Understanding Null Pointers
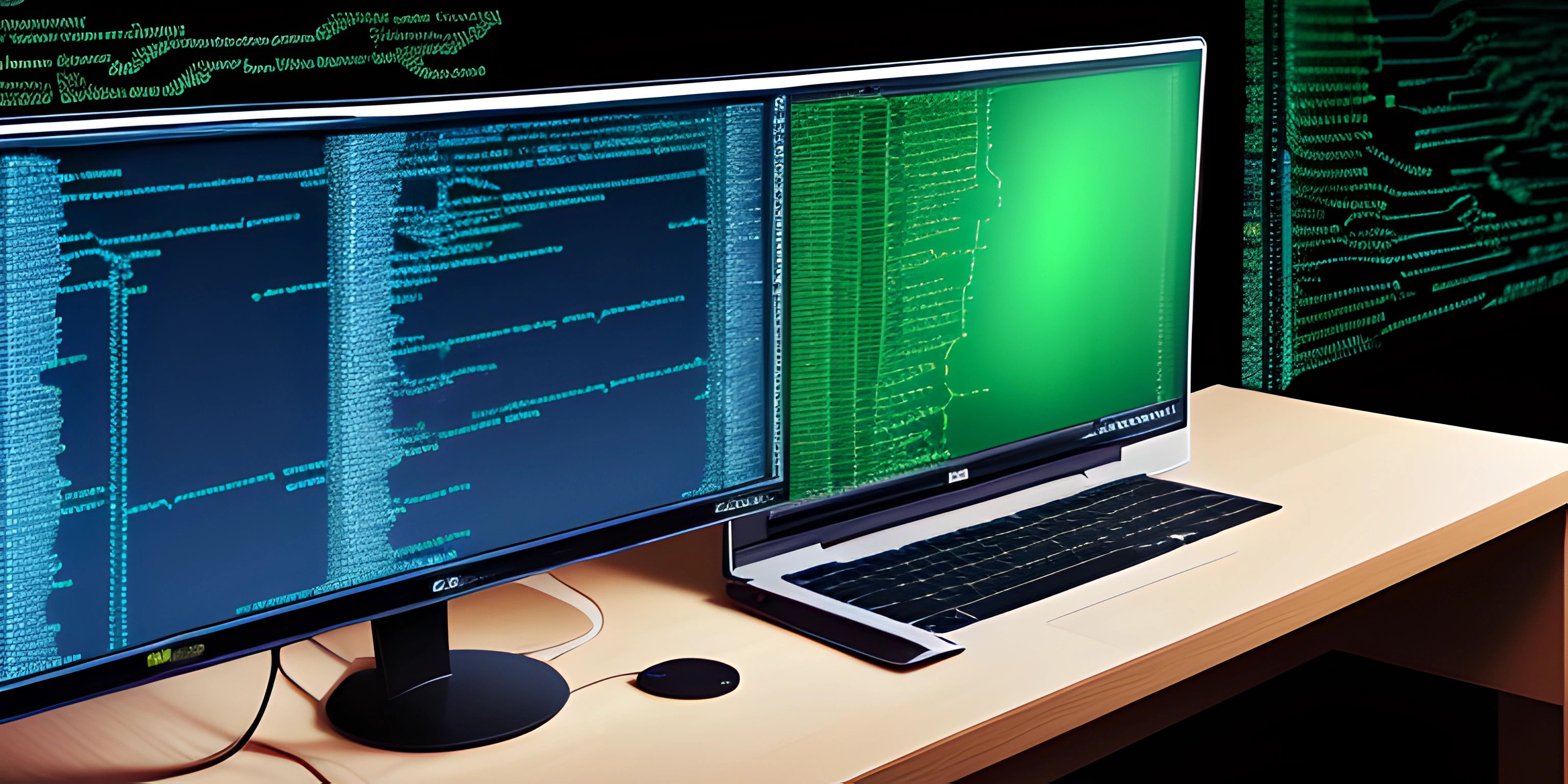
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Null pointers, or null references, are quite infamous in the programming world. They're like the banana peels of coding – you never want to slip up on them. So, let's take a closer look at what null pointers are and how you can avoid falling into their traps.
What is a Null Pointer?
A null pointer is a reference that doesn't point to any valid memory location. In other words, it's like having a treasure map that leads to nowhere or trying to call a friend who changed their phone number. When you attempt to access the memory location pointed to by a null pointer, you're bound to run into trouble, such as a crash or an error.
In many programming languages like C++, Java, and C#, null pointers are commonly represented by the keyword null
or a similar variant.
The Dangers of Null Pointers
Null pointers can cause some serious issues in your code:
- Crashes: When your program tries to access memory through a null pointer, it's likely to crash, causing the dreaded "Segmentation fault" in languages like C++.
- Undefined behavior: Languages like C++ leave the behavior of null pointer dereferences as "undefined," meaning that anything could happen – it's like a coding Pandora's box.
- Exceptions: In languages like Java and C#, attempting to access memory via a null pointer results in a
NullPointerException
orNullReferenceException
, respectively.
Regardless of the language, null pointers can bring chaos to your code, and it's crucial to handle them properly.
How to Avoid Null Pointer Issues
To dodge null pointer pitfalls, follow these tips:
-
Initialize your pointers: When you declare a pointer, always give it an initial value, either by pointing it to a valid memory location or explicitly setting it to
null
.int *ptr = nullptr; // C++ example
-
Check for null before dereferencing: Before accessing memory through a pointer, make sure it's not null.
if (myObject != null) { // Java example myObject.doSomething(); }
-
Use smart pointers or references: In languages like C++, opt for smart pointers or references when appropriate, as they help manage memory and prevent null pointer issues.
-
Leverage optional types: Some languages, like Swift and Kotlin, provide optional types that can help you safely work with nullable values.
By keeping these tips in mind and staying vigilant, you'll be well-prepared to avoid the perils of null pointers, making your code more robust and reliable.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust - A Language You'll Love (psst, it's free!).
FAQ
What is a null pointer?
A null pointer is a special type of pointer that points to nothing or has no address associated with it. It's represented by the keyword NULL
in most programming languages. It can be used to indicate that a pointer has not been assigned a valid memory address, or that it should not point to any valid memory location.
Why can null pointers be dangerous?
Null pointers can be dangerous because they can lead to unexpected behavior and crashes in a program if they are accidentally dereferenced (i.e., if you try to access the memory they point to). Since a null pointer doesn't point to any valid memory location, attempting to read or write at that location can result in undefined behavior and lead to segmentation faults, memory corruption, or other critical problems.
How can I avoid null pointer issues in my code?
To avoid null pointer issues in your code, follow these best practices:
- Always initialize your pointers, either by assigning them a valid memory address or explicitly setting them to
NULL
. - Before dereferencing a pointer, always check if it's not
NULL
to ensure that it points to a valid memory location. - Be cautious when using pointer arithmetic, as it can easily lead to accessing memory locations beyond the intended range.
- When deallocating memory, set the pointer to
NULL
to ensure that it doesn't point to the deallocated memory anymore, preventing accidental usage.
What are some common causes of null pointer dereferences?
Common causes of null pointer dereferences include:
- Forgetting to initialize a pointer before using it.
- Improperly checking whether a pointer is
NULL
before dereferencing it. - Accidentally setting a pointer to
NULL
instead of its intended memory address. - Returning a pointer to a local variable or temporary object that goes out of scope, resulting in a dangling pointer.
Are there any tools to help detect null pointer dereferences?
Yes, there are several tools available to help detect null pointer dereferences and other programming errors. Some popular options include static code analyzers like Clang's Static Analyzer, Coverity Scan, and PVS-Studio, as well as dynamic analysis tools like Valgrind and AddressSanitizer. These tools can help identify potential issues in your code early on, allowing you to fix them before they cause problems at runtime.