Express.js Basics: Creating a Simple Server
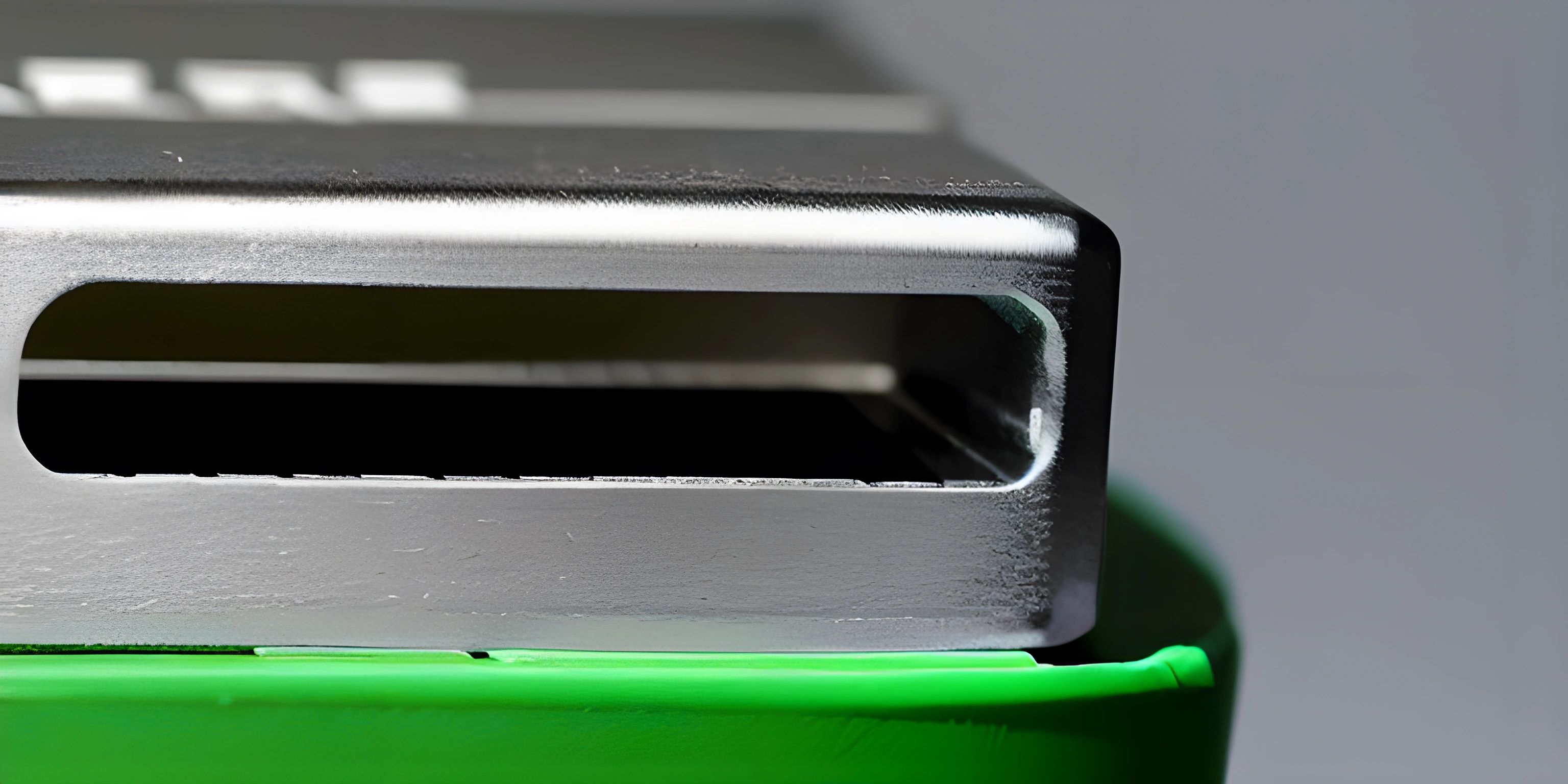
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
If you're here, it's probably because you've heard about this cool thing called Express.js and you want to make sense of it. Well, you're in the right place! Express.js is a fast, unopinionated, and minimalist web framework for Node.js. It's like the Swiss Army knife of server-side web development in the JavaScript realm.
Express.js - The Backstage Hero
Imagine you're at a rock concert. The band is your client-side JavaScript, jumping around the stage and engaging with the audience - your users. But behind the scenes, there's a crew working hard to make sure everything goes smoothly. That's your server-side JavaScript, powered by Express.js. It handles the hard work and lets the band take the spotlight.
Starting with Express.js
Starting with Express.js is like learning to ride a bicycle. It might seem tricky at first, but once you get the hang of it, you’ll be pedaling through web development in no time.
To kickstart your journey, you'll need Node.js installed on your machine. If you don't have it, hop over to the official Node.js website to download and install it.
Once Node.js is installed, you can install Express.js using npm (Node Package Manager) which comes bundled with Node.js. Open your terminal or command prompt and type the following command to install Express.js:
npm install express
Congratulations, you've just installed Express.js!
Creating a Simple Server
Now that Express.js is installed, let's create a simple server that sends a response saying 'Hello, world!' when someone visits our site. Here's how you can do it:
// Import Express.js module const express = require("express"); // Create a new Express.js application const app = express(); // Define the response for the root (/) URL app.get("/", function(req, res) { res.send("Hello, world!"); }); // Start the server on port 3000 app.listen(3000, function() { console.log("Server is running on http://localhost:3000"); });
In this script, we first import the Express.js module. We then create a new Express.js application and define a route for the root URL ("/"). When someone visits this URL, our server responds with 'Hello, world!'. Finally, we tell our server to start listening on port 3000.
That's it! You've created your first server with Express.js. You are now ready to rock the web development world!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Full-stack Web Frameworks (Next.js) (psst, it's free!).
FAQ
What is Express.js?
Express.js is an unopinionated, minimalist web framework for Node.js. It provides a robust set of features that can help you build single-page, multi-page, and hybrid web applications.
How do I install Express.js?
You can install Express.js using npm (Node Package Manager), which comes bundled with Node.js. All you need to do is open your terminal or command prompt and type npm install express
.
How do I create a server using Express.js?
Creating a server with Express.js is straightforward. You need to import the Express.js module, create a new application, define a route, and start the server on a specified port.
What is server-side rendering?
Server-side rendering (SSR) is a popular technique for rendering a client-side single-page application (SPA) on the server and then sending a fully rendered page to the client.
Why should I use Express.js for my server-side JavaScript?
Express.js is fast, unopinionated, and minimalist. It provides a simple interface for creating servers, and includes a robust set of features for web and mobile applications. It's widely used and well-documented, making it a great choice for server-side JavaScript.