Pointers Explained
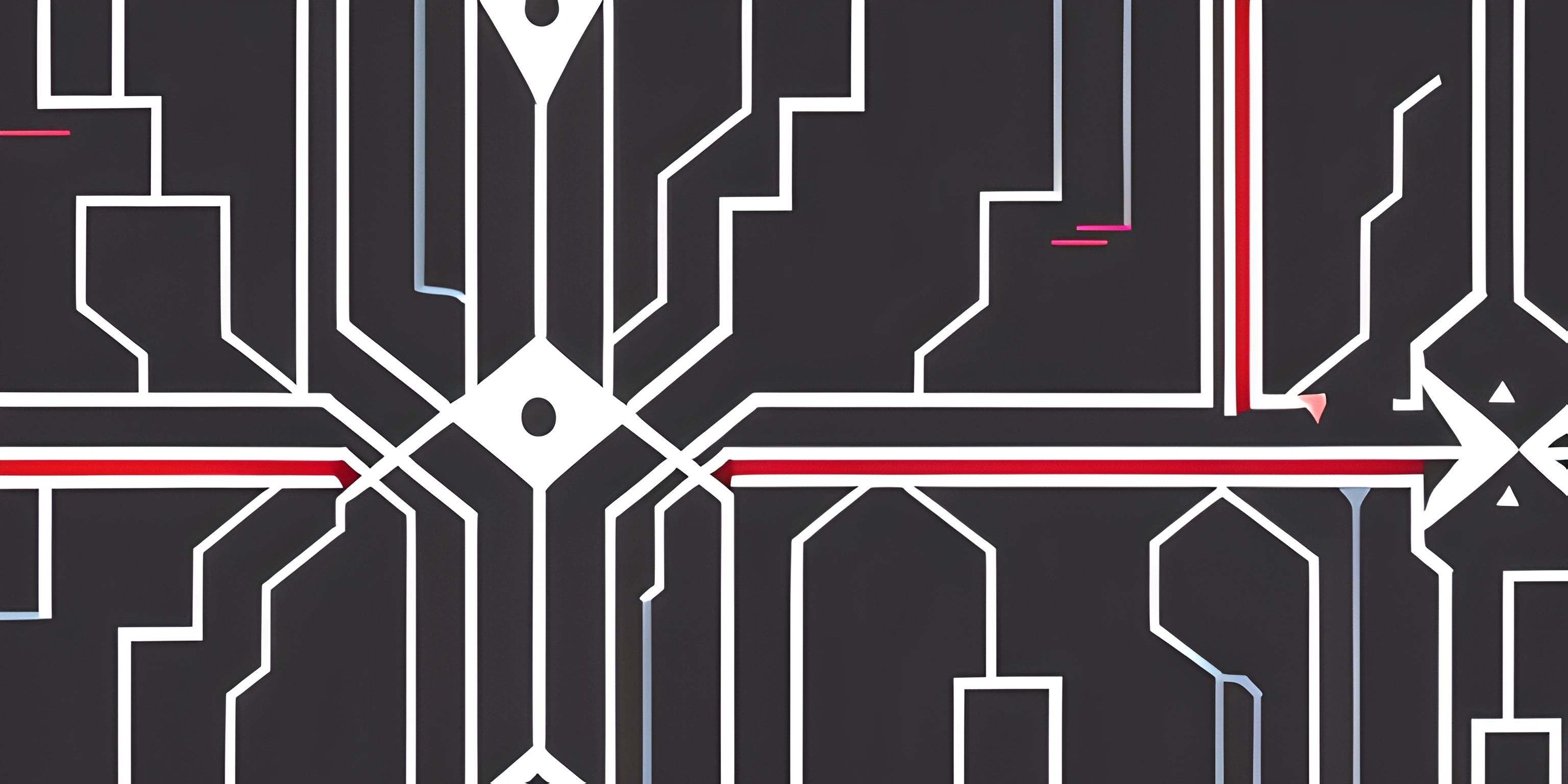
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Ever played a treasure hunt game where you got a clue leading to another clue, and so on, until you finally found the treasure? In programming, pointers are like those clues – they hold the memory address of another value, leading you right to the "treasure" (the actual data). Let's dive in and explore the world of pointers.
What are Pointers?
A pointer is a variable that stores the memory address of another variable or value. Instead of holding the actual data, it points to the location where the data is stored. This can be quite useful in certain scenarios, such as when working with large data structures or when you want to share data across different parts of your program.
Pointers are commonly used in languages like C and C++, where memory management is crucial to optimize performance.
Declaring Pointers
To declare a pointer, you need to specify the type of data it points to, followed by an asterisk (*) and the pointer name. Here's an example in C:
int *p; // Declare a pointer to an integer
This declares a pointer named p
that can store the address of an integer variable.
Initializing Pointers
Before you can use a pointer, it must be initialized with the address of a variable. To get the address of a variable, you use the "address-of" operator &
. Here's an example:
int num = 42; int *p; // Declare a pointer to an integer p = # // Initialize the pointer with the address of 'num'
Now, our pointer p
holds the memory address of the variable num
.
Accessing Data through Pointers
To access the data stored at the memory address a pointer points to, you use the "dereference" operator *
. This is also known as "indirection." Here's an example:
int num = 42; int *p = # // Declare and initialize a pointer to 'num' int data = *p; // Dereference the pointer to get the value stored at 'num'
In this example, data
will be assigned the value 42
, which is the value of the variable num
.
Why use Pointers?
Pointers offer several advantages in programming:
- Efficient memory usage: Pointers can be used to work with large data structures without copying the actual data, saving memory and improving performance.
- Dynamic memory allocation: Pointers allow you to allocate and deallocate memory at runtime, enabling the creation of dynamic data structures like linked lists and trees.
- Function arguments: Pointers can be used to pass data to functions by reference, allowing the function to modify the original data and not just a copy.
As you progress in your programming journey, you'll come to appreciate the power and flexibility that pointers provide. Just remember to handle them with care, or you might end up with a wild goose chase instead of a successful treasure hunt!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust - A Language You'll Love (psst, it's free!).
FAQ
What are pointers, and why are they important in programming?
Pointers are a programming concept that allows you to directly access and manipulate memory locations by storing the memory addresses of data objects. They are important in programming because they enable more efficient memory management, allow for dynamic data structures, and provide a way to pass large data objects to functions without making a copy.
How do you declare and initialize a pointer in C or C++?
To declare and initialize a pointer in C or C++, you need to specify its data type, followed by an asterisk (*), and then provide a variable name. To initialize a pointer, assign it the address of a variable using the '&' (address-of) operator. Here's an example in C++:
int main() { int num = 42; int* pNum; // Declare a pointer to an int pNum = # // Initialize the pointer with the address of 'num' }
How can I access and manipulate data using pointers?
You can access and manipulate data using pointers by dereferencing them. In C and C++, you use the asterisk (*) operator to dereference a pointer and access the data it points to. To modify the data, assign a new value to the dereferenced pointer. Here's an example:
int main() { int num = 42; int* pNum = # // Pointer pointing to 'num' // Access data using the pointer cout << "Value pointed by pNum: " << *pNum << endl; // Modify data using the pointer *pNum = 100; cout << "Value of num after modification: " << num << endl; }
What are some common pointer-related errors to watch out for?
Some common pointer-related errors to watch out for include:
- Uninitialized pointers: Using a pointer without initializing it can lead to undefined behavior and crashes.
- Null pointer dereferencing: Accessing or manipulating data through a null pointer can cause a crash.
- Dangling pointers: Pointers that still point to memory that has been freed or deallocated can cause undefined behavior if accessed.
- Memory leaks: Failing to properly manage dynamic memory allocation and deallocation can lead to memory leaks and eventually run out of memory.
Can you give an example of using pointers with dynamic memory allocation in C++?
Sure! In C++, you can use the new
operator to allocate memory dynamically, and the delete
operator to deallocate the memory when it's no longer needed. Here's an example using pointers with dynamic memory allocation:
int main() { int* pArray = new int[5]; // Allocate memory for an array of 5 integers // Assign values to the dynamically allocated array for (int i = 0; i < 5; i++) { pArray[i] = i * 10; } // Access and print the values for (int i = 0; i < 5; i++) { cout << "Value at index " << i << ": " << pArray[i] << endl; } delete[] pArray; // Deallocate memory when finished }