React Intro
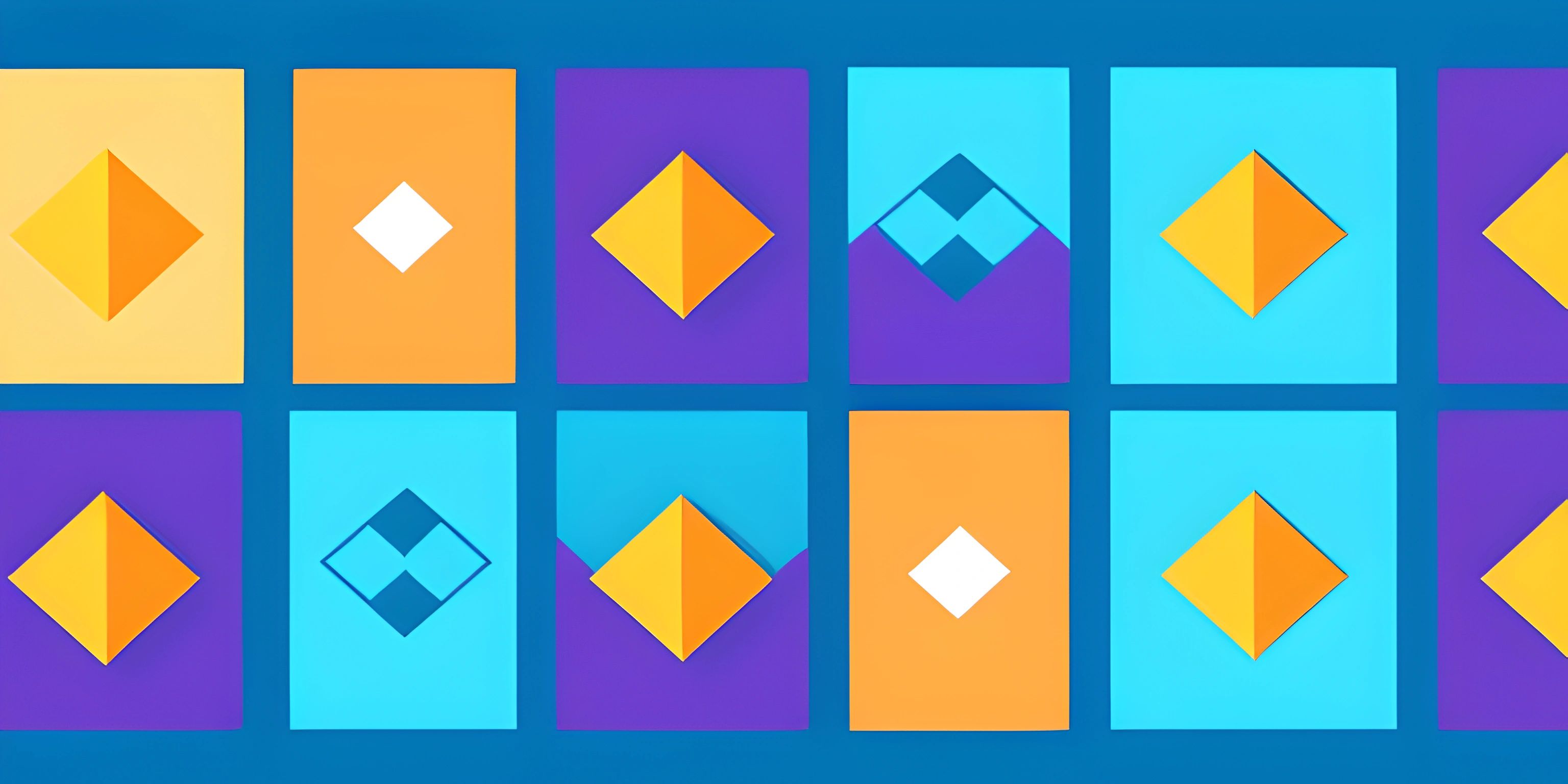
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
React is a popular JavaScript library for building interactive user interfaces (UIs) on the web. Developed by Facebook, it allows developers to create modular, reusable components that manage their own state and interact with each other. Whether you're building a simple website or a complex web application, React offers a powerful and flexible way to manage your UI.
React Features
React brings a number of features to the table that make it a popular choice for developers.
Components
The building blocks of React applications are components. Components are reusable, self-contained pieces of code that define the appearance and behavior of a part of your application. They can be as simple as a button or as complex as a navigation menu. By combining components, you can create complex UIs with ease.
JSX
React introduces a syntax extension called JSX, which allows you to write HTML-like code within your JavaScript. This makes it easy to create and modify the structure of your components directly in your code. JSX is not required to use React, but it's highly recommended as it makes writing React code more intuitive.
function WelcomeMessage() { return ( <div> <h1>Hello, world!</h1> <p>Welcome to our website.</p> </div> ); }
State Management
React uses a concept called state to manage the data within your application. Components can have their own local state, and you can also use global state management libraries like Redux to manage the state across your entire application. By managing state effectively, you can create highly interactive applications with ease.
Virtual DOM
React uses a virtual representation of the Document Object Model (DOM) to optimize UI updates. When the state of a component changes, React calculates the difference between the current and new virtual DOMs and updates only the parts of the real DOM that need to change. This process, called reconciliation, improves the performance of your application.
Getting Started with React
To start using React in your project, you can include it using a script tag or install it using npm or yarn:
<!-- Include React from a CDN --> <script src="https://unpkg.com/react@17/umd/react.production.min.js"></script> <script src="https://unpkg.com/react-dom@17/umd/react-dom.production.min.js"></script>
# Install React using npm npm install react react-dom # Install React using yarn yarn add react react-dom
Once you've added React to your project, you can start creating components and rendering them to the DOM. Here's a simple example of a React component that displays a welcome message:
import React from 'react'; import ReactDOM from 'react-dom'; function WelcomeMessage() { return ( <div> <h1>Hello, world!</h1> <p>Welcome to our website.</p> </div> ); } ReactDOM.render(<WelcomeMessage />, document.getElementById('root'));
In this example, we import the necessary React libraries, define a simple functional component, and use ReactDOM.render() to display the component in an HTML element with the ID "root".
Conclusion
React is a powerful and versatile library for building interactive web applications. By leveraging its features like components, JSX, state management, and the virtual DOM, you can create dynamic and efficient UIs with ease. Dive deeper into React by exploring more advanced topics like React hooks and React context. The world of web development awaits!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Full-stack Web Frameworks (Next.js) (psst, it's free!).
FAQ
What is React and what are its main features?
React is a popular JavaScript library for building interactive and dynamic web applications. Its main features include:
- Declarative programming: React makes it easy to create interactive UIs with a clear and concise syntax that focuses on expressing the desired end state.
- Component-based architecture: React encourages the creation of reusable components, making it simple to manage complex applications.
- Fast and efficient updates: React uses a virtual DOM (Document Object Model) to efficiently update the actual DOM, which results in better performance and smoother user experiences.
How do I create a React component?
To create a React component, you can use a class component or a functional component. Here's an example of a simple functional component:
import React from 'react'; function Welcome(props) { return <h1>Hello, {props.name}</h1>; } export default Welcome;
And here's an example of a class component:
import React, { Component } from 'react'; class Welcome extends Component { render() { return <h1>Hello, {this.props.name}</h1>; } } export default Welcome;
What is JSX and how is it used in React?
JSX (JavaScript XML) is a syntax extension for JavaScript that allows you to write HTML-like code within your JavaScript code. It's used in React to create and define the structure and appearance of the UI components. For example:
function Welcome(props) { return <h1>Hello, {props.name}</h1>; }
In this example, the JSX code <h1>Hello, {props.name}</h1>
is used to define a simple Welcome
component that displays a greeting with the user's name.
What are the benefits of using React for web development?
React offers numerous benefits for web development, such as:
- Improved performance: React uses a virtual DOM to optimize updates, resulting in faster and more efficient performance.
- Reusable components: The component-based architecture encourages code reuse, making it easier to manage large applications and maintain a consistent UI.
- Strong community and ecosystem: React has a large, active community and a rich ecosystem of tools, libraries, and resources to help you build and optimize your applications.
- Easy integration: React can be easily integrated with other libraries and frameworks, such as Redux for state management, and can be used in existing applications.
How do I get started with React?
To get started with React, you can use the Create React App tool that sets up a new React project with a sensible default configuration. Simply follow these steps:
- Install Node.js and npm (Node Package Manager) if you haven't already.
- Open your terminal or command prompt and run the following command to install Create React App globally:
npm install -g create-react-app
- Create a new React project by running:
create-react-app my-app
(replace "my-app" with your desired project name) - Navigate to the project folder using
cd my-app
- Start the development server by running
npm start
Your new React app should now be running in your web browser athttp://localhost:3000/
. You can start editing the code in thesrc
folder to create your application.