Pointers in Programming
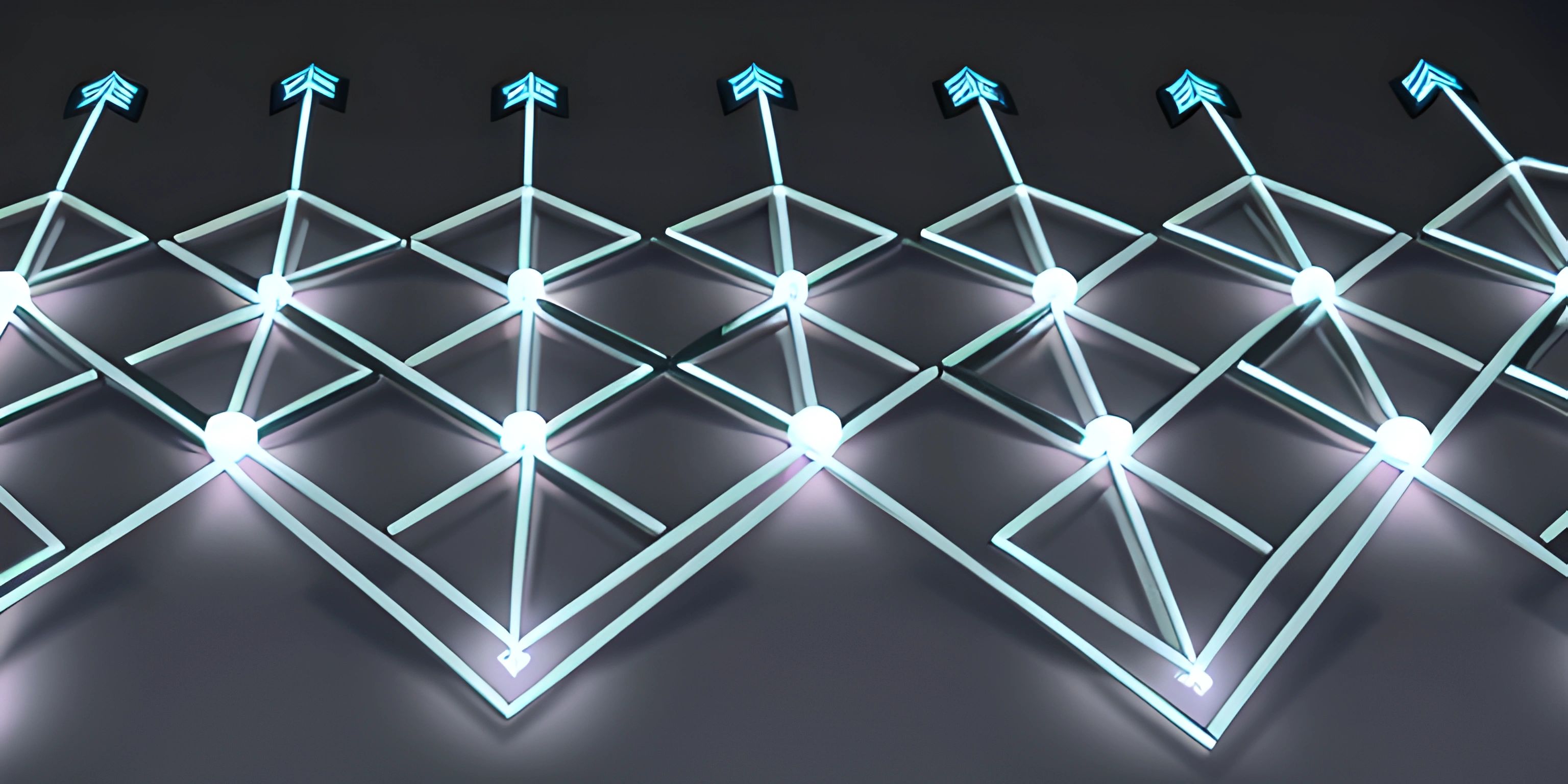
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
If you've ever gone treasure hunting, you know that a good map can be the difference between finding buried riches and digging up empty holes. In programming, the treasure is data, and pointers are the maps that help you find it. Pointers are variables that store the memory address of another variable. By using pointers, we can directly access and manipulate data in memory, leading to more efficient and flexible code.
How Pointers Work
When we create a variable in a program, it's stored in a specific location in memory. This location is represented by an address, just like the address of a house. A pointer, then, is a special variable that holds the address of another variable. Instead of storing the actual data, a pointer stores the map on how to find the data.
Let's take a look at an example using pseudocode:
int number = 42; int* pointerToNumber = &number;
Here, we first create an int
variable called number
and assign it the value 42
. Next, we create a pointer called pointerToNumber
that will store the address of number
. The &
symbol is used to get the address of number
.
Why Use Pointers?
You might be wondering, "Why not just use the variable directly?" While it's true that you can often work with variables directly, pointers offer certain advantages:
- Memory efficiency: Pointers allow us to work with large amounts of data without copying the data itself. Instead, we just pass around the address, which is typically much smaller.
- Dynamic memory allocation: Pointers enable us to allocate and deallocate memory during runtime, allowing for more flexible and efficient use of resources.
- Indirect access: Pointers can be used to access variables indirectly, which is useful when working with data structures like linked lists and trees.
Common Pointer Operations
There are a few common operations when working with pointers:
-
Dereferencing: When you want to access the data stored at the memory address a pointer is pointing to, you use the
*
symbol to "dereference" the pointer. Here's an example:int number = 42; int* pointerToNumber = &number; int data = *pointerToNumber; // data now holds the value 42
-
Pointer arithmetic: You can perform arithmetic operations on pointers, allowing you to move through memory and access different elements in an array, for example. Keep in mind that pointer arithmetic is dependent on the type of data being pointed to. The size of the data type affects how the pointer moves through memory.
int numbers[] = {10, 20, 30, 40}; int* pointerToNumbers = numbers; // points to the first element (10) pointerToNumbers++; // points to the second element (20)
-
Null pointers: A null pointer is a pointer that doesn't point to any valid memory address. It's a good practice to initialize pointers to
null
if you don't know what they'll point to yet. You can check if a pointer is null before using it to avoid errors.int* pointer = null; if (pointer != null) { int data = *pointer; // This won't happen because pointer is null }
Conclusion
Pointers, while sometimes intimidating, are an essential concept in programming that provide many benefits. They allow for more efficient use of memory, dynamic resource management, and indirect access to data. With a good grasp of pointers, you'll be well on your way to uncovering the treasures that lie within your code. Now, grab your map and get ready to explore the world of pointers!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: What Programming Means (psst, it's free!).
FAQ
What is a pointer in programming?
A pointer is a variable that stores the memory address of another variable or object. In programming languages like C and C++, pointers are often used to provide efficient ways of accessing and manipulating data in memory. They allow us to pass large data structures by reference, rather than by value, which can be more efficient in terms of memory usage and performance.
How do I declare and use a pointer?
To declare a pointer, you need to specify the data type it will point to, followed by an asterisk (*) before the pointer's name. Here's an example in C:
int *ptr; // Declares an integer pointer named ptr
To use the pointer, you'll need to assign it the address of a variable using the address-of operator (&):
int num = 42; ptr = # // Assigns the address of num to ptr
Now, you can access and manipulate the variable through the pointer using the dereference operator (*):
*ptr = 24; // Changes the value of num to 24
What are some common uses of pointers in programming?
Pointers are commonly used for the following purposes:
- Dynamic memory allocation: Pointers allow us to allocate and deallocate memory during runtime, which enables the creation of dynamic data structures like linked lists, trees, and graphs.
- Passing by reference: Pointers enable us to pass large data structures to functions by reference, rather than by value, which can be more efficient in terms of memory usage and performance.
- Arrays and strings: Pointers can be used to access and manipulate elements of arrays and strings, as they can point to specific elements and allow for pointer arithmetic.
- Implementing advanced data structures: Pointers are essential for implementing advanced data structures like linked lists, trees, and graphs, which rely on nodes that reference one another.
How do I use pointers with functions?
When using pointers with functions, you can pass a pointer as an argument, which allows the function to directly modify the variable it points to. Here's an example in C:
void doubleValue(int *value) { *value *= 2; } int main() { int num = 10; doubleValue(&num); printf("Doubled value: %d\n", num); // Output: Doubled value: 20 return 0; }
In this example, the doubleValue
function takes a pointer to an integer as its parameter. When we call the function with the address of num
, it directly modifies its value, doubling it.
What are some potential issues when working with pointers?
Pointers, if not used with care, can lead to several issues, such as:
- Dangling pointers: A dangling pointer is a pointer that points to memory that has been freed or deallocated. Accessing or modifying memory through a dangling pointer can cause undefined behavior and lead to crashes or data corruption.
- Memory leaks: Failure to properly release memory that was dynamically allocated using pointers can lead to memory leaks, which consume system resources and can cause the program to crash or slow down over time.
- Null pointer dereferencing: Attempting to dereference a null pointer (a pointer that doesn't point to any valid memory address) can cause a runtime error or crash.
- Buffer overflows: Accessing memory beyond the boundaries of an array or buffer using pointer arithmetic can lead to buffer overflows, which can cause crashes or security vulnerabilities.