A Guide to Dart Programming Language
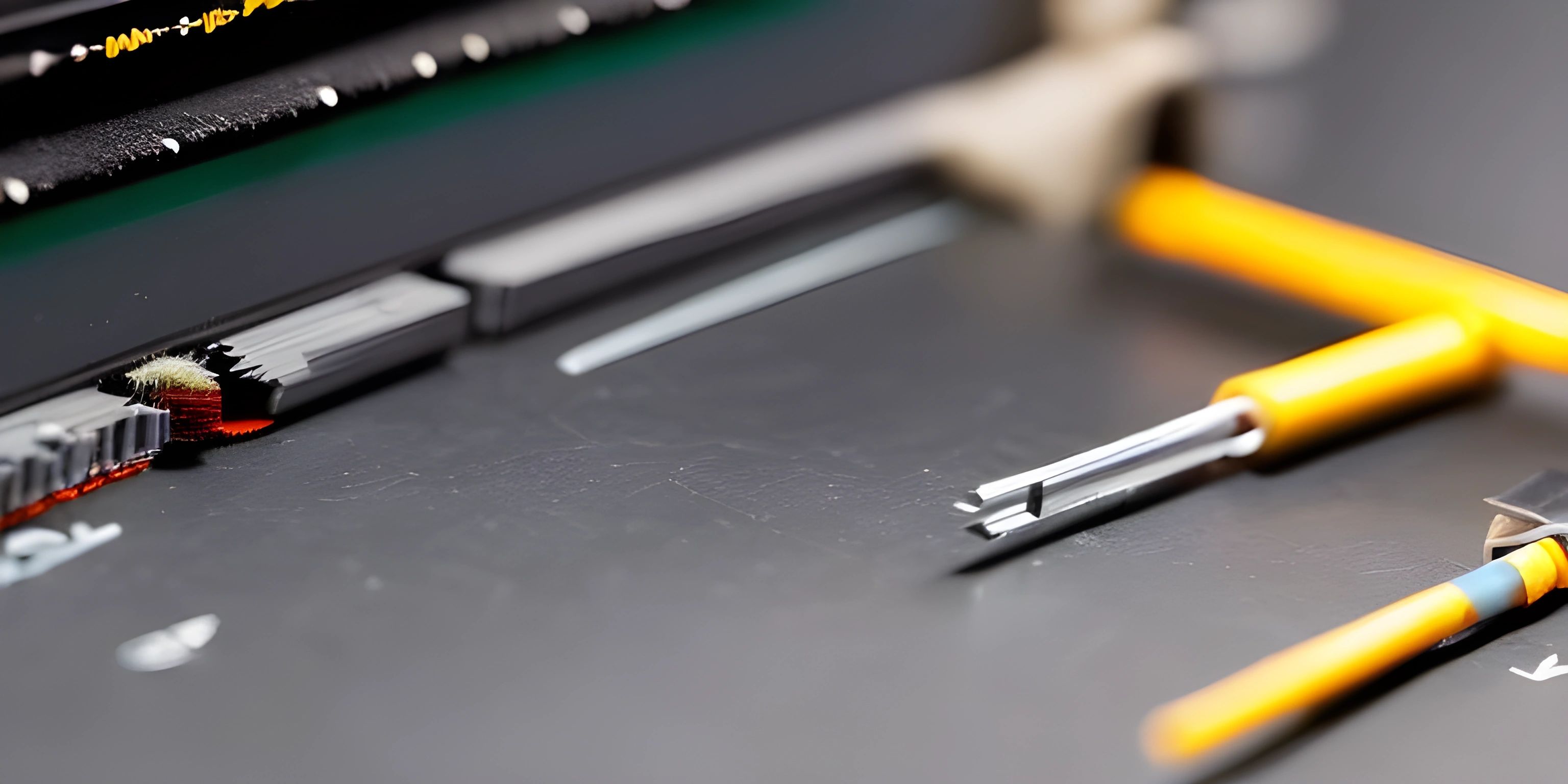
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Dart might not be the first name you think of when you consider programming languages, but it's making a big splash in the pond of front-end development. Why? Because it's the backbone of Flutter, Google's UI toolkit. So let's dive right in!
The Basics
Dart is an object-oriented language, which means it's all about creating and manipulating objects. Don't worry, we're not talking about real-world objects like your coffee mug or your pet iguana, but rather, digital constructs that hold data and behaviors.
Here's an example of how you might define a simple object in Dart:
class Coffee { String brand; double price; Coffee(this.brand, this.price); void drink() { print("You're drinking $brand coffee. Delicious!"); } }
In this example, Coffee
is our object, brand
and price
are properties, and drink()
is a method. When we call drink()
, it'll print out a message saying we're drinking our choice of brand. Tasty!
Dart for Flutter
Dart's real claim to fame is its relationship with Flutter. Flutter is a UI toolkit from Google that allows developers to create natively compiled applications for mobile, web, and desktop from a single codebase. Now that's what we call versatility!
Here's an example of a simple Flutter app written in Dart:
import 'package:flutter/material.dart'; void main() { runApp(MyApp()); } class MyApp extends StatelessWidget { Widget build(BuildContext context) { return MaterialApp( home: Scaffold( appBar: AppBar( title: Text('Hello, Flutter!'), ), body: Center( child: Text('Welcome to the world of Flutter!'), ), ), ); } }
In this snippet, we're creating a simple app with an app bar and a message in the center of the screen. If you've worked with JavaScript's React or Angular, the structure might feel familiar. The difference is, this code can run on iOS, Android, and the web. Talk about killing three birds with one stone!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Why Program? (psst, it's free!).
FAQ
What is Dart used for?
Dart is mainly used for front-end development. It's the primary language used in Flutter, Google's UI toolkit, which allows developers to build natively compiled applications for mobile, web, and desktop from a single codebase.
What's object-oriented programming and how does it relate to Dart?
Object-oriented programming is a programming paradigm based on the concept of "objects", which can contain data and code: data in the form of fields, and code, in the form of procedures. Dart is an object-oriented language, which means you'll be working with objects a lot when programming in Dart.
Is Dart difficult to learn?
Dart is designed to be simple and easy to understand, but like any language, it takes time and patience to master. If you have experience with languages like JavaScript or Java, you'll find many familiar concepts in Dart. The good news is - Dart has excellent documentation, so you'll have plenty of resources to help you along your learning journey.