React Testing
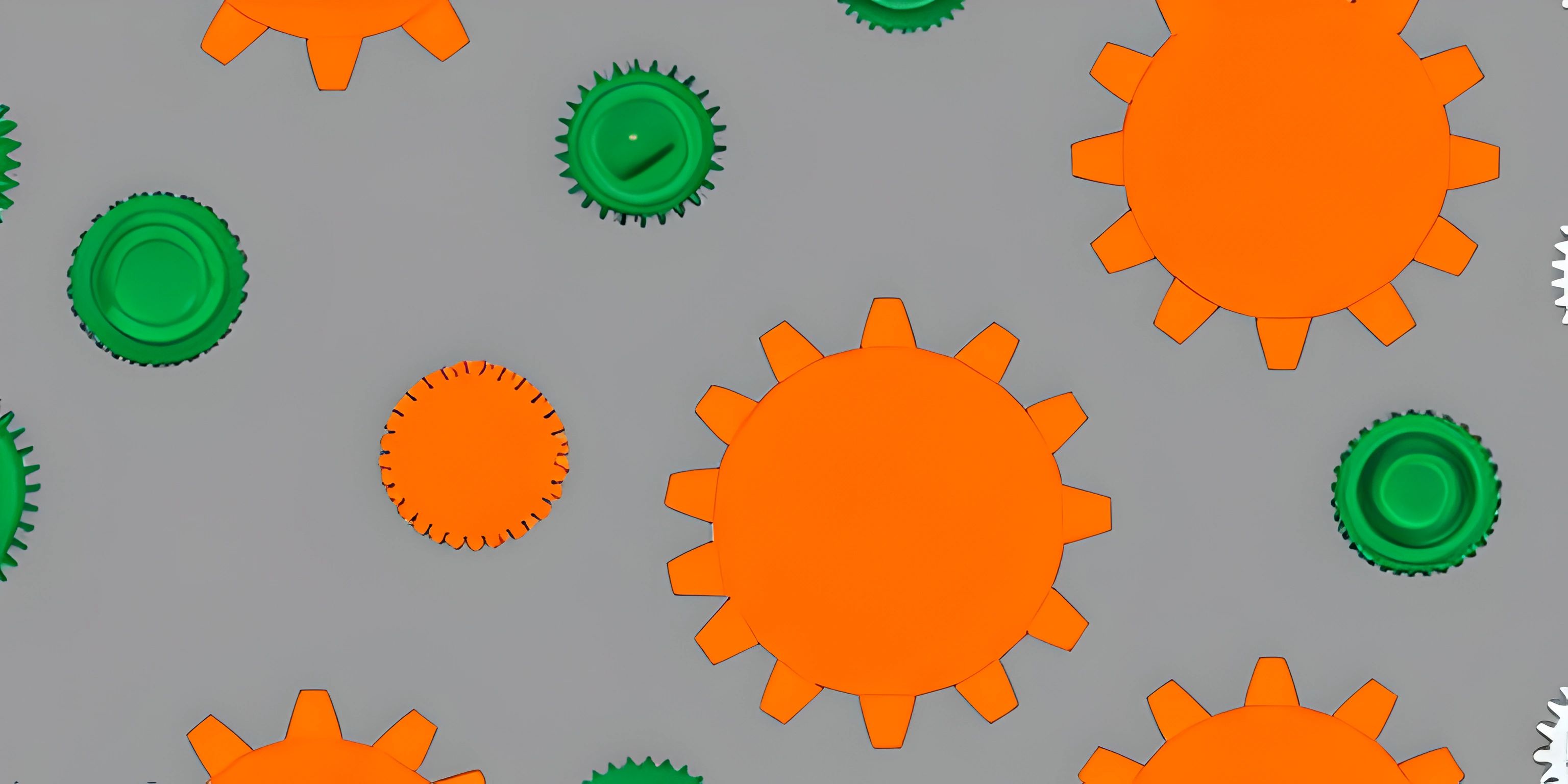
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
React is a powerful library for crafting user interfaces, but what if you want to ensure your components and applications are up to snuff? That's where testing comes in. By testing your React components, you can catch bugs early, maintain high code quality, and sleep soundly at night.
Testing Tools
There are several testing tools available for React applications. Here are a few popular ones:
Jest
Jest is a popular testing framework built by Facebook, the same team behind React. It's designed specifically for JavaScript and works seamlessly with React. Jest provides a variety of features such as snapshot testing, asynchronous testing, and mocking.
Enzyme
Enzyme is a testing library created by Airbnb. It's designed to simplify the testing of React components by providing utility functions to simulate user interactions, traverse the DOM, and manipulate component state. Enzyme can be used in conjunction with Jest or other testing frameworks.
React Testing Library
React Testing Library is a lightweight testing library focused on testing components as a user would interact with them. It encourages best practices by discouraging shallow rendering and testing implementation details. React Testing Library is often used with Jest as the testing framework.
Testing Strategies
When testing React components, it's important to select the right testing strategy for your needs. Here are some common strategies:
Unit Testing
Unit testing is the process of testing individual components in isolation. This ensures that each component works as expected without any external dependencies. Unit testing is a great way to catch bugs early and ensure that your components are reliable building blocks for your application.
Integration Testing
Integration testing goes a step further than unit testing by testing how multiple components work together. This helps to ensure that the communication between components is correct and that they function properly as a whole.
End-to-End Testing
End-to-end testing simulates complete user flows within your application. This can help you catch issues that might not be apparent during unit or integration testing, such as broken navigation or incorrect data handling.
Testing React Components
Let's dive into testing React components with Jest and React Testing Library. First, ensure you have both libraries installed in your project:
npm install --save-dev jest @testing-library/react
Next, create a test file with the same name as your component, but with a .test.js
extension. For example, if your component is named MyComponent.js
, your test file should be called MyComponent.test.js
.
In your test file, import the required libraries, your component, and write a simple test:
import React from "react"; import { render, screen } from "@testing-library/react"; import MyComponent from "./MyComponent"; test("renders my component", () => { render(<MyComponent />); const textElement = screen.getByText(/my component/i); expect(textElement).toBeInTheDocument(); });
This test checks if the text "my component" is present within the rendered component. If the test passes, you can be confident that your component renders correctly.
Remember that testing is an essential part of building reliable and maintainable React applications. By using tools like Jest and React Testing Library, and employing testing strategies like unit, integration, and end-to-end testing, you can ensure that your components and applications are in tip-top shape. Happy testing!