Dockerfile Best Practices
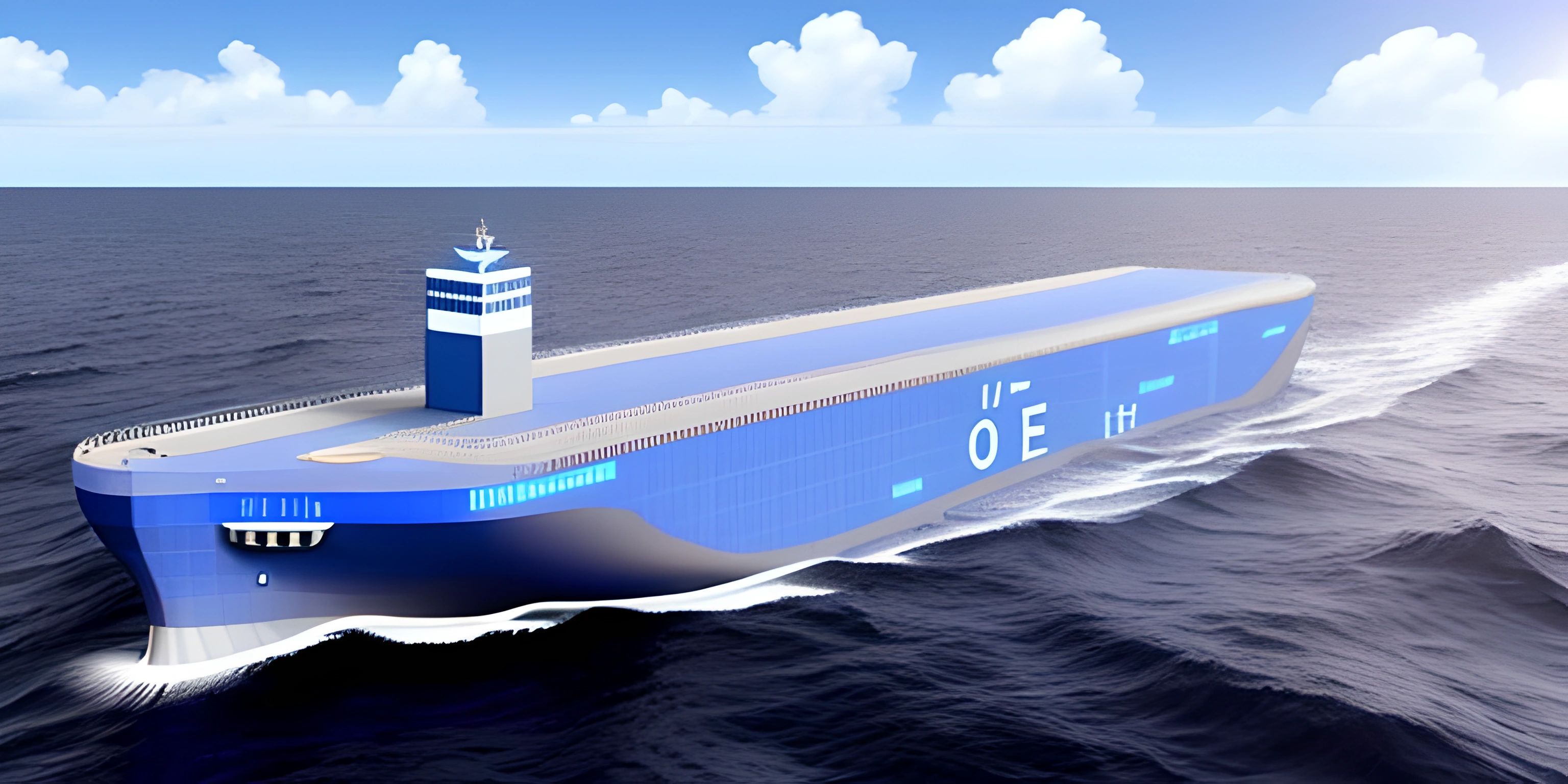
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Docker has become an essential tool in the world of software development and deployment. To harness its full potential, it's crucial to understand and follow best practices when creating Dockerfiles. In this article, we'll explore some tips and tricks to ensure your Dockerfiles are efficient and readable.
Use a Small Base Image
Starting with a small base image can significantly reduce your Docker image size, which in turn will improve download and deploy times. Some popular options for small base images include Alpine Linux and Distroless. Remember to choose a base image that aligns with your application's requirements.
# Good: Using a small base image FROM alpine:3.14 # Bad: Using a large base image FROM ubuntu:20.04
Multi-Stage Builds
To further optimize your Docker images, consider using multi-stage builds. This technique allows you to use multiple FROM
statements and copy artifacts between build stages, reducing the final image size.
# Multi-stage build example FROM node:14 AS build WORKDIR /app COPY . . RUN npm install RUN npm run build FROM nginx:alpine COPY /app/dist /usr/share/nginx/html
Organize and Optimize Layers
Docker images are built in layers, and each instruction in your Dockerfile creates a new layer. It's essential to optimize these layers to minimize the number of cache invalidations and to make your image as small as possible.
# Good: Install dependencies and copy source code in separate layers COPY package.json package-lock.json ./ RUN npm install COPY . . # Bad: Copy source code and install dependencies in a single layer COPY . . RUN npm install
Use .dockerignore
A .dockerignore file helps you exclude unnecessary files and directories from the build context, reducing the overall build time and image size. It's similar to a .gitignore
file.
# Example .dockerignore file node_modules .git Dockerfile *.log
Be Explicit
When writing your Dockerfile, be explicit about the versions of tools and packages you're using. This ensures that your builds are reproducible and prevents unexpected behavior due to updates.
# Good: Specify exact versions FROM node:14.17.6-alpine3.14 RUN npm install -g [email protected] # Bad: Use latest (unpredictable) versions FROM node:latest RUN npm install -g npm
Document Your Dockerfile
Good documentation is crucial for any code, and your Dockerfile is no exception. Use comments to explain the purpose of each instruction and how it contributes to the final image.
# Use a small base image FROM alpine:3.14 # Install required packages RUN apk add --no-cache curl # Set the working directory WORKDIR /app
Following these best practices will help you create efficient and readable Dockerfiles, improving your development and deployment processes. Remember that your specific use case may require additional optimizations, so always consider your application's unique requirements when crafting your Dockerfile.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Common Programming Pitfalls (psst, it's free!).
FAQ
What are some best practices for writing efficient and readable Dockerfiles?
Some best practices for writing efficient and readable Dockerfiles include:
- Use a small base image: Start with a minimal base image that provides only the essentials, such as Alpine Linux.
- Use multi-stage builds: Divide your Dockerfile into multiple stages to minimize the final image size and improve build times.
- Combine commands: Group related commands together using
&&
to reduce the number of layers created. - Use
.dockerignore
: Create a.dockerignore
file to exclude unnecessary files and directories from being copied to the image. - Keep sensitive information out of the Dockerfile: Use environment variables or secret management tools to handle sensitive data.
How can I use multi-stage builds to optimize my Dockerfile?
Multi-stage builds allow you to use multiple FROM
statements in your Dockerfile, creating separate build stages. Each stage can have its own base image and instructions. You can copy files from previous stages to the current one, allowing you to optimize the final image size. Here's an example using a multi-stage build:
# First stage: build the application FROM node:14 AS build WORKDIR /app COPY package*.json ./ RUN npm install COPY . . RUN npm run build # Second stage: create the final image FROM node:14-alpine WORKDIR /app COPY /app/dist /app/dist COPY package*.json ./ RUN npm install --production CMD ["npm", "start"]
How should I combine commands in a Dockerfile to reduce layers?
Combine related commands using &&
to execute them in a single layer, reducing the overall number of layers in your final image. This can improve build times and make your Dockerfile more efficient. For example, instead of writing separate RUN
instructions like this:
RUN apt-get update RUN apt-get install -y curl RUN apt-get install -y git RUN apt-get clean
Combine them into a single command like this:
RUN apt-get update && \ apt-get install -y curl git && \ apt-get clean
What should I include in a .dockerignore file?
The .dockerignore
file should list the files and directories that you want to exclude from the build context, preventing them from being copied to the Docker image. This can help to reduce the final image size and speed up the build process. Common items to include in a .dockerignore
file are:
.git node_modules/ *.log *.swp .DS_Store
How can I handle sensitive information in a Dockerfile?
Avoid storing sensitive information such as passwords, API keys, or other secrets directly in your Dockerfile. Instead, use environment variables or secret management tools like Docker Secrets or Kubernetes Secrets. For example, use the ENV
instruction to set an environment variable:
ENV API_KEY="your-api-key"
Or use the --build-arg
flag when building the image to pass sensitive data during the build process:
docker build --build-arg API_KEY="your-api-key" -t your-image .