Python Language Overview
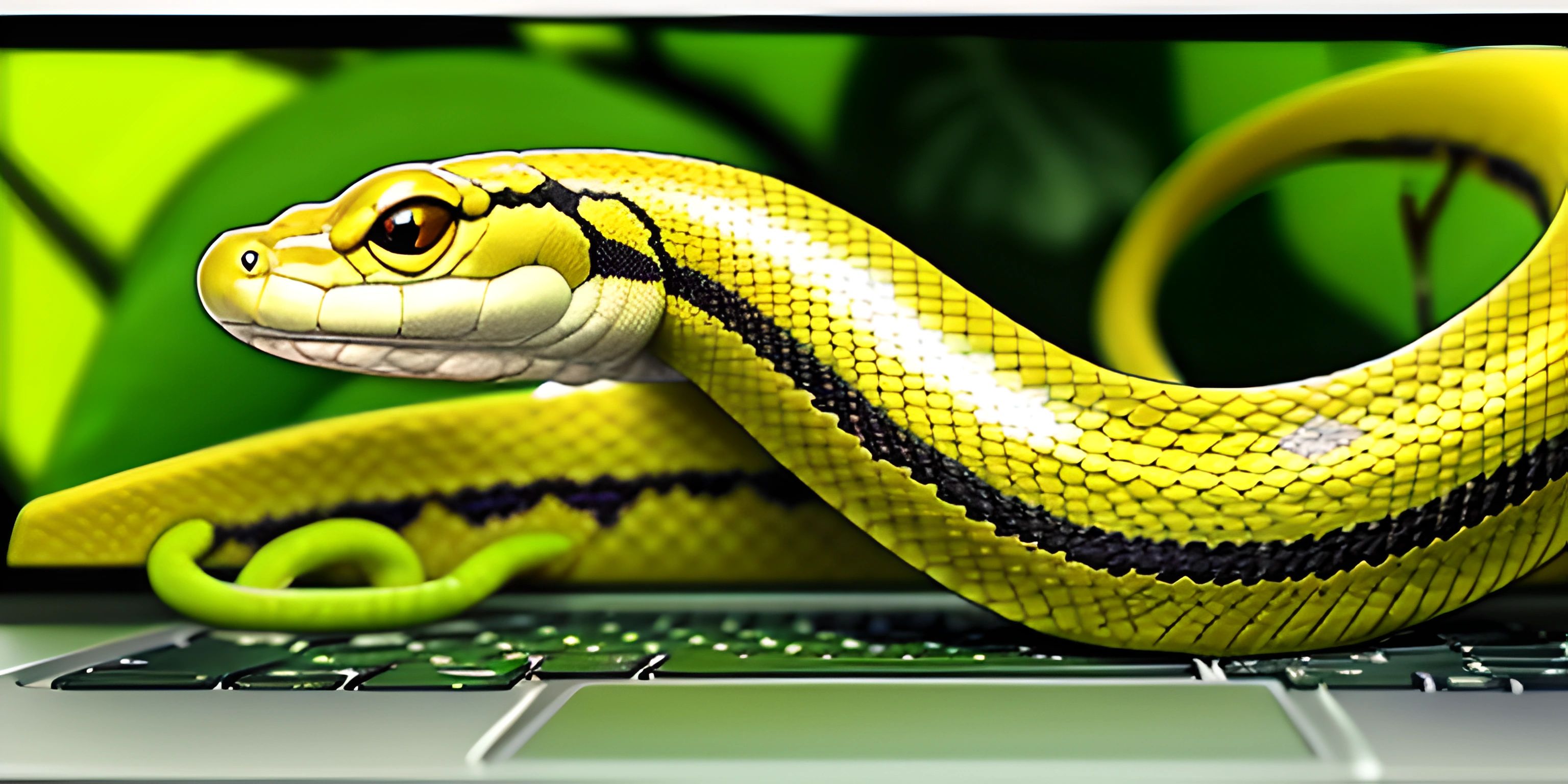
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Python is a popular, high-level, easy-to-learn programming language that has been around since the 1990s. It was created by Guido van Rossum, who wanted a language that was easy to read and write, with a focus on simplicity and readability. Today, Python is used for a wide range of applications, including web development, data analysis, artificial intelligence, and more. Let's dive into some of the features that make Python so beloved by developers.
Readability and Syntax
One of Python's main strengths is its readability. Its syntax is clean and easy to understand, even for beginners. It uses indentation to define code blocks, unlike other languages that rely on brackets or other symbols. This makes Python code appear less cluttered and more pleasant to read. Here's an example of a simple Python function that calculates the sum of two numbers:
def add_numbers(a, b): sum = a + b return sum result = add_numbers(1, 2) print(result) # Outputs: 3
Built-In Data Types
Python comes with a variety of built-in data types that make it easy to work with different kinds of data. Some of the most common data types include:
int
: Represents integers (whole numbers).float
: Represents floating-point numbers (numbers with a decimal point).str
: Represents strings (sequences of characters).list
: Represents ordered, mutable collections of items.tuple
: Represents ordered, immutable collections of items.dict
: Represents unordered collections of key-value pairs.bool
: Represents boolean values (True
orFalse
).
Here's an example of how you might use these data types in a Python program:
# Using different data types in Python age = 25 # int height = 1.75 # float name = "Alice" # str grades = [90, 85, 75, 80] # list colors = ("red", "green", "blue") # tuple person = {"name": "Bob", "age": 30} # dict is_happy = True # bool
Libraries and Modules
One of the reasons why Python is so versatile is its extensive library of modules. Modules are collections of pre-written code that you can import into your projects to perform specific tasks without having to write the code from scratch. Python has a large standard library that includes modules for working with files, regular expressions, networking, and more. Additionally, there are thousands of third-party libraries available for Python, covering a wide range of applications.
Here's an example of how to use the math
module from Python's standard library to calculate the square root of a number:
import math number = 9 sqrt = math.sqrt(number) print(sqrt) # Outputs: 3.0
Community and Ecosystem
Python has a large and active community of developers who contribute to its growth and success. This community is responsible for creating and maintaining the numerous libraries and frameworks available for Python, as well as providing support and resources for new developers.
There are also many resources available for learning Python, such as tutorials, online courses, books, and more. This makes it easy for beginners to get started and for experienced developers to deepen their knowledge of the language.
Conclusion
Python's readability, extensive library, and strong community make it a powerful programming language that is easy to learn and use in a wide range of applications. From web development to data analysis and artificial intelligence, Python's versatility and simplicity make it a popular choice for developers around the world. If you're looking to learn a programming language or expand your skill set, Python is definitely worth exploring.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust - A Language You'll Love (psst, it's free!).
FAQ
What is Python and why is it so popular?
Python is a high-level, interpreted programming language known for its simplicity and readability. It is popular due to its versatility, extensive library support, and strong community. Python is used in various domains, including web development, data analysis, artificial intelligence, and more.
How do I install Python on my computer?
To install Python, visit the official Python website at python.org and download the appropriate installer for your operating system. Run the installer and follow the instructions. Make sure to check the box to add Python to your system's PATH during installation.
What are some basic Python syntax rules?
Here are some basic Python syntax rules:
- Python uses indentation (usually 4 spaces) to define code blocks.
- Statements are terminated by a newline character, but you can use a semicolon (;) to put multiple statements on a single line.
- Comments start with a hash symbol (#) and extend to the end of the line.
- Variables are case-sensitive and can be assigned without declaring their type.
- Python uses double quotes (") for strings, though single quotes (') can be used as well.
How do I write a simple Python program?
To write a simple Python program, create a new text file with the extension ".py" (e.g., "hello_world.py"). Open the file in a text editor and write the following code:
print("Hello, World!")
Save the file and run it using the Python interpreter by opening a terminal (or command prompt) and executing the command python hello_world.py
. This will print "Hello, World!" to the console.
What are some built-in data types in Python?
Python has several built-in data types, including:
- Numeric: int (integer), float (floating-point), and complex (complex numbers)
- Sequence: str (string), list (ordered, mutable sequence), and tuple (ordered, immutable sequence)
- Mapping: dict (dictionary, an unordered collection of key-value pairs)
- Set: set (unordered collection of unique items) and frozenset (immutable set)
- Boolean: bool (true or false)