Node.js Overview
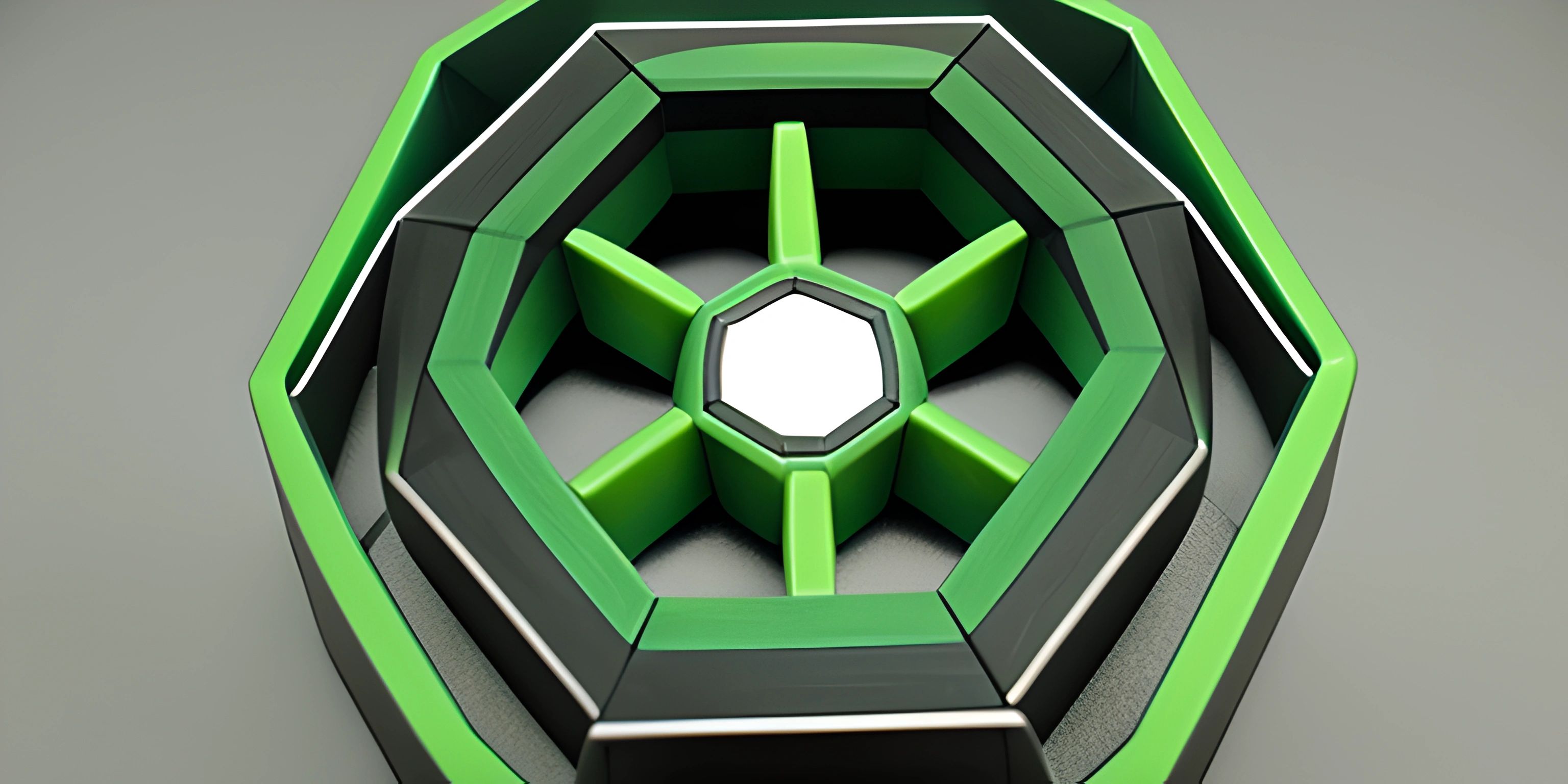
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Node.js presents a twist on the traditional JavaScript narrative - taking the language from the browser to the server. By leveraging the power of the V8 JavaScript Engine, Node.js has created a runtime environment that unlocks server-side development with JavaScript, changing the game for web developers everywhere.
From Browser to Server
JavaScript, created in the '90s, was initially designed for client-side web development within browsers. However, as web applications grew in complexity, developers craved a way to utilize JavaScript on the server-side too. This is where Node.js comes into play.
Created by Ryan Dahl in 2009, Node.js allows developers to run JavaScript code outside of a browser, enabling the creation of server-side applications and APIs using the language they already know and love.
The V8 JavaScript Engine
The power behind Node.js lies in the V8 JavaScript Engine, an open-source high-performance runtime engine developed by Google. It is responsible for compiling JavaScript directly into machine code, resulting in fast and efficient code execution.
Node.js takes advantage of V8's prowess, making it a favorite choice for building scalable and high-performance web applications.
Event-Driven Architecture
One of the key features of Node.js is its event-driven architecture, which is designed to handle asynchronous operations. This means that Node.js does not wait for an operation to complete before moving on to the next one. Instead, it registers callbacks and continues executing other code.
This non-blocking nature makes Node.js particularly well suited for handling large numbers of simultaneous connections, making it a popular choice for building web servers, APIs, and real-time applications.
The Node Package Manager (npm)
Node.js also introduced the Node Package Manager (npm), a package manager to manage dependencies and simplify the installation of JavaScript libraries and modules. The npm ecosystem is vast, with a wealth of community-contributed packages that developers can use to extend their applications and reduce the need to reinvent the wheel.
Use Cases
Node.js has found its sweet spot in various use cases, including:
- Web servers and RESTful APIs
- Real-time applications and chat applications
- Microservices architecture and serverless functions
- Web scraping and automation
- Command-line tools and utilities
The versatility of Node.js has made it a popular choice among developers, and its vibrant community ensures it remains an essential tool in the world of web development.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Full-stack Web Frameworks (Next.js) (psst, it's free!).
FAQ
What is Node.js?
Node.js is a JavaScript runtime environment built on the V8 JavaScript Engine that allows developers to run JavaScript code outside of a browser. It enables the creation of server-side applications and APIs using JavaScript.
What is the V8 JavaScript Engine?
The V8 JavaScript Engine is an open-source high-performance runtime engine developed by Google. It compiles JavaScript directly into machine code, resulting in fast and efficient code execution. Node.js uses the V8 engine to power its runtime environment.
What are some popular use cases for Node.js?
Some popular use cases for Node.js include building web servers and RESTful APIs, real-time applications and chat applications, microservices architecture and serverless functions, web scraping and automation, and command-line tools and utilities.
What is the Node Package Manager (npm)?
The Node Package Manager (npm) is a package manager for Node.js that manages dependencies and simplifies the installation of JavaScript libraries and modules. The npm ecosystem offers a wealth of community-contributed packages that developers can use to extend their applications.