Smart Pointers Overview
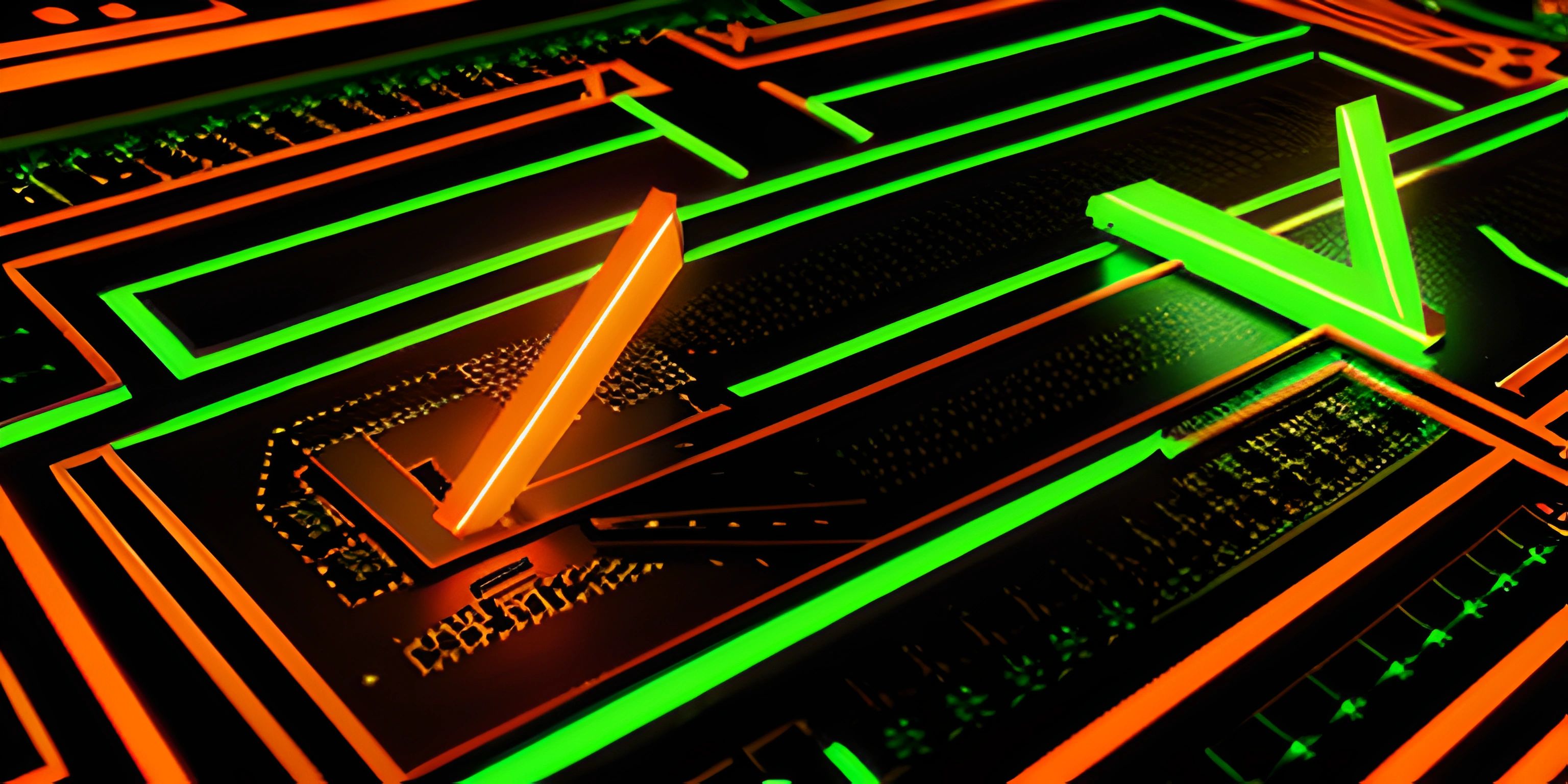
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Smart pointers are like little memory supervisors, ensuring that your programs behave responsibly when handling memory. They've got your back and help keep your code clean and efficient. So, let's dive into the world of smart pointers and see what they're all about!
What are Smart Pointers?
In programming, we often deal with pointers, which are variables that store memory addresses of other objects. However, working with raw pointers can be tricky and error-prone, leading to issues like memory leaks and dangling pointers.
Enter smart pointers! They're like having a memory management guardian angel, helping you avoid common pitfalls associated with pointers. Smart pointers are objects that automatically manage the memory of the objects they point to. They do this by keeping track of how many references exist to the object, and when the last reference goes out of scope, they clean up the memory. Neat, huh?
Benefits of Using Smart Pointers
1. Memory Safety
Smart pointers help ensure that your programs don't suffer from memory-related issues like memory leaks, double deletion, and dangling pointers. They take care of memory allocation and deallocation behind the scenes, allowing you to focus on the more important parts of your code.
2. Simplified Code
By handling memory management automatically, smart pointers allow you to write cleaner, more straightforward code. No more juggling memory allocation and deallocation yourself – smart pointers have got it covered!
3. Improved Performance
Smart pointers can help improve the performance of your programs by ensuring that memory is appropriately allocated and deallocated. This can lead to more efficient memory usage and reduced runtime overhead.
Types of Smart Pointers
There are several types of smart pointers, each with its own unique way of managing memory. Some common smart pointers include:
1. Unique Pointer
A unique pointer is a smart pointer that exclusively owns the object it points to. It ensures that only one reference to the object exists at any given time, and when the unique pointer goes out of scope, the object is automatically deleted.
2. Shared Pointer
As the name suggests, a shared pointer allows multiple references to the same object. It keeps track of the number of references and deallocates the memory when the last reference goes out of scope.
3. Weak Pointer
A weak pointer is a smart pointer that holds a non-owning reference to an object managed by a shared pointer. It doesn't influence the reference count of the object and is used to break reference cycles, preventing memory leaks in certain situations.
Conclusion
Smart pointers are like having a memory management superhero on your side, ensuring that your code is efficient, clean, and safe. By understanding their benefits and how they work, you'll be well on your way to writing better programs and impressing your fellow programmers!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Rust - A Language You'll Love (psst, it's free!).
FAQ
What are smart pointers and why are they useful?
Smart pointers are a type of pointer in programming languages that automatically manage the memory they point to. They're useful because they help prevent memory leaks and make it easier to write safe, efficient code. By automatically managing memory, programmers can focus on building the functionality of the application without having to worry about manual memory management.
How do smart pointers differ from regular pointers?
While both smart pointers and regular pointers store the address of an object, smart pointers go a step further by managing the memory of the object they point to. Smart pointers automatically delete the object when it's no longer needed, preventing memory leaks, while regular pointers require manual memory management. This makes using smart pointers less error-prone and easier to work with.
Can you give an example of a smart pointer in C++?
Sure! C++ provides several types of smart pointers, one of which is the shared_ptr
. Here's an example of how to use a shared_ptr
:
#include <iostream> #include <memory> class MyClass { public: MyClass() { std::cout << "MyClass constructor" << std::endl; } ~MyClass() { std::cout << "MyClass destructor" << std::endl; } }; int main() { std::shared_ptr<MyClass> ptr1(new MyClass()); // Automatically manages the memory of the MyClass object { std::shared_ptr<MyClass> ptr2 = ptr1; // ptr2 shares ownership of the MyClass object with ptr1 } // ptr2 goes out of scope, but the MyClass object is not deleted yet, as ptr1 still holds a reference to it return 0; } // ptr1 goes out of scope, and the MyClass object is automatically deleted
What are the main types of smart pointers in C++?
C++ provides three main types of smart pointers:
unique_ptr
: This smart pointer ensures that only one pointer owns the object at any given time. When theunique_ptr
goes out of scope, the object it points to is automatically deleted.shared_ptr
: This type of smart pointer allows multiple pointers to share ownership of an object. The object is automatically deleted when the lastshared_ptr
that owns it goes out of scope.weak_ptr
: Aweak_ptr
is a non-owning smart pointer that can be used to temporarily observe or access an object owned by ashared_ptr
. It doesn't contribute to the reference count of theshared_ptr
, so it doesn't prevent the object from being deleted.
How do smart pointers help with memory management in programming languages like Rust?
In Rust, the memory management is built around the concept of ownership and borrowing, which ensures memory safety without the need for a garbage collector. Smart pointers, like Rc
(reference counted) and RefCell
(mutable memory location with dynamically-checked borrow rules), play a crucial role in this process. They provide mechanisms for sharing and managing memory in a safe and efficient manner, making it easier to write reliable and performant code without worrying about manual memory management.