Introduction to Django for Python Web Development
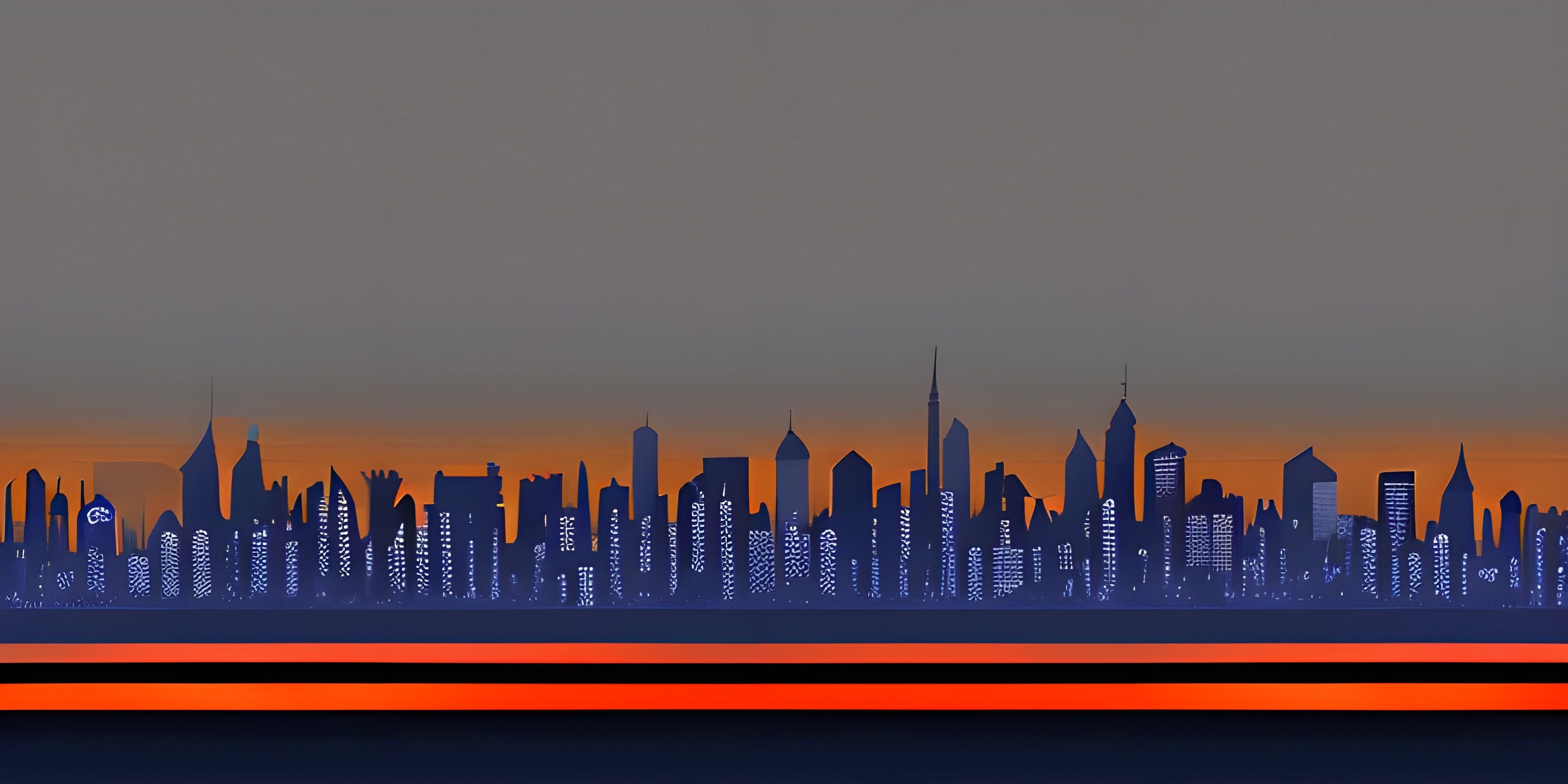
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Dive headfirst into the world of Django, a powerful web framework that makes Python web development a breeze. By the end of this adventure, you'll have a solid understanding of what Django is, how it works, and how you can harness its power to create your own web applications.
What is Django?
Django is a high-level Python web framework that promotes rapid development and clean, pragmatic design. Built by experienced developers, Django takes care of much of the hassle of web development, so you can focus on writing your app without needing to reinvent the wheel. It’s free and open source, which means you can use it, study it, and even modify it without any restrictions.
Django follows the Model-View-Controller (MVC) architectural pattern, which encourages separation of an application's concerns into three distinct components. In Django, these components are slightly modified and are known as Model-View-Template (MVT).
Model
The Model is the representation of your application's data structure. It defines the structure of your database tables and the relationships between them. In Django, models are created using Python classes, and the framework provides a powerful Object-Relational Mapping (ORM) system to interact with the database.
View
The View is responsible for defining what data is displayed and how it is presented to the user. In Django, views are created using Python functions or classes, which receive input from the user, process it, and return a response. This response can be an HTML page, a JSON object, or any other type of data.
Template
The Template is the presentation layer of your application. It is where you define the HTML structure and layout of your web pages. Django's template system allows you to create reusable templates that can be filled with data from your views. This keeps your HTML code clean and separate from your Python code.
Getting Started with Django
To start using Django, you'll first need to install it. Make sure you have Python installed on your system, and then run the following command:
pip install django
Once Django is installed, you can create a new Django project using the following command:
django-admin startproject my_project
Replace my_project
with the name of your project. This will create a new directory with the same name as your project, containing the necessary files and directories to get started.
Now, navigate to your project directory and run the following command to start the development server:
python manage.py runserver
If everything is set up correctly, you should see a message saying that the development server is running. Open your browser and go to http://127.0.0.1:8000/
to see the default Django welcome page.
Creating a Simple Web App
To create a simple web app, you'll need to make use of Django's URL routing and views. Here's a basic example:
-
In your project directory, create a new file called
views.py
and add the following code:from django.http import HttpResponse def hello(request): return HttpResponse("Hello, Django!")
This code defines a simple view called
hello
that returns a plain-text HTTP response. -
Create a new file called
urls.py
in the same directory and add the following code:from django.urls import path from . import views urlpatterns = [ path('hello/', views.hello, name='hello'), ]
This code sets up URL routing for your project, mapping the
/hello/
URL to thehello
view. -
Finally, open the
urls.py
file in your project's main directory (the one containingsettings.py
), and modify theurlpatterns
list to include your new URL routing:from django.contrib import admin from django.urls import include, path urlpatterns = [ path('admin/', admin.site.urls), path('', include('my_project.urls')), ]
Replace
my_project
with the name of your project.
Now, restart your development server and visit http://127.0.0.1:8000/hello/
in your browser. You should see the message "Hello, Django!" displayed.
Congratulations! You've just created your first Django web app. From here, the possibilities are endless. Explore Django's documentation and other Cratecode articles to learn more about this powerful framework and build your own amazing web applications. Happy coding!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Full-stack Web Frameworks (Next.js) (psst, it's free!).
FAQ
What is Django and why should I use it for Python web development?
Django is a high-level web framework for Python that allows you to rapidly build web applications. It follows the Model-View-Controller (MVC) design pattern and encourages reusable, clean, and modular code. Django is popular due to its extensive built-in features, strong community support, and ease of use. By using Django, you can speed up the web development process and create robust, scalable applications.
How does Django's Model-View-Controller (MVC) design pattern work?
In Django's MVC design pattern:
- Model: Represents the database schema and handles data validation, storage, and retrieval. It defines the structure of your data, including fields, relations, and constraints.
- View: Handles user interactions and renders the appropriate response (often an HTML page). It defines what data needs to be displayed and how the data should be presented.
- Controller: Manages the flow of data between the Model and the View. In Django, the controller's role is handled by the framework itself via URL routing and middleware. This separation of concerns allows for better code organization, maintainability, and scalability of your web application.
What are some built-in features that Django offers?
Django offers a wide range of built-in features that help streamline web development, including:
- Object-relational mapping (ORM): Allows for easy database queries and management using Python objects, without writing raw SQL.
- Authentication and authorization: Provides a ready-to-use user authentication system, including user registration, login, and permissions management.
- URL routing: Enables clean and readable URLs, making it easy to map URLs to corresponding views.
- Admin interface: Offers a fully customizable, auto-generated admin panel for managing your application's data.
- Form handling: Simplifies form creation, validation, and processing.
- Built-in support for internationalization and localization.
- Caching system for improved performance.
How do I get started with Django for my Python web development project?
To get started with Django, follow these steps:
- Install Django using pip:
pip install django
- Create a new Django project:
django-admin startproject myproject
- Change directory to your project folder:
cd myproject
- Run the development server:
python manage.py runserver
- Open your browser and go to
http://localhost:8000/
to see your new Django web application. From here, you can start building your application by defining models, views, and templates, and configuring the URL routing. Check out the official Django documentation for more detailed guidance and tutorials: Django Documentation