Object-Oriented Programming
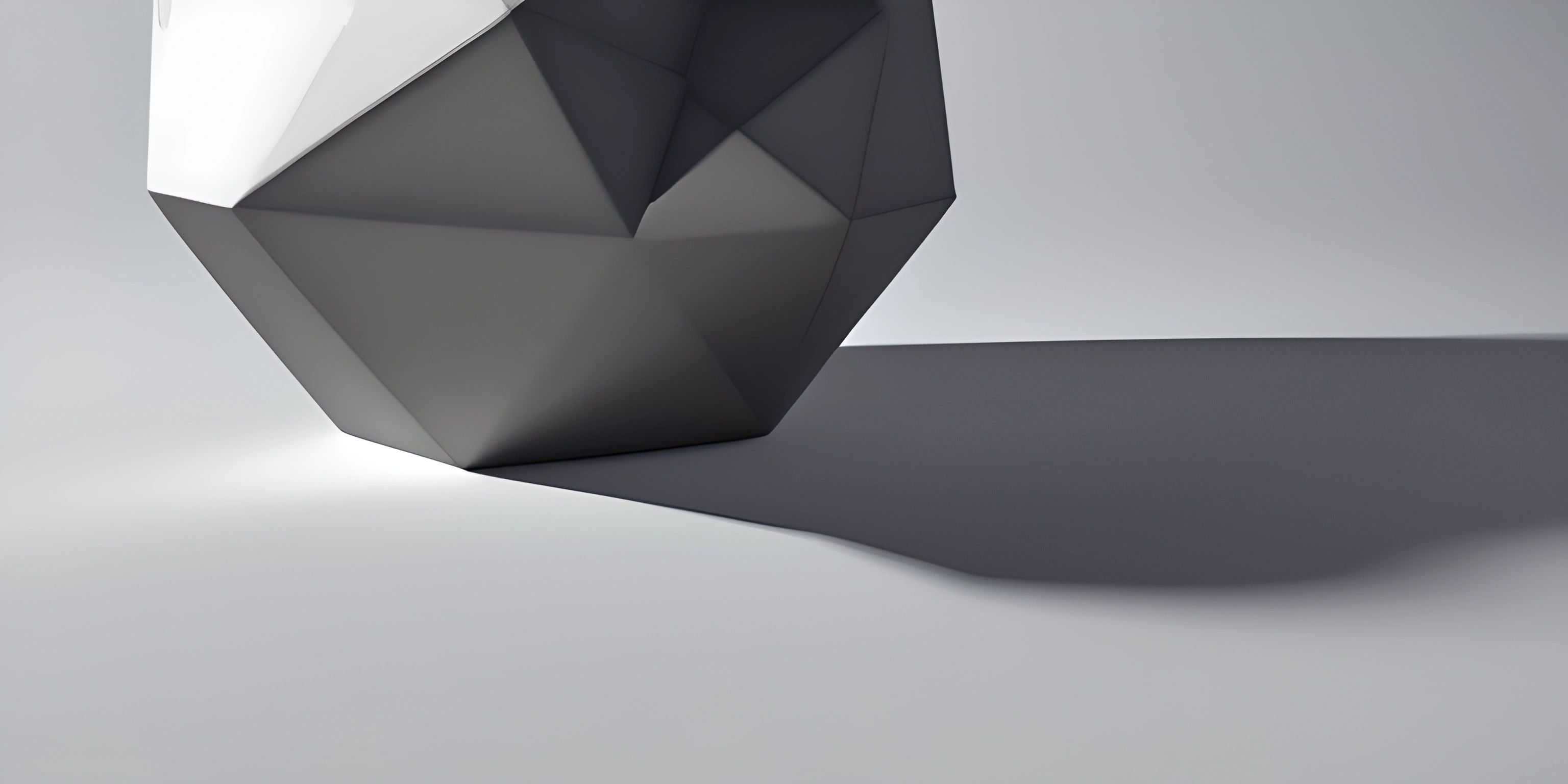
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Imagine you're a chef, and you have to make a complex meal with lots of different ingredients. You could throw everything into a huge pot and hope for the best, but that's likely to result in a mess. Instead, it's better to organize and compartmentalize each part of the meal. This is similar to the concept behind Object-Oriented Programming (OOP).
OOP is a programming paradigm that organizes code into objects, which contain both data and the methods that operate on that data. This approach provides a clear structure and promotes code reusability and maintainability.
Classes and Objects
In OOP, a class is a blueprint for creating objects. You can think of a class as a cookie cutter, and objects as the cookies made using that cutter. Each object created from a class is called an instance of that class.
Here's an example using Python:
class Dog: def __init__(self, name, age): self.name = name self.age = age def bark(self): print(f"{self.name} barks!") my_dog = Dog("Buddy", 3) my_dog.bark() # Output: Buddy barks!
In this example, we define a Dog
class with a constructor method (__init__
) and a bark
method. We then create an instance of the Dog
class called my_dog
and call the bark
method on it.
Inheritance
Inheritance is the process by which one class inherits the properties and methods of another class. This allows you to create a new class based on an existing one, without having to rewrite the code.
For example, let's say we want to create a GoldenRetriever
class that inherits from our Dog
class:
class GoldenRetriever(Dog): def fetch(self, item): print(f"{self.name} fetched the {item}!") my_golden = GoldenRetriever("Charlie", 2) my_golden.bark() # Output: Charlie barks! my_golden.fetch("stick") # Output: Charlie fetched the stick!
The GoldenRetriever
class inherits the bark
method from the Dog
class and adds a new fetch
method.
Encapsulation
Encapsulation is the act of bundling data and methods that operate on that data within a single unit (the object). This helps to hide the internal workings of an object from the outside world and promotes modularity.
For example, let's say we want to prevent direct access to the age
attribute of our Dog
class:
class Dog: # ... (previous code omitted for brevity) def get_age(self): return self.__age def set_age(self, age): if age > 0: self.__age = age else: print("Age must be greater than 0.") my_dog = Dog("Buddy", 3) print(my_dog.get_age()) # Output: 3 my_dog.set_age(-1) # Output: Age must be greater than 0.
We use the get_age
and set_age
methods to access and modify the age
attribute, rather than directly accessing it.
Polymorphism
Polymorphism allows methods to take on different forms depending on the context in which they're called. This means that a single method name can be used for different implementations within different classes.
For example, let's create a Cat
class and see how polymorphism works:
class Cat: def __init__(self, name, age): self.name = name self.age = age def speak(self): print(f"{self.name} meows!") class Dog: # ... (previous code omitted for brevity) def speak(self): print(f"{self.name} barks!") my_cat = Cat("Whiskers", 4) my_dog = Dog("Buddy", 3) for pet in [my_cat, my_dog]: pet.speak() # Output: # Whiskers meows! # Buddy barks!
Both Cat
and Dog
classes have a speak
method, but they behave differently. Polymorphism allows us to treat them as the same type of object and call their respective speak
methods without needing to know the exact class they belong to.
Embracing object-oriented programming concepts like classes, inheritance, encapsulation, and polymorphism helps developers write cleaner, more organized, and maintainable code. So, the next time you're cooking up a program, remember to keep things organized and compartmentalized – just like a chef preparing a delicious meal.
FAQ
What is object-oriented programming (OOP)?
Object-oriented programming, or OOP, is a programming paradigm that focuses on organizing code around objects, which represent real-world entities or abstract concepts. These objects have properties (attributes) and can perform actions (methods). By using OOP, code is more modular, maintainable, and reusable, making it easier to develop complex software systems.
What are the main principles of OOP?
The four main principles of OOP are:
- Encapsulation: Bundling data (attributes) and methods (functions) together in a single unit (object), which helps to keep the code organized and secure.
- Inheritance: Creating new classes (child classes) that inherit the properties and methods of existing classes (parent classes), promoting code reusability and modularity.
- Polymorphism: Allowing different objects to be treated as instances of the same class, enabling the use of a single interface for various data types and simplifying code.
- Abstraction: Simplifying complex systems by breaking them into smaller, more manageable parts (objects), focusing on essential features and hiding irrelevant details.
How do classes and objects relate in OOP?
In object-oriented programming, a class is a blueprint or template that defines the properties and methods for a particular type of object. An object is an instance of a class, created according to the class's blueprint. Each object created from the same class will have the same properties and methods, but may have different values for those properties.
How does inheritance work in OOP?
Inheritance is a key principle in OOP that allows one class (the child class) to inherit attributes and methods from another class (the parent class). The child class can then add or override properties and methods as needed. This promotes code reusability and modularity by allowing developers to build on existing classes without rewriting code.
Can you provide a simple example of OOP in Python?
Certainly! Here's a basic example of OOP in Python, where we define a class Dog
and create an object of that class:
class Dog: def __init__(self, name, age): self.name = name self.age = age def bark(self): print("Woof, woof!") # Create a Dog object my_dog = Dog("Buddy", 3) # Access properties and methods print(my_dog.name) # Output: Buddy print(my_dog.age) # Output: 3 my_dog.bark() # Output: Woof, woof!
In this example, we define a Dog
class with an __init__
method (constructor) and a bark
method. We then create a Dog
object named my_dog
and access its properties (name
and age
) and methods (bark
).