Entering the World of Vue.js
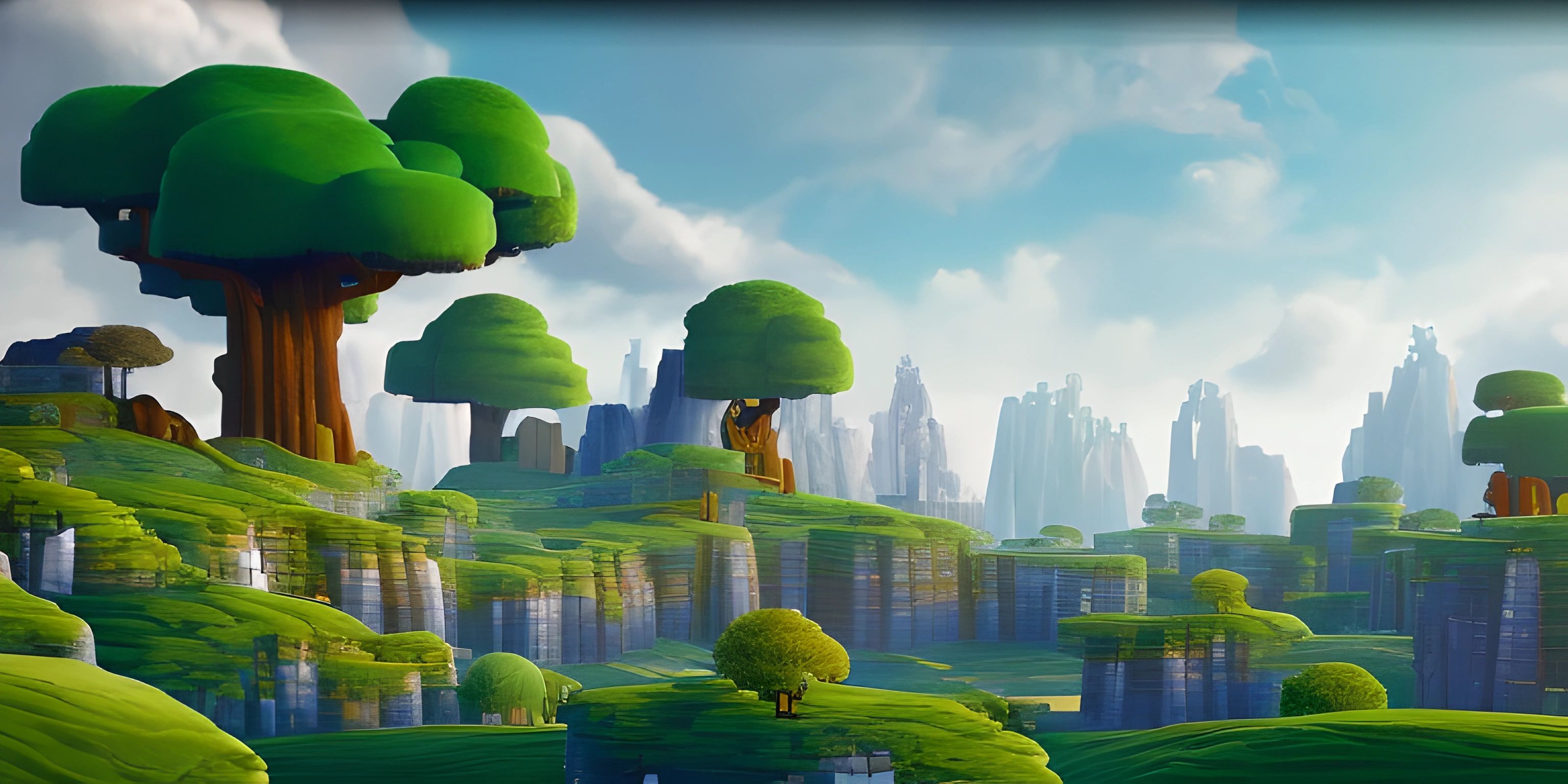
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Welcome to the world of Vue.js, a popular JavaScript framework for building lightning-fast, reactive web applications. As you dive into this exciting world, you'll be introduced to core concepts and features that set Vue.js apart from other frameworks.
What is Vue.js?
Vue.js (commonly known as Vue) is an open-source JavaScript framework for building user interfaces (UIs) and single-page applications (SPAs). It was created by Evan You in 2014 and has since gained a large community of developers due to its simplicity, flexibility, and powerful capabilities.
Core Concepts
Before we start creating amazing Vue applications, let's get familiar with some core concepts that Vue.js revolves around.
Components
In Vue.js, components are the building blocks of your application. A component is a self-contained, reusable piece of UI that can be nested and composed to create a complete web application. Components allow you to break down complex UIs into smaller, more manageable pieces.
Here's a simple example of a Vue component:
<template> <div> <h1>{{ title }}</h1> <p>{{ description }}</p> </div> </template> <script> export default { data() { return { title: "Entering the World of Vue.js", description: "An introduction to Vue.js and its core concepts." }; } }; </script>
In this example, we have a component with a title
and a description
that are both displayed within the component's HTML template. The component's data is defined within the data()
function.
Directives
Directives are special attributes in Vue templates that apply reactive behavior to the DOM. They're prefixed with v-
and allow you to manipulate the DOM based on the component's data. Some common directives include v-if
, v-for
, and v-bind
.
Here's an example using the v-for
directive to display a list of items:
<template> <ul> <li v-for="item in items" :key="item.id"> {{ item.name }} </li> </ul> </template> <script> export default { data() { return { items: [ { id: 1, name: "Item One" }, { id: 2, name: "Item Two" }, { id: 3, name: "Item Three" } ] }; } }; </script>
In this example, we use the v-for
directive to loop through the items
array and render a list item for each element.
Reactivity
One of Vue's most powerful features is its reactivity system. Reactivity means that when the data of a component changes, the DOM is automatically updated to reflect those changes. This reactivity allows you to create dynamic UIs with minimal effort.
Here's a simple example demonstrating Vue's reactivity:
<template> <div> <input v-model="name" placeholder="Enter your name" /> <p>Hello, {{ name }}!</p> </div> </template> <script> export default { data() { return { name: "" }; } }; </script>
In this example, we use the v-model
directive to bind the input field to the name
data property. As the user types, the name
data property is updated, and the greeting message is automatically updated as well.
Getting Started with Vue.js
To start building applications with Vue.js, you can simply include the Vue.js library in your HTML file, or you can use the Vue CLI to create a new Vue project with a more sophisticated build system and development environment.
As you embark on your Vue.js journey, you'll encounter a variety of technologies and libraries that complement Vue, such as Vuex for state management and Vue Router for client-side routing.
Now that you have a solid understanding of Vue.js's core concepts, you're ready to explore this powerful framework and build amazing web applications. Good luck, and happy coding!
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Full-stack Web Frameworks (Next.js) (psst, it's free!).