Express.js Introduction
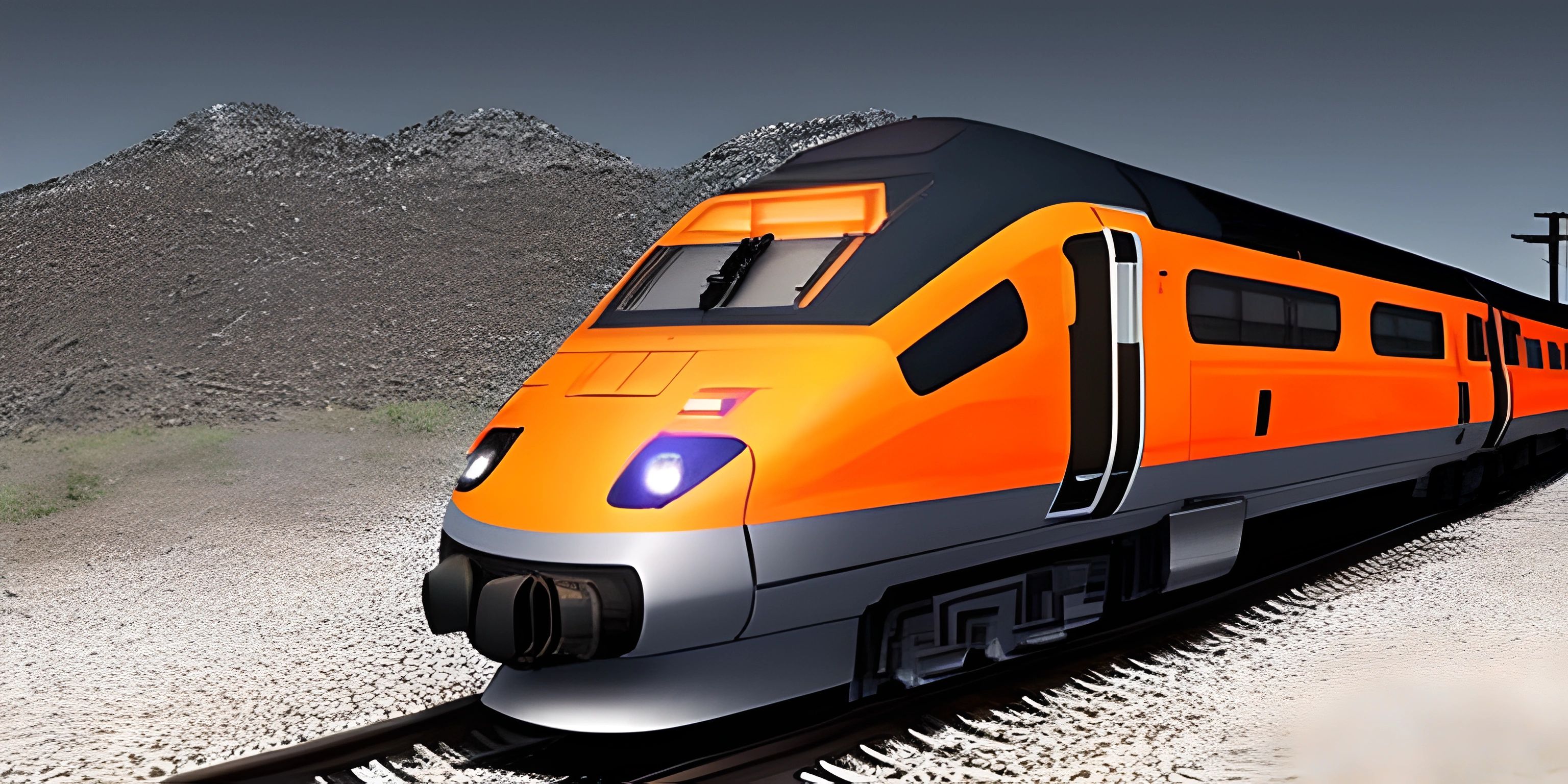
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Web development has come a long way. It is no longer about creating simple static web pages with plain HTML and CSS. Today's web applications are more dynamic, interactive, and complex. Enter the world of Node.js, a runtime environment that allows executing JavaScript on the server-side. While Node.js is powerful, using it to build web applications from scratch can be a daunting task. That's where Express.js shines, a flexible and minimalist web application framework for Node.js.
Express.js: A Bird's Eye View
Express.js is a go-to choice for many developers when building web applications using Node.js. It simplifies the process by providing a set of features that make building robust APIs and web applications a breeze. It's built on top of Node.js's native HTTP module, enhancing it with useful tools and middleware options. Express.js makes it easy to create a web server, route requests, and handle responses, making your life as a developer much more enjoyable.
Middleware
One of the core concepts in Express.js is middleware. Middleware are functions that have access to the request object (req), the response object (res), and the next middleware function in the application's request-response cycle. Middleware can execute any code, make changes to the request and response objects, or end the request-response cycle.
Middleware functions are like the gears of a well-oiled machine, each one performing a specific task as the request passes through. They can also be used for tasks like authentication, logging, or even serving static files.
Getting Started with Express.js
Setting up a basic Express.js app is quite simple. First, make sure you have Node.js installed. Next, create a new directory for your project and navigate to it using your terminal. Run the following command to initialize a new Node.js project:
npm init -y
Now, let's install Express.js using npm (Node.js package manager):
npm install express
Once Express.js is installed, create an app.js
file in your project directory and add the following code:
const express = require("express"); const app = express(); const PORT = process.env.PORT || 3000; app.get("/", (req, res) => { res.send("Hello, Express.js!"); }); app.listen(PORT, () => { console.log(`Server running at http://localhost:${PORT}`); });
This is a basic Express.js app that listens on port 3000 and responds with "Hello, Express.js!" when accessed at the root URL. Save the file and run the app using the following command:
node app.js
Open your browser and visit http://localhost:3000
. You should see the "Hello, Express.js!" message.
Routing and Beyond
Express.js provides an intuitive way to handle different routes and HTTP methods. In our example above, we used app.get()
to handle a GET request to the root path. Express.js supports all HTTP methods like GET
, POST
, PUT
, DELETE
, and more. You can create routes to handle different paths and even use route parameters to capture values in the URL.
Express.js is just the beginning of a fantastic journey into the world of Node.js web development. With its powerful features, extensibility through middleware, and an active community, there's no limit to what you can build. Whether you're creating a simple API or a full-fledged web application, Express.js is an invaluable tool that makes web development with Node.js a pleasure. So go ahead, give it a try, and see for yourself how Express.js can streamline your web development process.
FAQ
What is Express.js and why is it important in Node.js web development?
Express.js is a popular and lightweight web application framework for Node.js, which simplifies the process of building web applications and APIs. It provides a set of robust features that make it easier to create and manage routes, handle HTTP requests and responses, and create middleware functions. Express.js is important in Node.js web development because it enables developers to build web applications and APIs quickly and efficiently, while maintaining a high level of flexibility and customization.
How do I install and set up Express.js in my Node.js project?
To install Express.js, you first need to have Node.js and npm (Node Package Manager) installed on your system. Once you have those, follow these steps:
- Create a new directory for your project and navigate to it in your command line.
- Run
npm init
to create apackage.json
file for your project. - Install Express.js by running the command:
npm install express --save
- Create an
app.js
file in your project directory. - In the
app.js
file, import Express.js and create an instance of the Express app:const express = require("express"); const app = express();
Now, you're all set to start building your Express.js web application or API!
How can I create and manage routes in Express.js?
In Express.js, you can create routes by defining various HTTP methods (GET, POST, PUT, DELETE, etc.) on the app instance. You need to specify the endpoint (path) and a callback function that takes req
(request) and res
(response) as parameters. Here's an example:
app.get("/", (req, res) => { res.send("Welcome to our Express.js app!"); });
To manage multiple routes, you can use Express.js Router, which allows you to create modular and mountable route handlers. First, create a Router instance and define your routes on it:
const express = require("express"); const router = express.Router(); router.get("/example", (req, res) => { res.send("This is an example route."); }); router.post("/example", (req, res) => { res.send("This is an example POST route."); });
Then, you can import the Router in your app.js
and use it as middleware:
const exampleRoutes = require("./routes/exampleRoutes"); app.use("/api", exampleRoutes);
What are middleware functions in Express.js and how do I create them?
Middleware functions are functions that have access to the request object (req
), the response object (res
), and the next middleware function in the application's request-response cycle. These functions can be used to execute any code, make changes to the request and response objects, or end the request-response cycle.
To create a middleware function, you need to define a function with three parameters: req
, res
, and next
. Here's an example:
function logRequestInfo(req, res, next) { console.log(`Request received: ${req.method} ${req.url}`); next(); }
To use this middleware function, add it to your app using the app.use()
function:
app.use(logRequestInfo);
This will make the middleware function execute for every request that hits your app. You can also apply middleware to specific routes or route groups by passing them as arguments before the callback function:
app.get("/example", logRequestInfo, (req, res) => { res.send("This route uses the logRequestInfo middleware."); });
Remember to always call next()
at the end of your middleware function, unless you want to terminate the request-response cycle.