Python Data Types
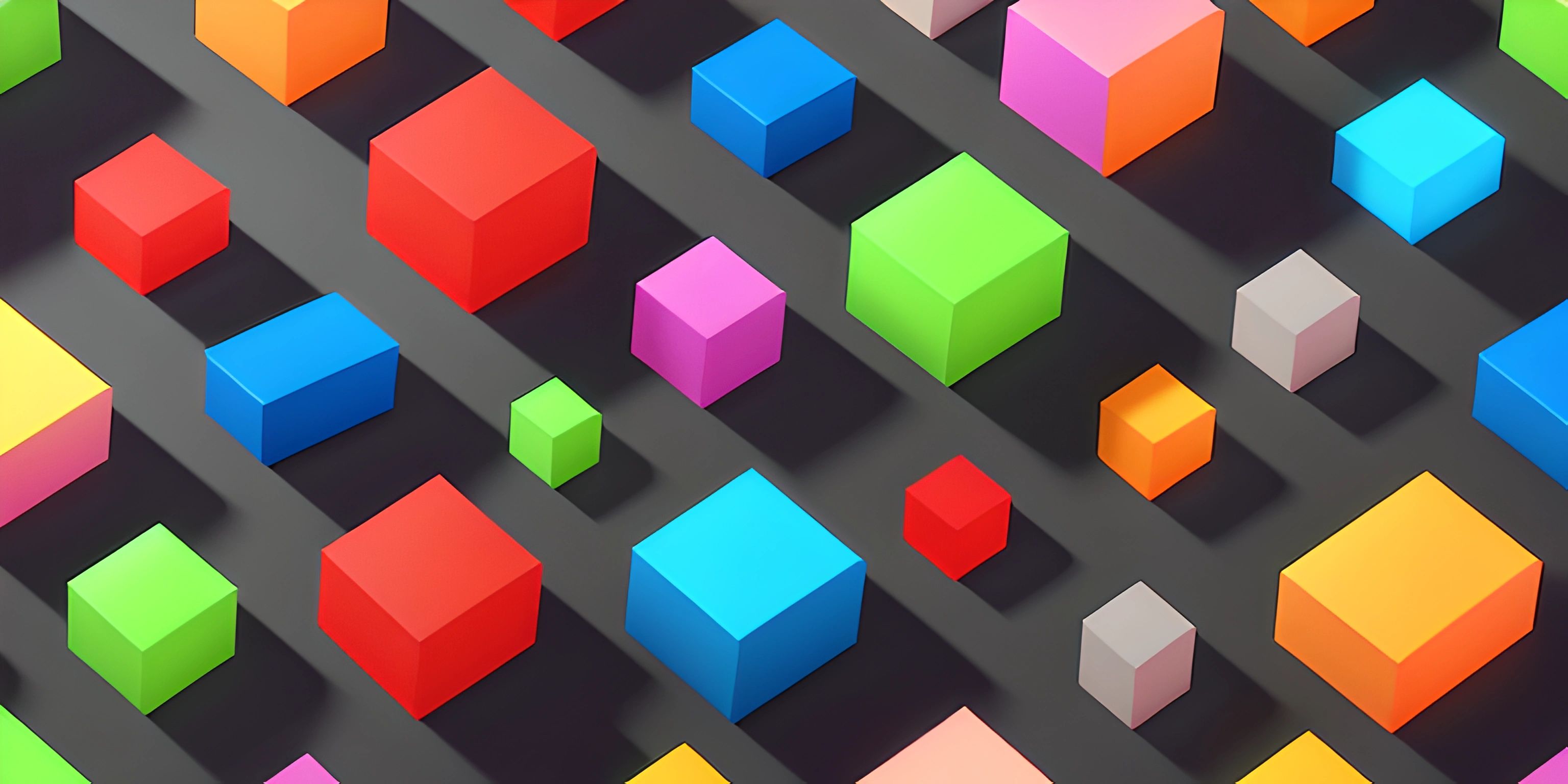
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Python is a versatile language that supports a variety of data types to help you manage and manipulate information in your programs. From simple integers to complex data structures like dictionaries and sets, Python has got you covered. Let's explore the different data types available in Python and how to use them effectively.
Basic Data Types
Integers
Integers, or int
, are whole numbers, both positive and negative. Python supports arbitrarily large integers, so you can have an integer as big as you can fit in your computer's memory. Here's an example of declaring and using integers in Python:
age = 25 days_in_year = 365 days_alive = age * days_in_year print(days_alive) # Output: 9125
Floats
Floating-point numbers, or float
, represent real numbers with decimal points. Python's float values are approximately 64-bit double-precision numbers. Here's an example of declaring and using floats in Python:
pi = 3.14159 radius = 5.0 circle_area = pi * (radius ** 2) print(circle_area) # Output: 78.53975
Strings
Strings, or str
, are sequences of characters. They can be created using single quotes ('
), double quotes ("
), or even triple quotes ('''
or """
) for multiline strings. Here's an example of declaring and using strings in Python:
greeting = "Hello, World!" name = 'Alice' multiline_string = """This is a multiline string.""" print(greeting) # Output: Hello, World!
Booleans
Booleans, or bool
, represent True or False values. They are often used in conditional statements and comparisons. Here's an example of declaring and using booleans in Python:
is_hungry = True has_food = False should_eat = is_hungry and not has_food print(should_eat) # Output: False
Data Structures
Lists
Lists, or list
, are ordered, mutable collections of items. They are similar to arrays in other languages, but can store items of different data types. Here's an example of declaring and using lists in Python:
fruits = ["apple", "banana", "cherry"] fruits.append("orange") print(fruits) # Output: ['apple', 'banana', 'cherry', 'orange']
Tuples
Tuples, or tuple
, are ordered, immutable collections of items. They are similar to lists, but cannot be changed once they are created. Here's an example of declaring and using tuples in Python:
coordinates = (10, 20, 30) x, y, z = coordinates print(x) # Output: 10
Sets
Sets, or set
, are unordered collections of unique items. They are useful for removing duplicates or performing set operations like union and intersection. Here's an example of declaring and using sets in Python:
fruits = {"apple", "banana", "cherry"} more_fruits = {"banana", "orange", "kiwi"} unique_fruits = fruits.union(more_fruits) print(unique_fruits) # Output: {'apple', 'banana', 'cherry', 'orange', 'kiwi'}
Dictionaries
Dictionaries, or dict
, are unordered collections of key-value pairs. They are similar to hash tables or associative arrays in other languages. Here's an example of declaring and using dictionaries in Python:
person = {"name": "Alice", "age": 30, "city": "New York"} person["age"] = 31 print(person) # Output: {'name': 'Alice', 'age': 31, 'city': 'New York'}
By understanding these data types and their usage, you'll be well-equipped to handle a wide variety of programming tasks in Python. Don't be afraid to mix and match them to create complex data structures that perfectly suit your needs. Happy coding!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Syntax (psst, it's free!).
FAQ
What are the basic data types in Python?
Python has several built-in data types, including:
- int (integer): Whole numbers, such as 1, 42, or -7
- float (floating-point): Decimal numbers, such as 3.14 or -0.001
- str (string): Text enclosed in quotes, such as "Hello, World!"
- bool (boolean): Logical True or False values
- list: Ordered, mutable collection of items, such as [1, 2, 3]
- tuple: Ordered, immutable collection of items, such as (1, 2, 3)
- dict (dictionary): Key-value pairs, such as {"key": "value"}
- set: Unordered, unique collection of items, such as {1, 2, 3}
How do I determine the data type of a variable in Python?
You can use the type()
function to determine the data type of a variable. For example:
x = 42 print(type(x)) # Output: <class 'int'>
Can I change the data type of a variable in Python?
Yes, you can convert a variable from one data type to another using built-in functions like int()
, float()
, str()
, bool()
, list()
, tuple()
, dict()
, and set()
. For example:
x = 3.14 y = int(x) # Convert float to int, y becomes 3
How do I create a list, tuple, dictionary, or set in Python?
You can create these data types using the following syntax:
# List my_list = [1, 2, 3] # Tuple my_tuple = (1, 2, 3) # Dictionary my_dict = {"key1": "value1", "key2": "value2"} # Set my_set = {1, 2, 3}
Are Python strings mutable or immutable?
Python strings are immutable, meaning their characters cannot be changed after they are created. However, you can create a new string by concatenating, slicing, or using other string methods.