Introduction to C++
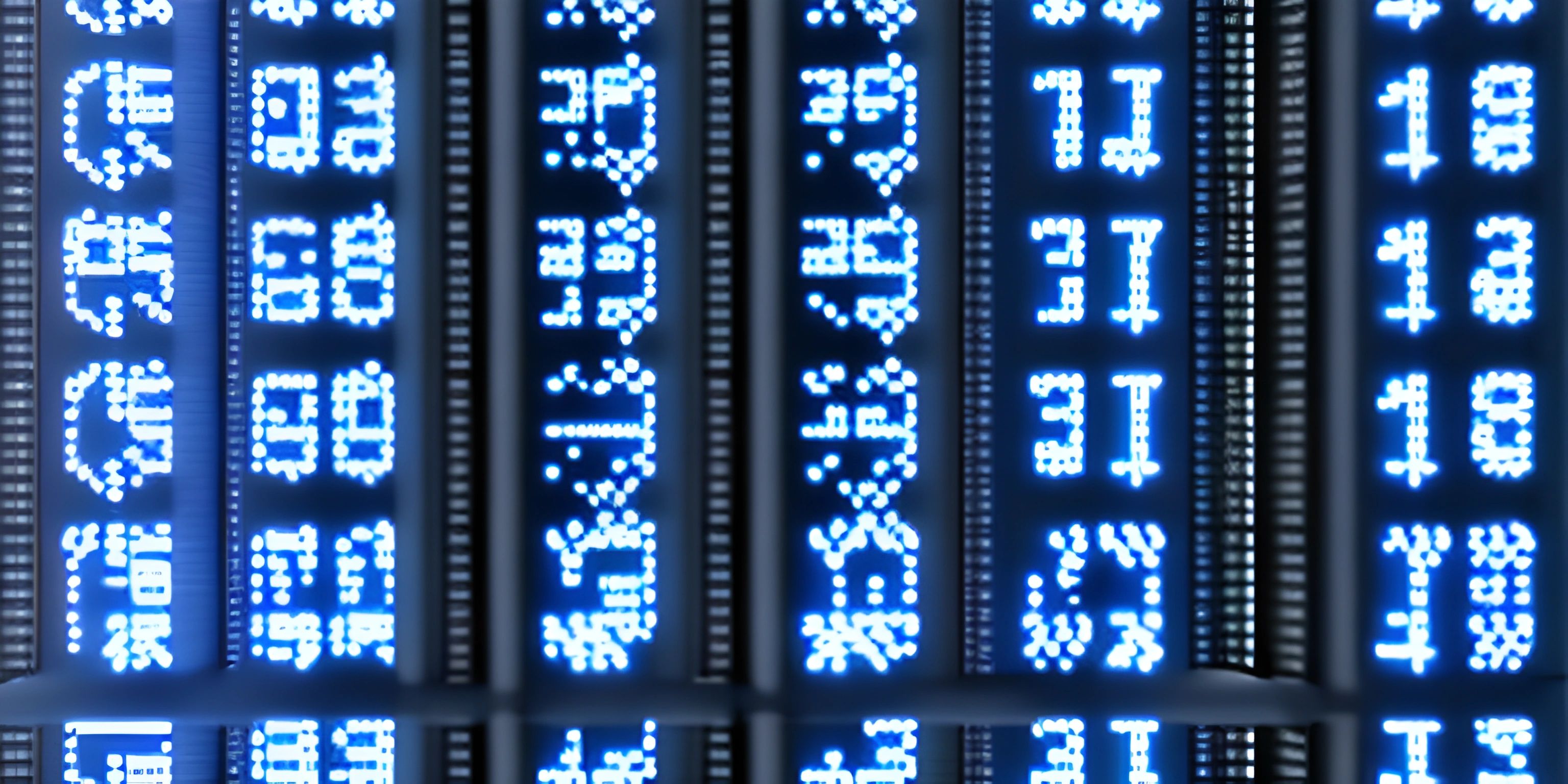
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
C++ has been a staple in the programming world since its inception in the 1980s. As a powerful, high-performance language, it has found a home in a variety of applications, from game engines to embedded systems. In this article, we'll introduce you to the world of C++ and explore some of its key features that make it stand out among other programming languages.
Origins and Purpose
C++ was designed by Bjarne Stroustrup as an extension of the C programming language. The idea behind C++ was to provide a language that offers both high-level abstractions and low-level control over system resources. This unique blend of features makes C++ an excellent choice for performance-critical applications, such as video games and financial trading systems.
Object-Oriented Programming
One of the most significant additions that C++ brought to the table is its support for object-oriented programming (OOP). OOP allows developers to model real-world entities using classes and objects, which can help make code more organized and easier to understand.
In C++, you can create a class to define a custom data type, complete with its own member variables and member functions. For example, here's a simple C++ class that represents a point in two-dimensional space:
class Point { public: double x; double y; void print_coordinates() { std::cout << "(" << x << ", " << y << ")\n"; } };
This class has two member variables, x
and y
, which store the coordinates of a point. It also has a member function, print_coordinates
, that prints the coordinates to the console.
Standard Template Library (STL)
Another notable feature of C++ is the Standard Template Library (STL). The STL is a collection of template classes and functions that provide common programming data structures and algorithms, such as vectors, lists, and queues. It's designed to be efficient and easy to use, making it a valuable asset for C++ developers.
For example, if you need a dynamic array in C++, you can use the std::vector
class from the STL:
#include <vector> #include <iostream> int main() { std::vector<int> numbers = {1, 2, 3, 4, 5}; for (int num : numbers) { std::cout << num << '\n'; } return 0; }
This code demonstrates how to create a vector, initialize it with a list of numbers, and iterate over its elements using a range-based for
loop.
Performance and Control
C++ provides a high level of control over system resources, making it an attractive option for performance-critical applications. With features like pointers and manual memory management, developers can fine-tune their code to optimize for specific hardware and use cases.
However, this power comes with a trade-off: it's easier to make mistakes that can lead to issues like memory leaks or crashes. As a C++ developer, you'll need to balance the benefits of low-level control with the risks of writing error-prone code.
Conclusion
C++ is a versatile and powerful programming language with a rich feature set, including object-oriented programming, the Standard Template Library, and fine-grained control over system resources. If you're interested in learning more about C++, you can explore the many resources available online, including tutorials, documentation, and community forums. Good luck, and happy coding!