Docker Multi-Stage Builds
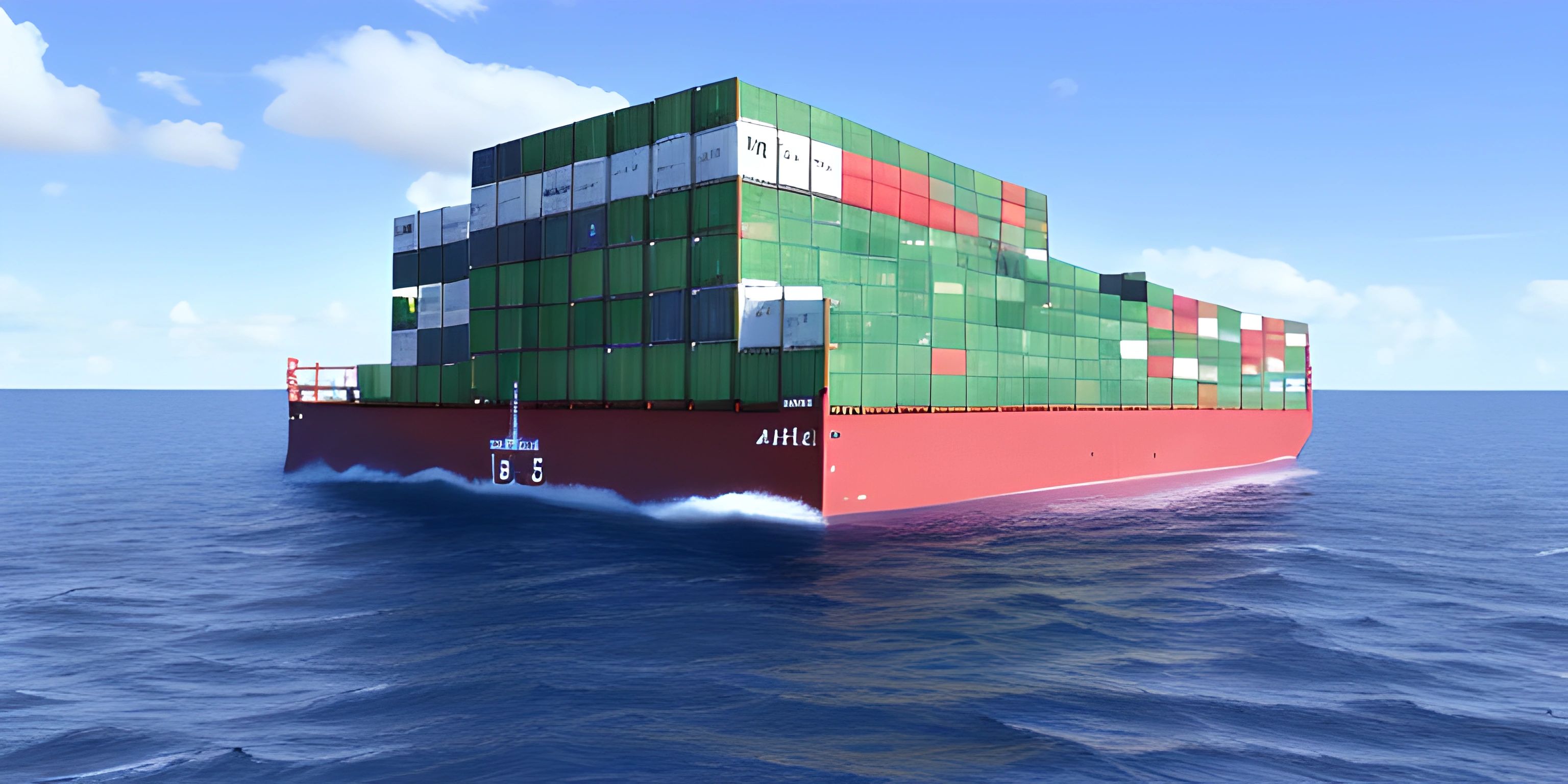
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Docker has become an essential tool in modern software development, as it allows us to build and manage containers and their runtime environments. With Docker, we can create consistent, portable, and reproducible environments that run anywhere Docker is installed. However, to take full advantage of Docker, we must also ensure that our images are lean, fast, and secure. Enter Docker multi-stage builds!
What are Multi-Stage Builds?
Multi-stage builds are a feature of Docker that allows us to build images in multiple stages within a single Dockerfile. Each stage can have its own base image, dependencies, and build artifacts. Stages can be used to perform different tasks, such as building an application, running tests, or creating a production-ready image.
The primary advantage of using multi-stage builds is reducing the final image size by only including the necessary files, dependencies, and tools. This results in smaller images that are faster to build, push, and pull.
How to Use Multi-Stage Builds
A multi-stage build starts by defining a base image for the first stage with the FROM
directive, followed by an AS
directive to give the stage a name:
FROM node:14 AS build
This example creates a stage named build
using the Node.js 14 base image. You can then execute commands within this stage, such as copying files or installing dependencies:
WORKDIR /app COPY . . RUN npm install RUN npm run build
Now, let's create a second stage for the production-ready image. We'll use a different base image (e.g., Alpine) for a smaller final image size:
FROM node:14-alpine AS production
In this stage, we can copy the build artifacts from the previous stage using the COPY
directive with the --from
flag:
WORKDIR /app COPY /app/dist /app/dist
Finally, we can define the command to start the application:
CMD ["npm", "start"]
When you build this Dockerfile, Docker will execute each stage sequentially, using the output of the previous stage as input for the next one:
docker build -t my-app:latest .
The final image will only contain the files from the production
stage, resulting in a smaller and optimized image.
Benefits of Multi-Stage Builds
- Smaller image sizes: By selectively copying files and dependencies between stages, you can reduce the final image size significantly.
- Better separation of concerns: Each stage can have a specific goal, such as building the application, running tests, or creating the production image. This makes the Dockerfile more maintainable and easier to understand.
- Improved security: By not including build tools or unused dependencies in the final image, you minimize the attack surface of your container.
Conclusion
Docker multi-stage builds are an excellent way to create optimized, efficient, and secure images. By breaking the build process into multiple stages, you can reduce image size, improve performance, and better organize your Dockerfile. Start incorporating multi-stage builds into your Docker projects, and reap the benefits of leaner and faster containers!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Mandelbrot Set (psst, it's free!).
FAQ
What is a Docker multi-stage build?
Docker multi-stage build is a technique that allows you to efficiently build and optimize Docker images. It works by breaking down the image building process into multiple stages, each with its own set of instructions and dependencies. By doing so, the final image only includes the necessary components, resulting in a smaller image size and improved performance.
How do I use multi-stage builds in Dockerfile?
To use multi-stage builds in a Dockerfile, you need to define multiple stages using the FROM
instruction with the AS
keyword. Each stage can have its own set of instructions and copy artifacts from previous stages using the COPY --from
instruction. Here's an example:
# First stage: build the application FROM node:14 AS build WORKDIR /app COPY package*.json ./ RUN npm install COPY . . RUN npm run build # Second stage: create the final image FROM node:14-alpine WORKDIR /app COPY /app/dist /app/dist COPY package*.json ./ RUN npm install --production CMD ["npm", "start"]
How can multi-stage builds reduce Docker image size?
Multi-stage builds help reduce Docker image size by allowing you to selectively copy only the required artifacts from previous stages into the final image. This way, you can avoid including unnecessary files, build tools, and intermediate artifacts that would otherwise increase the final image size. As a result, you get a lean and optimized image that takes less storage space and loads faster.
Are there any best practices for using Docker multi-stage builds?
Yes, here are some best practices for using Docker multi-stage builds:
- Use a base image with minimal footprint: Choose a lightweight base image such as Alpine Linux to reduce the size of the final image.
- Separate build and runtime dependencies: Install only runtime dependencies in the final stage to avoid including unnecessary build tools and libraries.
- Leverage caching: Organize your Dockerfile instructions to take advantage of Docker's layer caching for faster builds.
- Use explicit stage names: Assign meaningful names to each stage using the
AS
keyword, such asAS build
orAS test
, for better readability and maintainability. - Clean up intermediate artifacts: Remove any temporary files or artifacts that are not needed in the final image after each build stage.