Next.js for Server Side Rendering
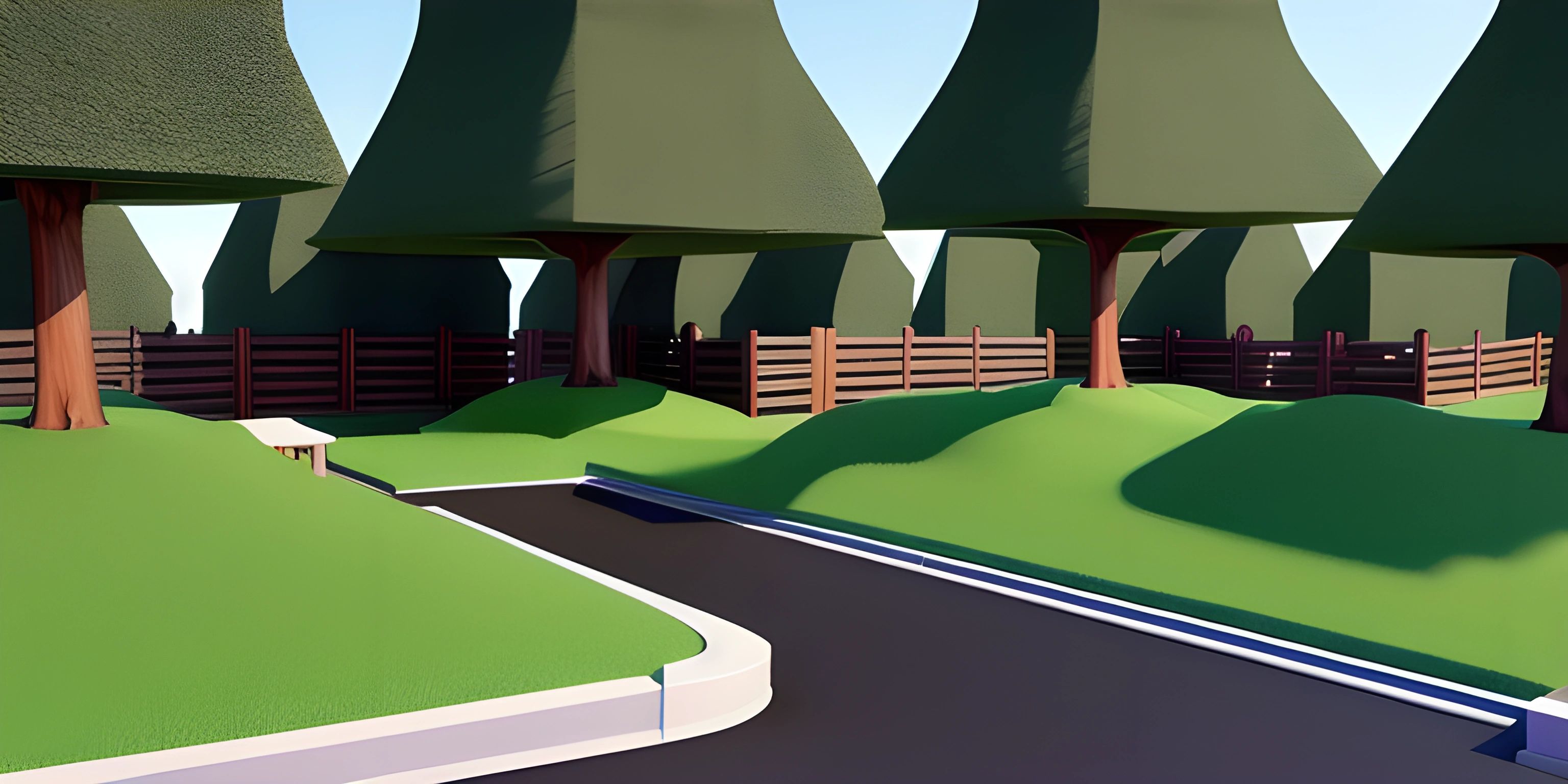
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Before we delve into the magical world of server side rendering with Next.js, let's take a moment to appreciate the beauty of JavaScript. JavaScript, the language that laughs at the face of the server-client divide and says, "I can be both!" It's the Swiss Army Knife of the programming world, always there when you need to cut through the clutter of the web. But sometimes, even the Swiss Army Knife needs a little extra power. That's where Next.js comes into play.
Server Side Rendering with Next.js
Next.js is a React framework that makes server-side rendering a breeze. But why server-side rendering (SSR), you ask? Well, consider the impatient web surfer who wants their webpage and wants it now. With traditional client-side rendering, our surfer has to wait for the entire JavaScript bundle to load before anything meaningful shows up on their screen. That's like waiting for your entire pizza to cook before you can start eating. Who has the patience for that?
With SSR, your server does the heavy lifting and sends a fully rendered HTML page to the client. That's like getting a slice of pizza while the rest of it is still cooking. Faster initial load times, better SEO, and improved performance - that's the power of SSR!
Here's a simple example of how you create a Next.js app with server-side rendering:
import React from 'react'; class HomePage extends React.Component { static async getInitialProps({ req }) { // This function runs on server side and fetches data before page is served const userAgent = req ? req.headers['user-agent'] : navigator.userAgent; return { userAgent }; } render() { return ( <div> Hello World. You are visiting from "{this.props.userAgent}" </div> ); } } export default HomePage;
In the code above, getInitialProps
is a special function in Next.js that runs on the server side before the page is served. It fetches the necessary data and passes it as props to the page. This is the heart of server-side rendering in Next.js.
SSR vs CSR
It's not always sunshine and rainbows with SSR, as it comes with its own set of challenges. Increased server load, complexity of code, and potentially slower time-to-interactive are some of the trade-offs. That's why it's important to understand when to use SSR and when to stick with client-side rendering (CSR).
Remember, SSR is like getting a slice of pizza while the rest of it is still cooking. But sometimes, you need the whole pizza to fully enjoy the experience. Similarly, for applications that are not dependent on SEO and where user interaction is more important than initial load time, CSR might be a better choice.
FAQ
What benefits does server-side rendering offer?
Server-side rendering (SSR) offers a number of benefits. First, it can lead to faster initial load times because the server sends a fully rendered HTML page to the client. Second, it can lead to improved SEO because search engine crawlers can easily parse and index the server-rendered HTML. Finally, SSR can lead to improved performance, particularly for users with slower internet connections or less powerful devices.
What are some of the trade-offs of server-side rendering?
Server-side rendering can increase the load on your server, as the server has to do more work to render the HTML pages. It can also make your code more complex, as you have to handle both server-side and client-side rendering. Additionally, while SSR can lead to faster initial load times, it may also lead to slower time-to-interactive, as the client may have to wait for JavaScript to load before they can interact with the page.
When should I use server-side rendering and when should I use client-side rendering?
Server-side rendering is a good choice for websites where SEO is important or when you want to provide fast initial load times. On the other hand, client-side rendering is a good choice for applications where user interaction is more important than initial load time, or when you want to offload some of the rendering work to the client to reduce server load.