React State Management
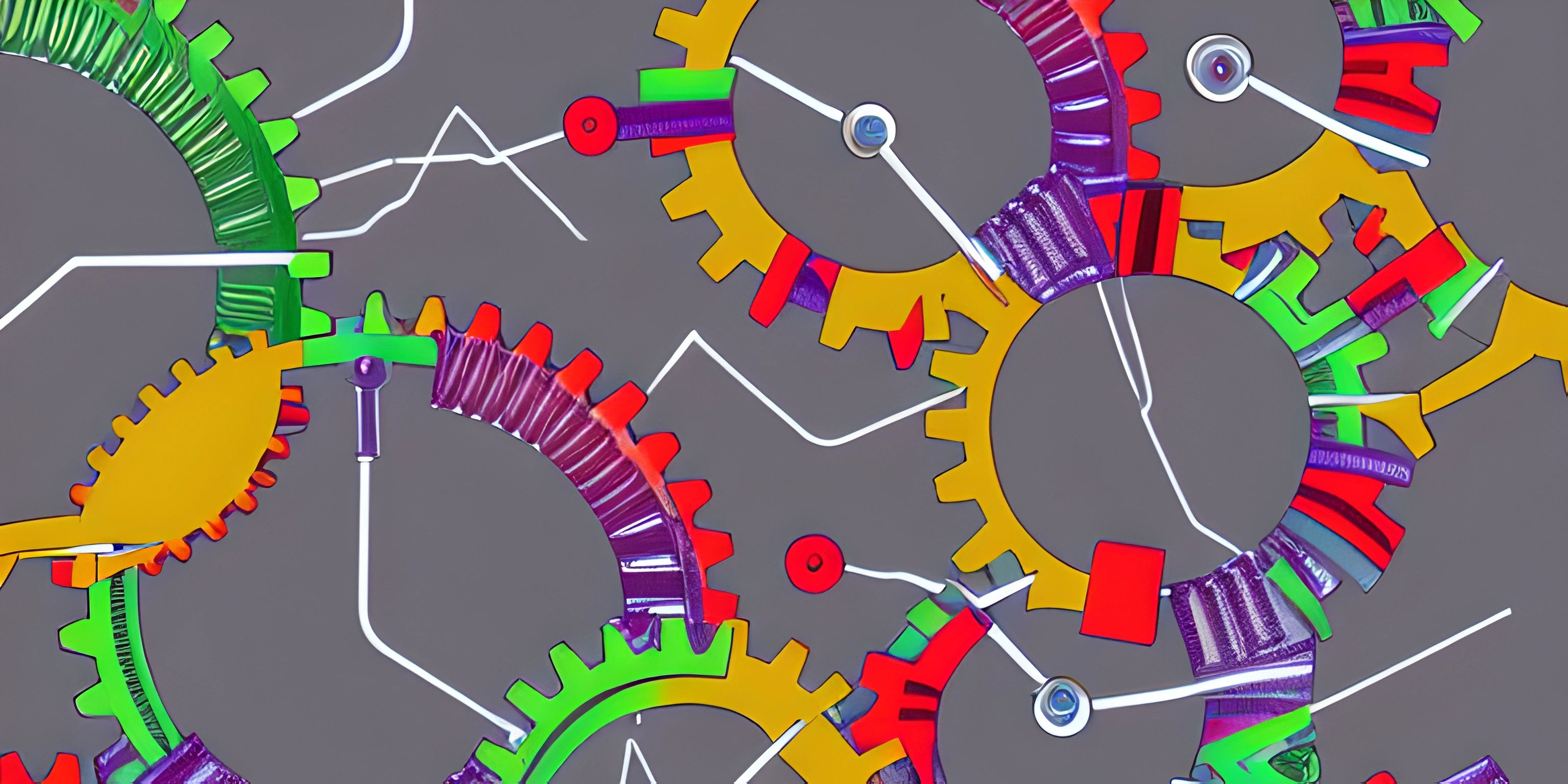
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
State management is one of the key concepts you'll need to grasp when developing applications with React. In React, state refers to the data that drives the behavior and appearance of your components. Proper management of this data is essential to ensure a smooth user experience and maintainable codebase.
Local Component State
React components can have their own internal state, known as local component state. You might use local state to manage things like form inputs, button toggles, and other UI-specific data. To manage local state in a React component, you can use React's built-in useState
hook.
Here's a simple example of using local state to manage the text of an input field:
import React, { useState } from "react"; function MyInput() { const [inputText, setInputText] = useState(""); const handleChange = (event) => { setInputText(event.target.value); }; return ( <input type="text" value={inputText} onChange={handleChange} /> ); }
In this example, the useState
hook initializes inputText
with an empty string and provides a function, setInputText
, to update the state. The input field's value is controlled by the inputText
state, and any changes to the field invoke the handleChange
function, which updates the state using setInputText
.
External State Management Libraries
While local component state is useful for managing small amounts of UI-specific data, larger applications often require a more centralized and robust solution for managing state. This is where external state management libraries come in. These libraries help you manage application state more efficiently by providing tools and patterns to handle complex state management scenarios.
Here are a few popular state management libraries for React applications:
Redux
Redux is a popular state management library that provides a centralized store for your application's state. It uses a pattern known as Flux to handle actions and reducers, which are responsible for updating the state. Redux is particularly useful for complex applications with a large number of state updates and actions.
MobX
MobX is another state management library that focuses on making it simple to manage your application's state using observable properties and actions. It provides a more reactive approach to state management, making it easier to reason about data flow in your application.
Recoil
Recoil is a relatively new state management library developed by Facebook, the creators of React. Recoil introduces the concept of atoms and selectors to manage state in a more granular and scalable way. It is designed specifically for React applications and provides a simple API for managing state that feels familiar to React developers.
Choosing the Right State Management Solution
Selecting the right state management solution for your React application largely depends on the complexity of your application and your personal preferences as a developer. It's essential to evaluate the trade-offs and benefits of different libraries and choose the one that best fits your needs.
Remember, you can always start with local component state and transition to an external state management library as your application grows and evolves. The key is to understand the state management patterns and libraries available and how they can help you manage your application's state effectively.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust Chat App Backend (psst, it's free!).