Genetic Algorithms: Selection Techniques
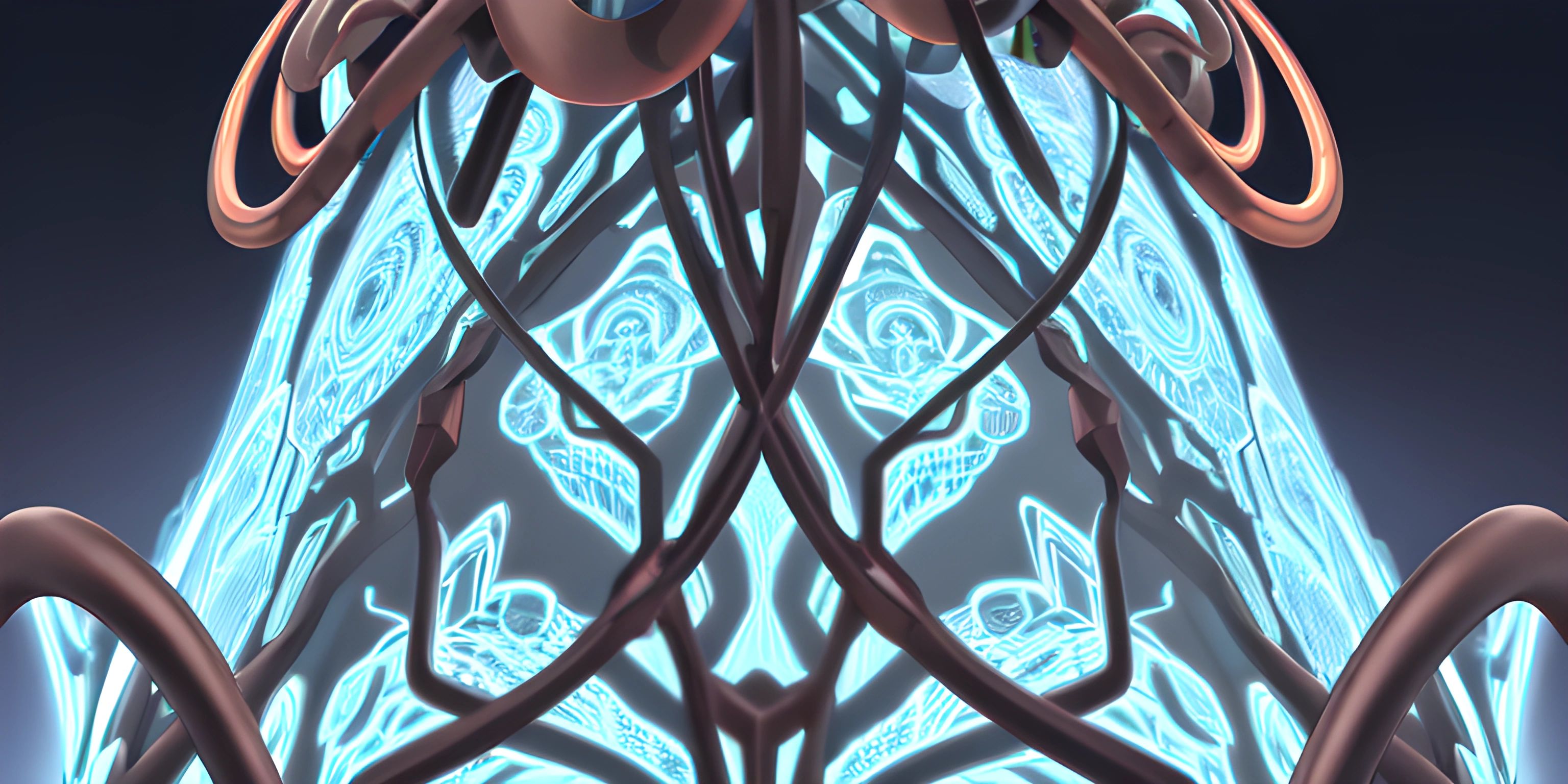
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Genetic algorithms are fascinating creatures! They incorporate the principles of evolution and natural selection into the world of computation, allowing us to solve complex problems in novel ways. One of the central parts of any genetic algorithm is the selection process, where we choose which individuals get to pass on their genes to the next generation.
The Importance of Selection
Think of the selection process in genetic algorithms like a high-stakes game show. In each round, some of the contestants (or in our case, solutions) will be selected to go on to the next round, while the others will be eliminated. But instead of a cheesy game show host, the selection process is guided by a complex set of rules, or selection techniques, which decide who gets to stay and who has to leave.
Roulette Wheel Selection
The first selection technique we're going to talk about is the Roulette Wheel Selection. Imagine a roulette wheel where each solution has a spot on the wheel. The size of the spot is determined by the fitness of the solution – the fitter the solution, the bigger the spot. The wheel is spun and whichever solution the ball lands on is selected to pass on its genes.
Here's a simple example:
def roulette_wheel_selection(population): max_fitness = sum(individual.fitness for individual in population) pick = random.uniform(0, max_fitness) current = 0 for individual in population: current += individual.fitness if current > pick: return individual
This code calculates the total fitness of the population, picks a random number between 0 and the total fitness, then goes through the population and adds up the fitness until it reaches the picked number. The individual whose fitness pushed the total over the pick is selected.
Tournament Selection
Another technique is Tournament Selection. It's like a mini-game within our game show, where a few solutions are randomly selected to compete against each other in a tournament. The winner of the tournament (the solution with the highest fitness) is then selected to pass on its genes.
def tournament_selection(population, tournament_size): tournament = random.sample(population, tournament_size) return max(tournament, key=lambda x: x.fitness)
In this example, a subset of the population is chosen randomly for the tournament. The fittest individual in this subset is selected.
Rank Selection
Lastly, let's talk about Rank Selection. In this technique, solutions are ranked based on their fitness, and the chance of being selected is proportional to their rank, not their absolute fitness value. This can be useful when the fitness values vary widely.
def rank_selection(population): population.sort(key=lambda x: x.fitness) rank_sum = sum(range(len(population))) pick = random.uniform(0, rank_sum) current = 0 for i, individual in enumerate(population): current += i if current > pick: return individual
In this code, the population is sorted by fitness, a random number between 0 and the sum of all ranks is picked, and individuals are selected proportionally to their rank.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Full-stack Web Frameworks (Next.js) (psst, it's free!).
FAQ
What is the importance of selection in genetic algorithms?
In genetic algorithms, selection refers to the process of choosing which individuals in the current generation get to pass on their genes to the next generation. The selection process is a key aspect of genetic algorithms as it influences the quality of the solutions found and the speed at which the algorithm converges to the optimal solution.
What is Roulette Wheel Selection in genetic algorithms?
Roulette Wheel Selection is a selection technique in genetic algorithms where each individual in the population is assigned a slice of a circular "roulette wheel." The size of each individual's slice is proportional to its fitness. A random number is then generated that determines which individual's slice the "ball" lands on, and that individual is selected.
How does Tournament Selection work in genetic algorithms?
Tournament Selection is a selection technique in genetic algorithms where a subset of individuals is chosen randomly from the population, and the individual with the highest fitness in this subset is selected. The process can be repeated as many times as necessary until the desired number of parents is chosen.
What is Rank Selection in genetic algorithms and when is it useful?
Rank Selection is a selection technique in genetic algorithms where individuals are selected based on their rank rather than their absolute fitness value. This is especially useful when the fitness values vary widely, as it helps prevent premature convergence by ensuring a more uniform spread of selection pressure.
Can these selection techniques be used together in a single genetic algorithm?
Yes, these selection techniques can be used together in a single genetic algorithm. For instance, you might use Roulette Wheel Selection for one part of the selection process and then use Tournament Selection for another part. The choice of selection techniques depends on the specific needs of the problem being solved.