Django Introduction
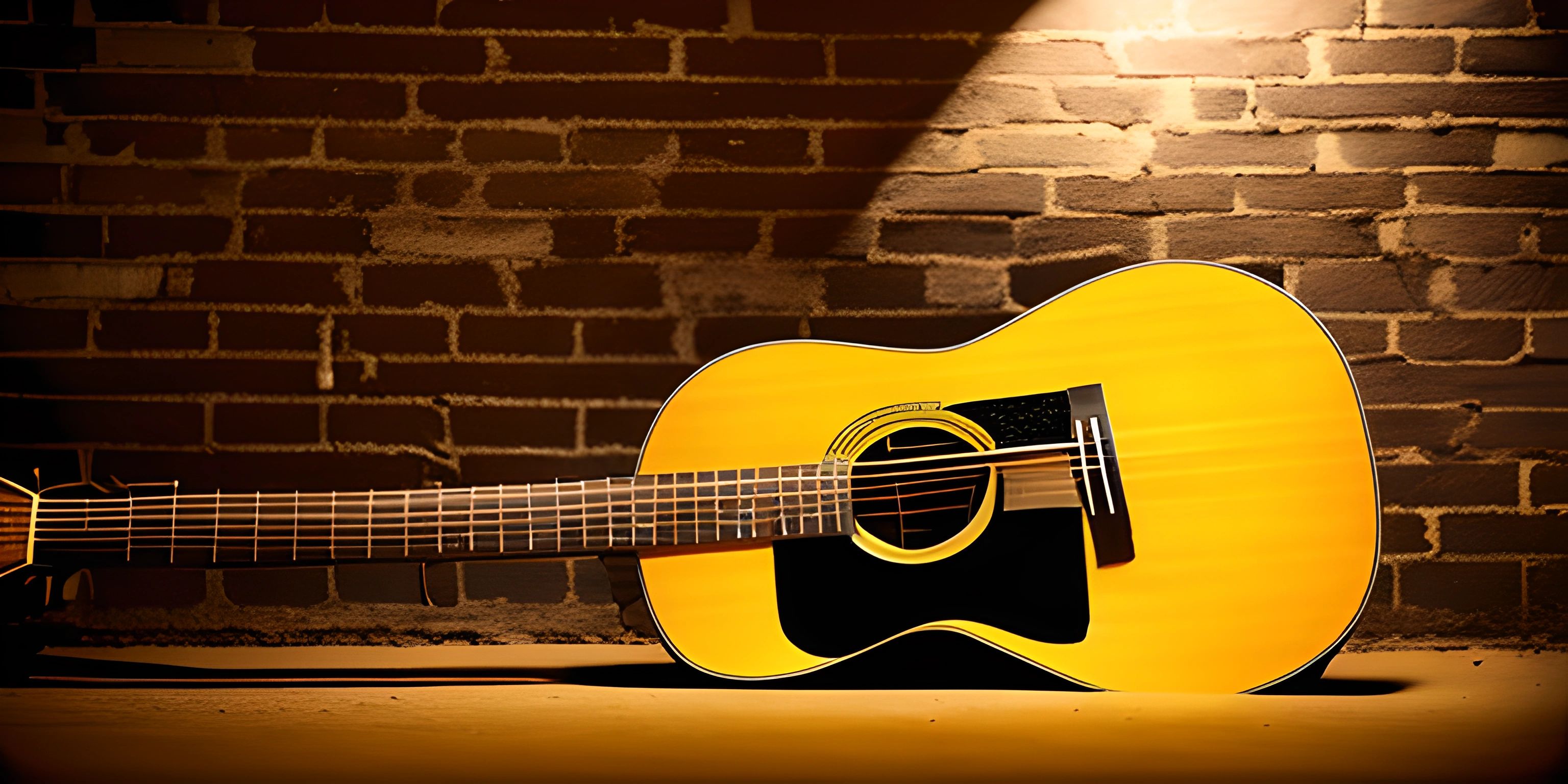
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Django is a high-level Python web framework that encourages rapid development and clean, pragmatic design. It's built for developers who want to build robust and scalable web applications without reinventing the wheel. Django takes care of much of the hassle of web development, allowing you to focus on writing your app without needing to start from scratch.
Django's Architecture
The Django framework follows the Model-View-Template (MVT) architectural pattern, which is a variation of the Model-View-Controller (MVC) pattern. In MVT, the Model represents the data structure, the View is responsible for displaying the data, and the Template formats the data for presentation. This architecture allows for a clear separation of concerns and promotes reusability of code.
Model
In Django, the model represents the structure of the data in your application. It defines the fields and their data types, as well as any relationships between tables in the database. The model also handles data validation, ensuring that only valid data is saved to the database.
View
The view is responsible for handling HTTP requests and returning HTTP responses. It takes a request, processes it, and returns a response, usually by rendering a template with the data passed to it. Views can also handle form submissions, handle user authentication, and perform other tasks related to handling user interactions with your web application.
Template
A template in Django is a text file that defines the structure and layout of the HTML that will be sent to the user's browser. Templates use a simple, easy-to-learn syntax to define placeholders for dynamic content, as well as basic looping and conditional logic. This allows you to separate the presentation of your data from the processing and retrieval of that data.
Getting Started with Django
To start a new Django project, you'll first need to install Django by running the following command:
pip install django
Next, you can create a new Django project by running:
django-admin startproject myproject
This will create a new directory called myproject
with the following structure:
myproject/ manage.py myproject/ __init__.py settings.py urls.py asgi.py wsgi.py
The manage.py
file is a command-line utility for managing your Django project. You'll use it to run development servers, create database tables, and perform other tasks related to managing your project.
The settings.py
file contains settings for your Django project, such as database configuration, timezone settings, and static file locations.
The urls.py
file is where you define the URL patterns for your project. This file tells Django which views to call when a user requests a specific URL.
To run your Django project, navigate to the project directory and run the following command:
python manage.py runserver
This will start a development server at http://127.0.0.1:8000/
. If you visit this URL in your browser, you should see the default Django welcome page.
Final Thoughts
Django is a powerful and versatile web framework that makes it easy to build web applications quickly and efficiently. By following the Model-View-Template architecture, Django promotes clean and reusable code, making your projects easier to maintain and expand as they grow. With Django's extensive documentation and thriving community, you'll have all the support you need to build your next great web application.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Full-stack Web Frameworks (Next.js) (psst, it's free!).