Heap vs Stack: Memory in Programming
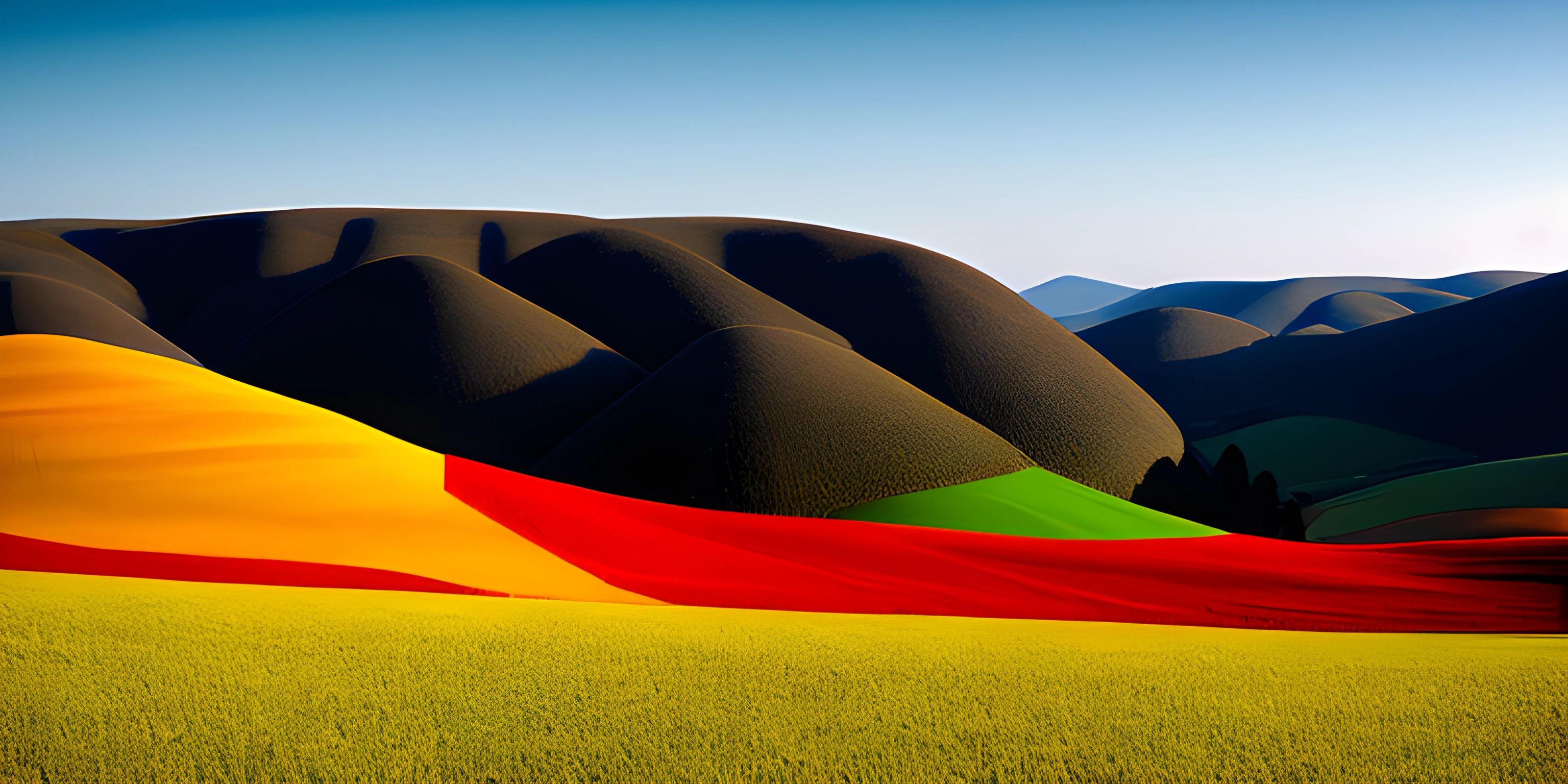
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Understanding how memory works is like knowing the backstage of a theater. It's not just about learning the lines of the play (the code), but also understanding how the stage is set and organized. In programming, memory management is critical and is primarily divided into two key areas: the stack and the heap. Both serve different functions and have their own advantages and disadvantages.
Stack Memory
First, let's explore the stack. Stack memory is like the Star of the evening, always in the limelight. It is mainly used for storing temporary variables created by each function. These variables are automatically allocated and deallocated.
In terms of structure, the stack is like a stack of pancakes. The last pancake (or the most recent function) to be added is the first one to be removed. This characteristic is called Last In, First Out (LIFO). Here's a simple example using pseudocode to illustrate this:
function pancakes() { var pancake1 = "Yummy"; var pancake2 = "Delicious"; }
In this case, pancake2 will be removed from the stack before pancake1, following the LIFO rule. It's simple and efficient, but the size of the stack is limited. It's like a plate of pancakes, you can't add more than it can hold!
Heap Memory
Next, let's dive into the heap. Heap memory is like the unsung hero, always working in the background. Unlike stack memory, heap memory is used for dynamic memory allocation, and has no automatic management mechanism.
Think of the heap as a vast farmland where you can plant anything, anywhere. Unlike the orderly stack, heap memory doesn't follow a pattern. You can allocate and deallocate memory anywhere in the heap.
But with great power comes great responsibility! Because the heap has no automatic management, it's up to the programmer to make sure memory is properly managed to avoid memory leaks and other issues.
function plantSeeds() { var seed1 = new Seed(); // Planting more seeds... delete seed1; // Remember to clean up! }
In this example, we have to manually delete seed1 when we're done. It's a bit more work, but the heap offers flexibility and ample space.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust - A Language You'll Love (psst, it's free!).
FAQ
What is stack memory in programming?
Stack memory in programming is a type of memory that stores temporary variables created by each function. It follows a Last In, First Out (LIFO) structure, meaning the most recently added items are removed first. It's known for its efficiency but has limited size.
What is heap memory in programming?
Heap memory in programming is used for dynamic memory allocation and doesn't have an automatic management mechanism like the stack. It offers more flexibility and space, but it's up to the programmer to manage the memory properly to avoid issues such as memory leaks.
How do stack and heap memory differ?
Stack and heap memory serve different purposes and have different characteristics. Stack memory is mainly used for storing temporary variables and has automatic management, but it's limited in size. On the other hand, heap memory is used for dynamic memory allocation, offers more space and flexibility, but requires manual memory management.