Color Modes in p5.js
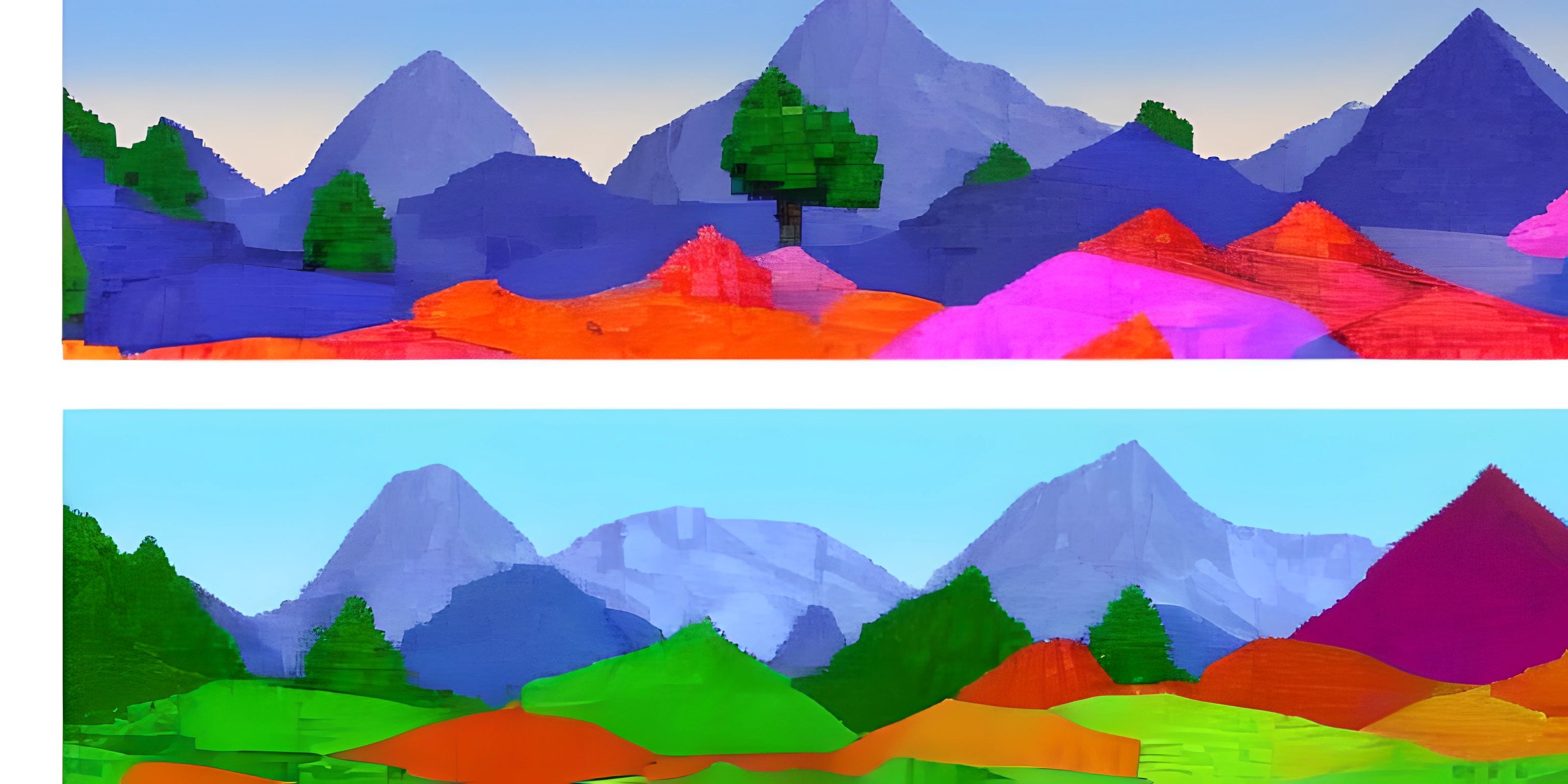
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
We've all seen what a splash of color can do to make our digital art and visual projects more vibrant and engaging. In p5.js, a JavaScript library designed to make coding accessible for artists, designers, and educators, we have the power to create colorful masterpieces with ease. One of the key aspects of working with color is understanding color modes. Buckle up as we dive into the world of color in p5.js!
What are Color Modes?
In p5.js, color modes determine how colors are defined and interpreted. By default, p5.js uses the RGB color mode (Red, Green, Blue), but it also supports two other modes: HSB (Hue, Saturation, Brightness) and HSL (Hue, Saturation, Lightness).
Each color mode has its own way of specifying colors, making it easier to work with colors in different contexts. Want to blend colors together like a painter? Use HSB mode. Need a specific shade of red? RGB mode has you covered.
RGB Color Mode
As mentioned, p5.js uses the RGB color mode by default. In this mode, colors are defined by three values representing the intensity of red, green, and blue components, respectively. The values range from 0 to 255, with 0 being the lowest intensity (black) and 255 being the highest intensity (full color).
Here's a simple example that sets the background color to red:
function setup() { createCanvas(400, 400); background(255, 0, 0); // Red background } function draw() { // Drawing code here }
Changing Color Modes
To switch between color modes, we use the colorMode()
function. This function takes one mandatory argument: the desired color mode (RGB
, HSB
, or HSL
). You can also provide optional arguments to set the maximum value for each component of the color mode.
Here's an example of switching to HSB mode and setting the background to a bright red:
function setup() { createCanvas(400, 400); colorMode(HSB, 360, 100, 100); // Switch to HSB mode background(0, 100, 100); // Bright red background } function draw() { // Drawing code here }
In this example, we set the maximum values for hue, saturation, and brightness to 360, 100, and 100, respectively. This allows us to work with percentages, making it easier to reason about colors in HSB mode.
HSB and HSL Color Modes
While RGB is all about the primary colors, HSB and HSL modes focus on hue, saturation, and either brightness or lightness.
- Hue: The base color (measured in degrees on a color wheel)
- Saturation: The intensity of the color (0% represents grayscale, 100% represents full color)
- Brightness (HSB): The amount of light in the color (0% is black, 100% is full color)
- Lightness (HSL): The amount of light in the color (0% is black, 50% is full color, 100% is white)
These two modes provide more intuitive ways to work with colors, especially when it comes to blending or creating color palettes.
Conclusion
Understanding color modes in p5.js is crucial for creating visually stunning projects. With RGB, HSB, and HSL modes at your disposal, you can now harness the power of color and transform your digital canvas into a mesmerizing work of art. Happy coloring!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Drawing Basic Shapes (psst, it's free!).
FAQ
What are the different color modes available in p5.js?
In p5.js, there are two primary color modes:
- RGB (Red, Green, Blue) - The default color mode, where colors are specified using values for red, green, and blue components.
- HSB (Hue, Saturation, Brightness) - An alternative color mode, where colors are specified using values for hue, saturation, and brightness.
How do I change the color mode in p5.js?
To change the color mode in p5.js, use the colorMode()
function. For example, to switch to HSB mode, you would use:
colorMode(HSB);
And to switch back to RGB mode, use:
colorMode(RGB);
Can I set a custom range for color values in p5.js?
Yes, you can set a custom range for color values in p5.js by providing additional arguments to the colorMode()
function. For example, if you want to use a 0-1 range for RGB values, you can do:
colorMode(RGB, 1, 1, 1);
Or, for HSB values in the range of 0-360 for hue and 0-100 for saturation and brightness, do:
colorMode(HSB, 360, 100, 100);
How do I create a color using the current color mode?
To create a color using the current color mode, use the color()
function. For example, in RGB mode, you can create a red color by specifying the red, green, and blue components like this:
let redColor = color(255, 0, 0);
Similarly, in HSB mode, you can create a color with specific hue, saturation, and brightness values like this:
let hsbColor = color(120, 50, 75);
Can I mix colors from different color modes?
Yes, you can mix colors from different color modes by creating colors using the color()
function and then blending them using the lerpColor()
function. For example, to blend a red color (RGB) with a green color (HSB), do:
colorMode(RGB); let redColor = color(255, 0, 0); colorMode(HSB, 360, 100, 100); let greenColor = color(120, 50, 75); let blendedColor = lerpColor(redColor, greenColor, 0.5);