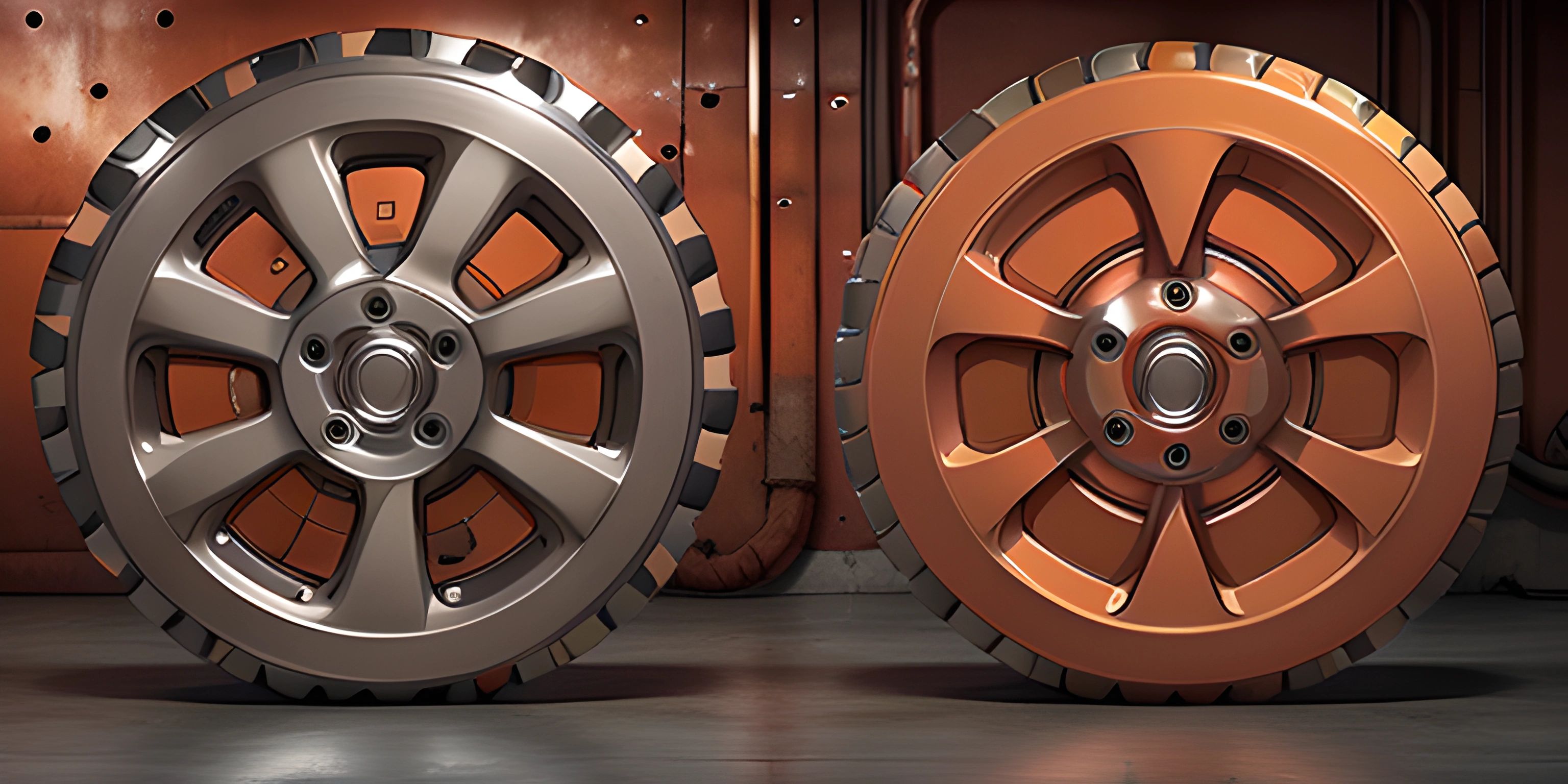
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
In the world of programming languages, Rust has made a name for itself with its focus on safety, performance, and concurrency. To get comfortable with Rust, it's important to familiarize yourself with its syntax and key language features. So, buckle up and let's dive into the world of Rust!
Basic Syntax
A Rust program starts with the main
function, which is the entry point of your application. Here's a simple "Hello, world!" example:
fn main() { println!("Hello, world!"); }
Variables and Constants
In Rust, you can declare variables using the let
keyword, and constants using the const
keyword. Variables are immutable by default, but you can make them mutable by adding the mut
keyword:
fn main() { let x = 42; // immutable variable let mut y = 7; // mutable variable const Z: i32 = 5; // constant with explicit type }
Control Flow
Rust has the usual control flow structures you'd expect, such as if
, else
, while
, and for
loops:
fn main() { let number = 5; if number % 2 == 0 { println!("Even"); } else { println!("Odd"); } let mut counter = 0; while counter < 10 { counter += 1; } for i in 1..11 { println!("Number: {}", i); } }
Functions
Functions in Rust are defined with the fn
keyword, followed by the function name, parameters, and return type (if applicable). Functions can also be nested inside other functions:
fn main() { let result = multiply(2, 3); println!("The product is {}", result); } fn multiply(x: i32, y: i32) -> i32 { x * y }
Key Language Features
Ownership and Borrowing
Rust's unique ownership system allows it to manage memory safely without a garbage collector. Ownership rules enforce that for any given value, there is exactly one owner, and when the owner goes out of scope, the value is dropped. Rust also supports borrowing, which allows values to be temporarily borrowed by references without changing ownership:
fn main() { let s1 = String::from("hello"); let len = calculate_length(&s1); println!("The length of '{}' is {}.", s1, len); } fn calculate_length(s: &String) -> usize { s.len() }
Enums and Pattern Matching
Rust has strong support for enumerations, or enums, which allow you to define a type that has a fixed set of possible values. Enums can be used with Rust's powerful pattern matching to handle different cases concisely:
enum Color { Red, Green, Blue, } fn main() { let color = Color::Red; match color { Color::Red => println!("It's red!"), Color::Green => println!("It's green!"), Color::Blue => println!("It's blue!"), } }
Rust is an exciting language with unique features that help you write safe, performant, and concurrent code. With a basic understanding of its syntax and features, you're now ready to dive deeper into the Rust ecosystem and start building amazing applications!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust - A Language You'll Love (psst, it's free!).
FAQ
What is the basic syntax structure of a Rust program?
The basic structure of a Rust program consists of functions, variables, and expressions. The main
function is the entry point of the program. Here's an example of a simple Rust program:
fn main() { let greeting = "Hello, world!"; println!("{}", greeting); }
How do I define a variable in Rust?
In Rust, you can define a variable using the let
keyword followed by the variable name, an equal sign (=), and the value. By default, variables are immutable, meaning their values cannot be changed. To make a variable mutable, use let mut
. Here's an example:
fn main() { let name = "Alice"; let mut age = 30; println!("{} is {} years old.", name, age); age = 31; println!("{} is now {} years old.", name, age); }
What are some common control structures in Rust?
Rust has several control structures, including if
expressions, loop
, while
, and for
. Here are some examples:
// if expression if age >= 18 { println!("You are an adult."); } else { println!("You are a minor."); } // loop loop { println!("This will print until you stop the program."); } // while while counter < 10 { println!("Counter: {}", counter); counter += 1; } // for for number in 1..6 { println!("Number: {}", number); }
How do I create a function in Rust?
You can create a function in Rust using the fn
keyword followed by the function name, a pair of parentheses ()
containing any input parameters, and a block containing the function body. Here's an example:
fn greet(name: &str) { println!("Hello, {}!", name); } fn main() { greet("Alice"); }
How do I return a value from a Rust function?
To return a value from a Rust function, add an arrow ->
followed by the return type after the input parameters. In the function body, use the return
keyword followed by the value to return early, or simply write the value without a semicolon at the end for an implicit return. Here's an example:
fn add(a: i32, b: i32) -> i32 { a + b } fn main() { let sum = add(5, 7); println!("The sum is: {}", sum); }