Flask Framework
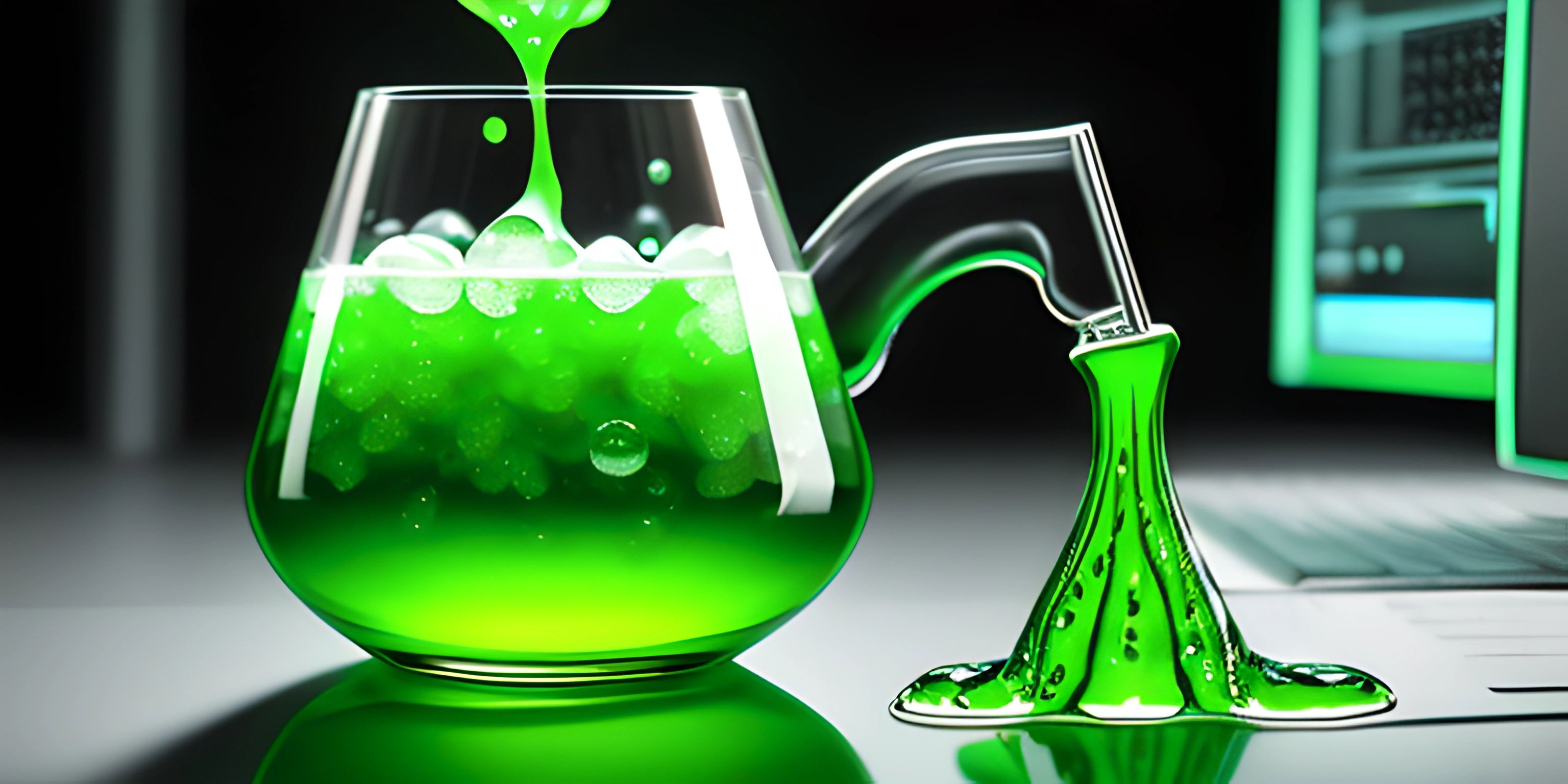
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Flask is a lightweight and flexible Python framework for web development. It allows developers to create web applications quickly, offering a minimalistic approach that doesn't impose strict rules or requirements. Flask is often considered the perfect starting point for beginners, as well as experienced developers seeking a simple and efficient tool for building web applications.
Getting Started with Flask
Before diving into Flask, make sure you have Python installed on your system. Flask requires Python 3.5 or later. Once you have Python installed, you can install Flask using pip
, Python's package manager:
pip install Flask
With Flask installed, you're ready to create your first web application!
A Simple Flask Application
A basic Flask application consists of a single Python file with a few lines of code. Let's create a simple app that displays "Hello, World!" when accessed through a web browser.
Create a new file named app.py
and add the following code:
from flask import Flask app = Flask(__name__) @app.route('/') def hello_world(): return 'Hello, World!' if __name__ == '__main__': app.run()
Let's break down this code:
- First, we import the
Flask
class from theflask
module. - Next, we create an instance of the
Flask
class, passing__name__
as an argument. This tells Flask to use the current module as the starting point for the application. - We define a function
hello_world()
that returns the string "Hello, World!". This function will be called when a client requests the root URL (/
) of the application. - The
@app.route('/')
decorator above thehello_world()
function tells Flask to call this function when the specified URL is requested. - Finally, the
if __name__ == '__main__':
block tells Python to run the application when the script is executed directly (not imported as a module).
To run the application, open a terminal, navigate to the folder containing app.py
, and run the following command:
python app.py
You should see output similar to this:
* Running on http://127.0.0.1:5000/ (Press CTRL+C to quit)
Open your web browser and visit http://127.0.0.1:5000/. You should see the message "Hello, World!" displayed on the page.
Congratulations, you've successfully created your first Flask application!
Expanding Your Flask Application
Flask offers many features and extensions to help you build more complex web applications. Some of these include:
- Template rendering: Use the Jinja2 template engine to create dynamic HTML pages.
- URL routing: Define custom URL routes and capture URL parameters.
- Form handling: Process and validate user input from HTML forms.
- Session management: Store and retrieve user-specific data across multiple visits.
- Database integration: Connect to databases and perform CRUD operations using Flask.
As you become more familiar with Flask, you can explore these features and many others to build powerful and efficient web applications tailored to your needs.
Conclusion
The Flask framework is a versatile and lightweight tool for Python web development. Its minimalistic approach makes it easy to learn and use, making it an excellent choice for both beginners and experienced developers. As you continue to explore Flask and its capabilities, you'll discover that it's a powerful ally in creating robust web applications. So, gear up and get ready to conquer the web with Flask!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Full-stack Web Frameworks (Next.js) (psst, it's free!).
FAQ
What is Flask Framework?
Flask is a lightweight Python web development framework that allows developers to easily build web applications.
How do I install Flask?
To install Flask, simply run pip install Flask
in your terminal or command prompt.
What are the main features of Flask?
Flask offers features like URL routing, template engine, request handling, and support for third-party extensions.
Can Flask be used for large-scale projects?
Flask is primarily designed for small to medium-sized projects, but with proper structuring and use of extensions, it can be used for larger projects as well.
How does Flask compare to Django?
Flask is more lightweight and flexible compared to Django, which is a full-fledged web development framework. Flask is a better choice for smaller projects or when more control over components is desired.