Understanding Null Pointer Dereferences and How to Avoid Them
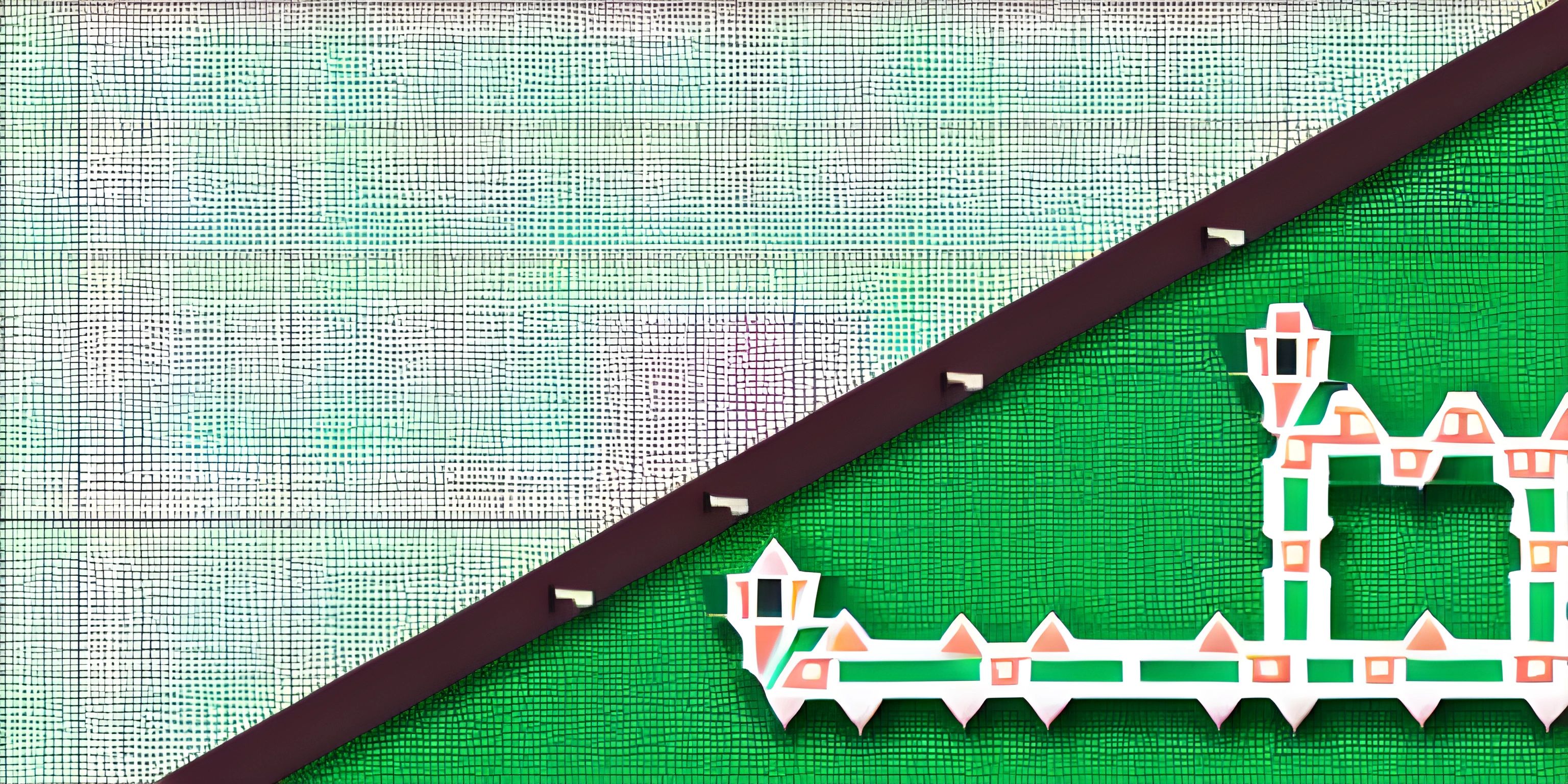
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
In the wonderful world of programming, nothing inspires more dread than the phrase "null pointer dereference." It sounds like something out of a sci-fi horror movie, but it's actually a common programming bug that can cause epic meltdowns. In this article, we'll dive deep into what null pointer dereferences are, why they occur, and how to avoid them. Buckle up, because we're about to embark on a journey through the memory lanes of your code!
What is a Null Pointer Dereference?
Picture this: You have a pointer, which is like a treasure map leading to a memory location where your valuable data is stored. Now imagine if that map pointed to nowhere! That’s what a null pointer is—a pointer that doesn’t point to any valid memory location. When your code tries to dereference this null pointer (i.e., follow the treasure map to retrieve the data), it’s like trying to find treasure in a place that doesn’t exist. The result? A program crash or, even worse, undefined behavior.
int *ptr = NULL; // ptr is a null pointer int value = *ptr; // Dereferencing null pointer
In the above example, ptr
is a null pointer. When we try to dereference it to get the value it points to, the program crashes. It's like trying to read a book that isn’t there—your program just won't know what to do.
Why Do Null Pointer Dereferences Occur?
Null pointer dereferences can occur for several reasons. Here are a few common ones:
- Uninitialized Pointers: Forgetting to initialize a pointer before using it.
- Dangling Pointers: Pointers that used to point to valid memory but the memory has been deallocated.
- Incorrect Assumptions: Assuming a pointer will never be null without checking.
Let's explore these scenarios with some examples.
Uninitialized Pointers
Imagine you’re a detective, and you’ve been given a case file without any information in it. Trying to solve the case would be impossible! Similarly, if you try to use a pointer without initializing it, you’re asking for trouble.
int *ptr; // Uninitialized pointer *ptr = 42; // Dereferencing uninitialized pointer
In the above code, ptr
is never assigned a valid memory address before it's used. It's like trying to solve a case with an empty file—it just doesn’t work.
Dangling Pointers
Dangling pointers occur when the memory a pointer points to is deallocated, but the pointer still holds the address of that memory. It’s like having an old address for a friend who moved away; showing up at their former house won’t get you anywhere.
int *ptr = (int *)malloc(sizeof(int)); free(ptr); // Deallocating memory *ptr = 42; // Dereferencing dangling pointer
Here, ptr
becomes a dangling pointer after the free
function is called. Trying to use it afterward is like knocking on a door that no longer exists.
Incorrect Assumptions
Sometimes, we make assumptions that certain pointers will never be null. This overconfidence can land us in hot water!
void printValue(int *ptr) { printf("%d\n", *ptr); // Assuming ptr is never null }
In the printValue
function, we're assuming ptr
will always be valid. If ptr
is null, we’re in for a nasty surprise when we try to dereference it.
How to Avoid Null Pointer Dereferences
Avoiding null pointer dereferences requires a combination of good practices and vigilant coding. Here are some strategies to keep your code safe:
Initialize Your Pointers
Always initialize your pointers before using them. This is like making sure your detective case file has the necessary information before you begin your investigation.
int value = 42; int *ptr = &value; // Initialize pointer printf("%d\n", *ptr);
Check for Null
Before using a pointer, always check if it’s null. This is like calling your friend to make sure they're home before you visit.
if (ptr != NULL) { printf("%d\n", *ptr); } else { printf("Pointer is null\n"); }
Use Smart Pointers (in C++)
In C++, smart pointers like std::unique_ptr
and std::shared_ptr
help manage the lifetime of objects automatically, reducing the risk of dangling pointers.
#include <memory> std::unique_ptr<int> ptr = std::make_unique<int>(42); printf("%d\n", *ptr);
Defensive Programming
Adopt defensive programming techniques by validating inputs and assumptions throughout your code. This is like double-checking your work to ensure everything is in order.
void safePrintValue(int *ptr) { if (ptr != NULL) { printf("%d\n", *ptr); } else { printf("Pointer is null\n"); } }
Use Static Analysis Tools
Static analysis tools can automatically detect potential null pointer dereferences in your code. These tools act like automated code detectives, patrolling your codebase for bugs.
Conclusion
Null pointer dereferences are like hidden traps in your code. They can cause crashes and undefined behavior, making them a major headache for developers. By understanding why they occur and following best practices to avoid them, you can keep your code safe and sound. Remember to initialize your pointers, check for null, use smart pointers in C++, adopt defensive programming techniques, and leverage static analysis tools.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust - A Language You'll Love (psst, it's free!).
FAQ
What is a null pointer dereference?
A null pointer dereference occurs when a program tries to access or modify data through a pointer that is null, meaning it does not point to any valid memory location. This often leads to crashes or undefined behavior.
Why do null pointer dereferences occur?
Null pointer dereferences can occur due to uninitialized pointers, dangling pointers (pointers that reference deallocated memory), or incorrect assumptions that a pointer will never be null without proper checks.
How can I avoid null pointer dereferences?
You can avoid null pointer dereferences by always initializing your pointers, checking if pointers are null before using them, using smart pointers in C++, adopting defensive programming techniques, and using static analysis tools to detect potential issues.
What are smart pointers and how do they help?
Smart pointers in C++ (such as std::unique_ptr
and std::shared_ptr
) automatically manage the lifetime of objects, ensuring that memory is properly allocated and deallocated. This reduces the risk of dangling pointers and memory leaks.
Are there any tools for detecting null pointer dereferences?
Yes, static analysis tools like Clang Static Analyzer, Coverity, and PVS-Studio can automatically detect potential null pointer dereferences in your code, helping you identify and fix issues before they cause problems.