Express.js For Server-Side Rendering
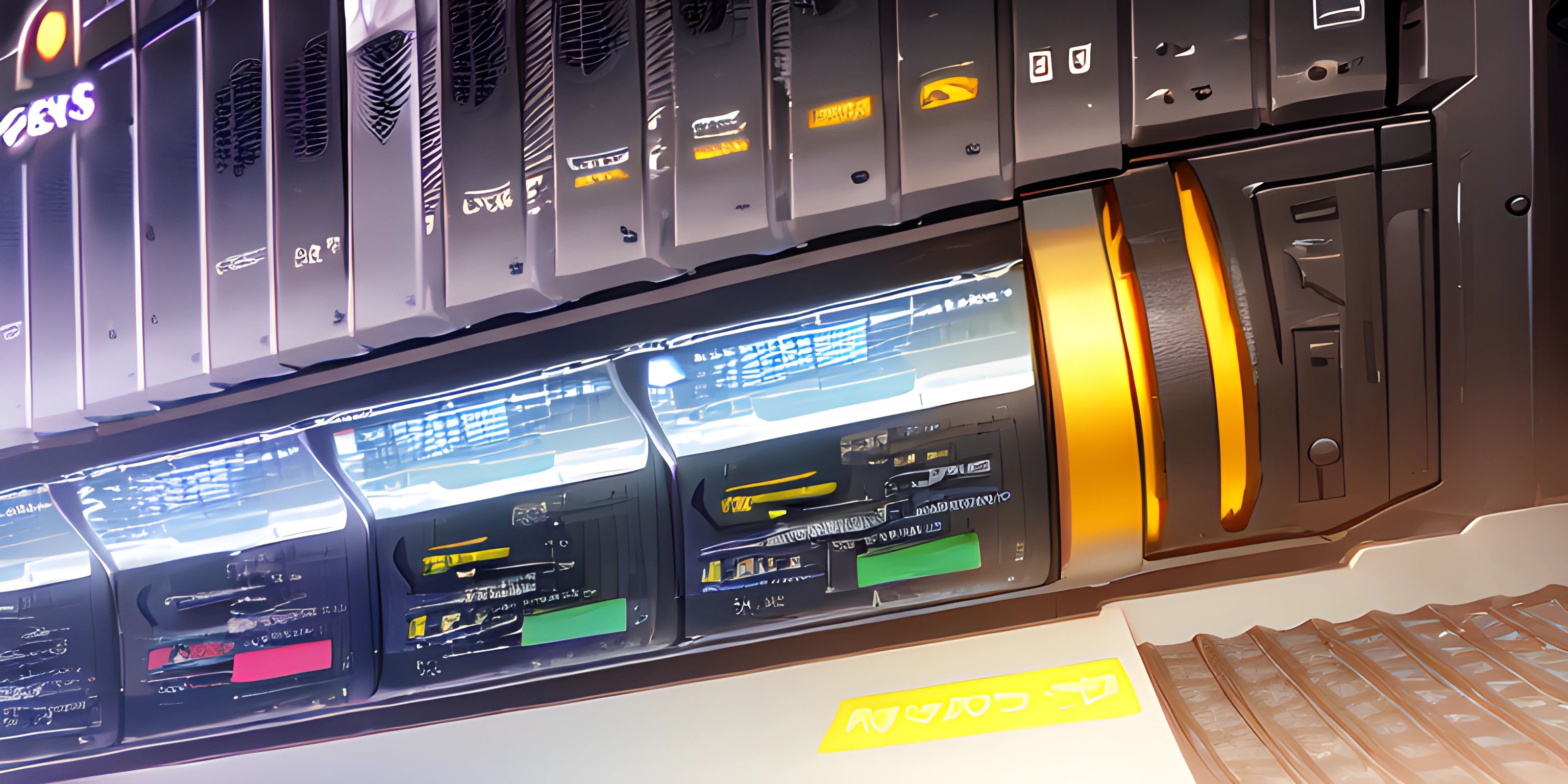
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Express.js might just ring a bell if you're familiar with the world of web development. It's a nifty framework built on top of Node.js that makes it a breeze to build web applications. Today, we're going to be using Express.js to explore the wonderland of server-side rendering.
The Concept
Before we jump in, let's first understand what server-side rendering (SSR) is. In a nutshell, it's when the server creates the entire HTML for a page in response to a user's request, instead of leaving it to the client's browser. Thanks to SSR, the user sees a fully rendered page without any laggy loading, and search engines find it easier to crawl and index your site. The tradeoff? Your server might need to hit the gym to handle the extra workload.
The Setup
To get started with Express.js for server-side rendering, you'll first need Node.js and npm installed on your machine. If Node.js is the heart of your server, npm is like its personal assistant, helping it manage packages and dependencies.
The Showtime
Once you're all set, it's time to roll up your sleeves and start writing some code. Here's how to create a basic Express.js server:
const express = require("express"); const app = express(); app.get('/', (req, res) => { res.send('Hello, Cratecode!'); }); app.listen(3000, () => { console.log("Server is running on port 3000"); });
This script will start a server at http://localhost:3000
. If you navigate to that URL, you'll see the message "Hello, Cratecode!" That's great and all, but it's not server-side rendering. Let's amp up the complexity a bit.
To implement server-side rendering, we need a way to create our HTML server-side. A handy library for this purpose is EJS. Let's install it using npm:
npm install ejs
Now let's modify our script to use EJS for server-side rendering:
const express = require("express"); const app = express(); app.set('view engine', 'ejs'); app.get('/', (req, res) => { res.render('index', {message: 'Hello, Cratecode!'}); }); app.listen(3000, () => { console.log("Server is running on port 3000"); });
Now, when you navigate to http://localhost:3000
, the server will use EJS to render an HTML file named 'index.ejs', passing it an object {message: 'Hello, Cratecode!'}
.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Full-stack Web Frameworks (Next.js) (psst, it's free!).
FAQ
What is Express.js?
Express.js is a minimal and flexible Node.js web application framework that provides a robust set of features for web and mobile applications. It's designed to make developing Node.js web applications and APIs more enjoyable and efficient.
What is server-side rendering and why is it useful?
Server-side rendering is a technique where the server generates the initial HTML of a page, allowing the browser to display a fully rendered page right away. This can improve perceived performance, provide a better user experience, and make your site more SEO-friendly.
How do I install EJS?
You can install EJS using npm, a package manager for Node.js. Just run the command npm install ejs
in your terminal. EJS, or Embedded JavaScript, is a simple templating language that lets you generate HTML markup with plain JavaScript.