Elixir Introduction
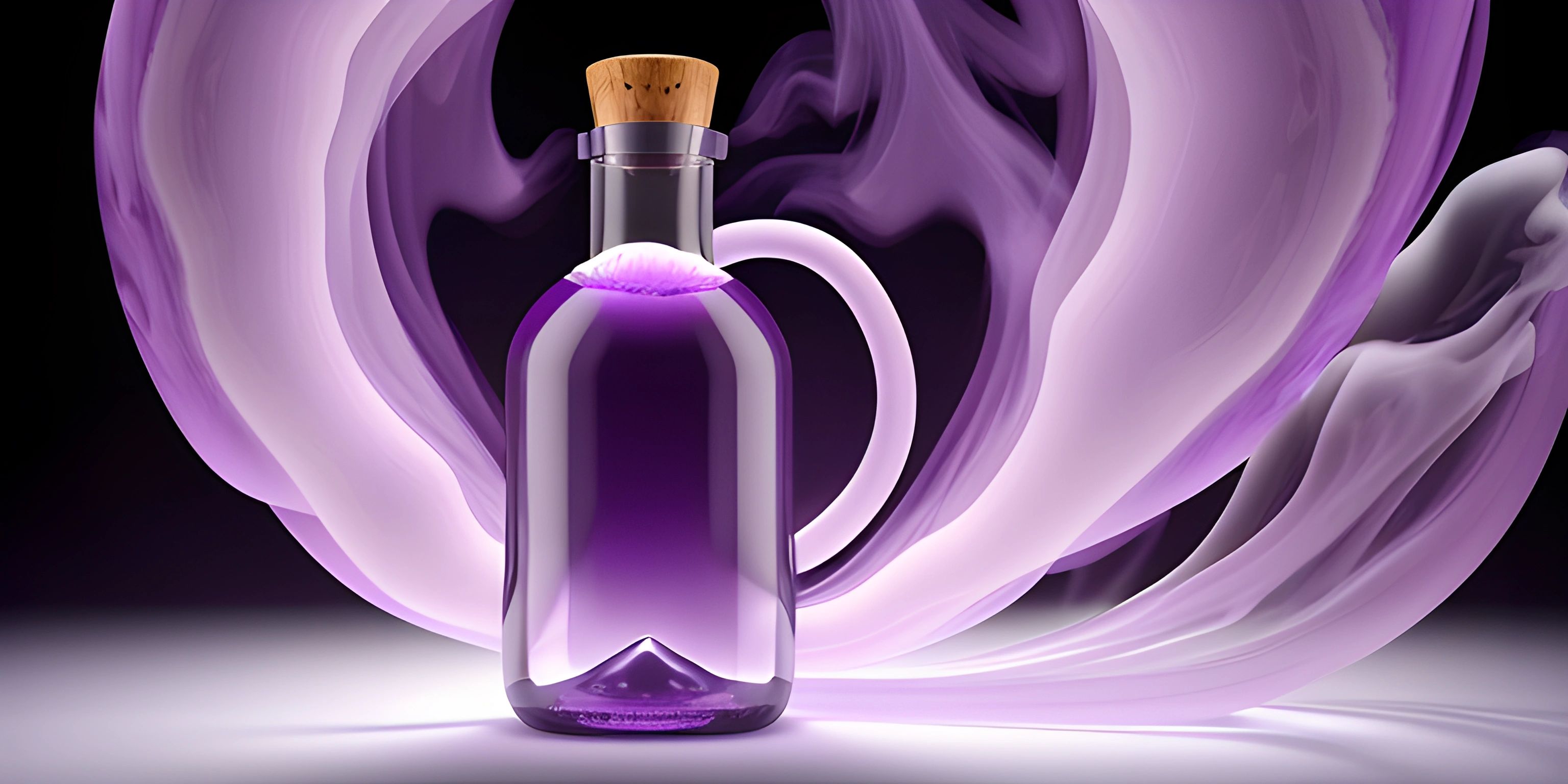
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Elixir is a dynamic, functional programming language built on top of the reliable and concurrent Erlang Virtual Machine (BEAM). It was created by José Valim in 2011 and has been gaining popularity ever since. With a friendly syntax, powerful concurrency model, and the ability to leverage existing Erlang libraries, Elixir offers a fresh approach to software development.
Functional Programming
At its core, Elixir is a functional language, which means it emphasizes the use of functions and immutable data. This can lead to cleaner, more maintainable code, as well as making it easier to reason about a program's behavior. To get a feel for Elixir's functional style, let's look at a simple example:
defmodule Greeter do def hello(name) do "Hello, " <> name end end IO.puts(Greeter.hello("Cratecode"))
In this example, we define a module Greeter
with a function hello/1
that takes a single argument, name
. The function concatenates the string "Hello, " with the name and returns the resulting string. Finally, we use the IO.puts/1
function to print the greeting to the console.
Concurrency and Fault Tolerance
One of Elixir's most notable features is its support for concurrency and fault tolerance. Elixir runs on the Erlang Virtual Machine (BEAM), which is known for its ability to handle thousands of lightweight processes efficiently. This makes Elixir an excellent choice for building highly concurrent, distributed systems.
Elixir uses a concurrency model called the Actor Model, where individual processes communicate by sending and receiving messages. This allows Elixir to isolate errors, ensuring that a single failed process won't bring down the entire system. Let's see this in action with a simple example:
defmodule Worker do def start do spawn_link(fn -> loop() end) end def loop do receive do {:work, task} -> IO.puts("Working on #{task}") loop() end end end pid = Worker.start() send(pid, {:work, "writing an Elixir article"})
Here, we define a Worker
module with a start/0
function that spawns a new process running the loop/0
function. This function waits to receive a message, and when it receives a :work
message, it prints out the task and continues to wait for more messages. We then start a worker process and send it a work message.
Metaprogramming
Elixir also provides powerful metaprogramming capabilities through its macro system. Macros allow developers to generate and manipulate code at compile-time, which can be useful for creating domain-specific languages or reducing boilerplate code. Elixir itself makes heavy use of macros to define its elegant syntax.
Here's a simple example of a macro in Elixir:
defmodule MyMacros do defmacro two_times(fun) do quote do unquote(fun) * 2 end end end defmodule MyApp do import MyMacros def double_it do two_times(3 + 4) end end IO.puts(MyApp.double_it()) #=> 14
In this example, we define a macro two_times/1
that takes a function and returns a new function that multiplies the result of the original function by 2. We then use this macro in our MyApp
module to define a double_it/0
function that doubles the result of an expression.
Conclusion
Elixir is an exciting language with a unique set of features that make it well-suited for building concurrent, fault-tolerant systems. Its functional nature encourages clean, maintainable code, while its concurrency model and macro system provide powerful tools for developers. If you're interested in learning more, be sure to check out other Cratecode articles on Elixir and dive into the vibrant Elixir community. Happy coding!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust - A Language You'll Love (psst, it's free!).
FAQ
What is Elixir and what makes it unique?
Elixir is a dynamic, functional programming language designed for building scalable and maintainable applications. It's built on top of the Erlang VM (BEAM), which provides low-latency, distributed, and fault-tolerant systems. Elixir's unique features include its elegant syntax, support for pattern matching, and concurrency model (based on lightweight processes called "actors").
How does Elixir's syntax differ from other programming languages?
Elixir's syntax is heavily influenced by Ruby, and is designed to be clean and expressive. It uses pattern matching extensively, which allows for more concise and readable code. Here's an example of a simple Elixir function definition:
defmodule HelloWorld do def greet(name) do "Hello, #{name}!" end end
What is pattern matching in Elixir and how does it work?
Pattern matching is a powerful feature in Elixir that allows you to destructure data structures and assign variables based on the structure of the data. It works by comparing the left-hand side of an assignment with the right-hand side, and attempting to find a match. If a match is found, variables on the left-hand side are bound to the corresponding values on the right-hand side. For example:
{a, b, c} = {:ok, 42, "world"}
In this case, a
will be bound to :ok
, b
to 42
, and c
to "world"
.
How does Elixir handle concurrency and parallelism?
Elixir leverages the Actor model of concurrency, which is based on lightweight processes that communicate via message passing. These processes are managed by the Erlang VM and are isolated from each other, allowing them to run concurrently and in parallel without sharing memory. This makes it easier to write concurrent code and build fault-tolerant systems.
Can I use Elixir for web development?
Absolutely! Elixir has a growing ecosystem of libraries and frameworks for web development, the most popular being Phoenix. Phoenix is a high-performance web framework that takes advantage of Elixir's capabilities to provide real-time communication, low-latency request handling, and easy scalability.