JavaScript Introduction
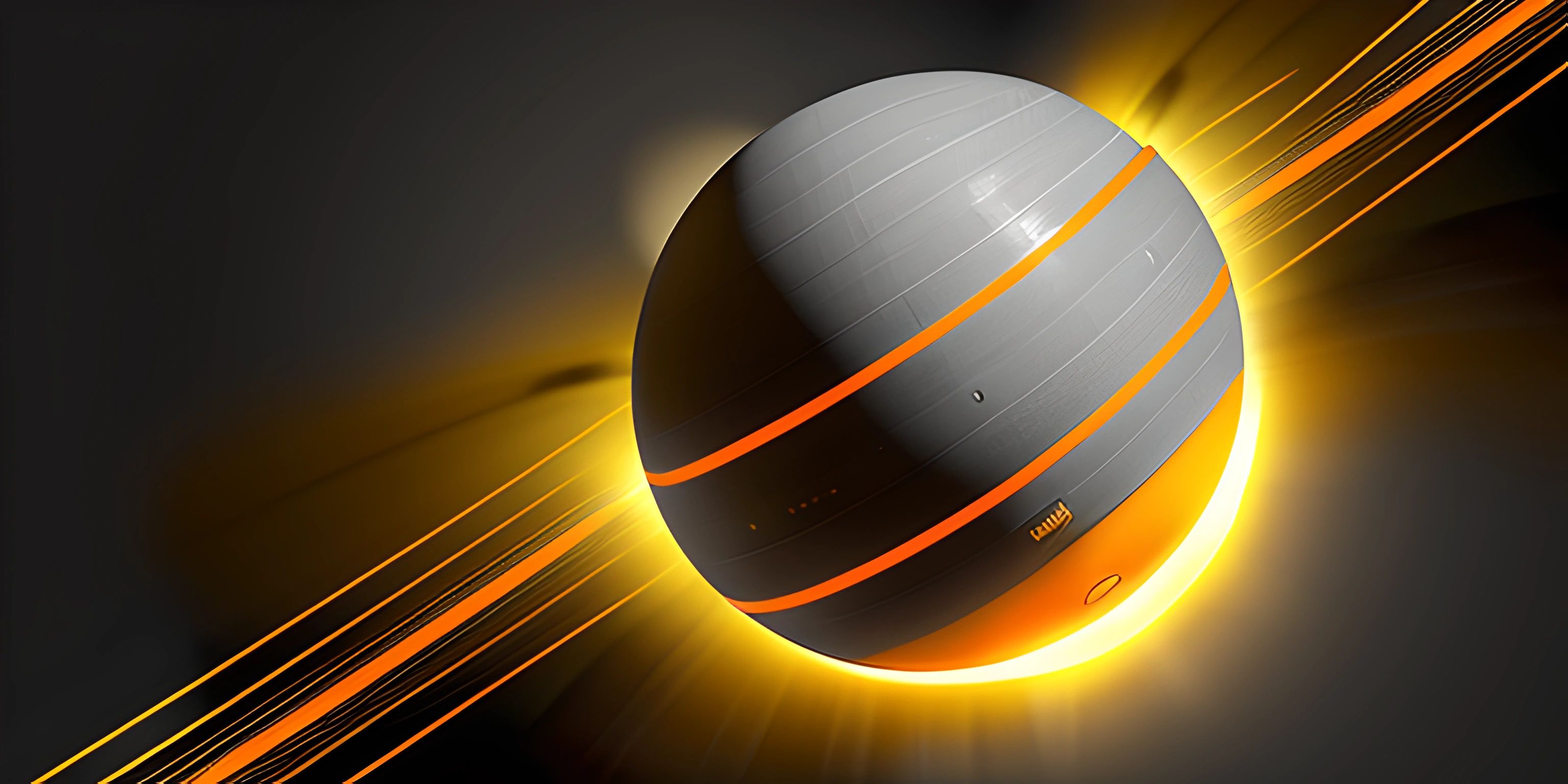
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
JavaScript, the swiss army knife of programming languages, is here to make your websites pop with interactivity, animation, and dynamic content. It's like your website just got a new pair of dancing shoes and is ready to boogie! 🕺
A Brief History
JavaScript was created in just 10 days in 1995 by Brendan Eich, who was working for Netscape at the time. It was originally called Mocha, then LiveScript, and finally JavaScript - borrowing some of Java's fame but, plot twist, having no direct relation to it.
Despite the rushed birth, JavaScript has evolved to become an essential part of the web. Along with HTML and CSS, it forms the holy trinity of front-end web development.
What Can JavaScript Do?
JavaScript brings life to your website by adding interactivity, handling user input, and making requests to APIs. It's like the puppeteer behind the curtains, pulling the strings to make your site dance, sing, and respond to user actions.
Here are some examples of JavaScript's magical powers:
- Change the content of an HTML element
- Show or hide elements based on user input
- Validate form data before submission
- Create and manipulate arrays and objects
- Animate elements, like fading in or sliding out
- Fetch data from a server and display it on your site
Running JavaScript
JavaScript code can be embedded directly into HTML using the <script>
tag or placed in separate .js
files, which can be linked to the HTML document.
Here's an example of a simple JavaScript code snippet embedded in an HTML file:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>My First JavaScript</title> </head> <body> <button onclick="showAlert()">Click me!</button> <script> function showAlert() { alert("Hello, World!"); } </script> </body> </html>
When you click the button, the showAlert
function is called and a popup saying "Hello, World!" appears.
Variables and Data Types
In JavaScript, we have variables to store data. Variables are like little boxes that can hold information. Here's how to declare a variable:
let myVariable = "Hello, JavaScript!";
JavaScript has a few basic data types, such as strings, numbers, booleans, and more complex ones like objects and arrays. You can learn more about them in this JavaScript Data Types article.
Functions
Functions in JavaScript are like recipes that perform specific tasks. You can write your own functions, or use built-in ones provided by the language. Here's a simple example of a function:
function greet(name) { console.log("Hello, " + name); } greet("JavaScript"); // Output: Hello, JavaScript
JavaScript functions can also be anonymous or arrow functions.
JavaScript Libraries and Frameworks
As JavaScript's popularity grew, developers created libraries and frameworks to make it even more powerful and easier to use. Some famous examples include jQuery, React, Angular, and Vue.js. These tools help in writing less code and achieving more functionality.
Conclusion
JavaScript is the heart and soul of modern web development, breathing life into websites and making them interactive, dynamic, and engaging. As you delve deeper into the world of JavaScript, you'll uncover more of its power and versatility. It's time to unleash your creativity and make your websites dance to the rhythm of JavaScript! 💃🕺
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Making Websites (psst, it's free!).
FAQ
What is JavaScript and why is it popular for web development?
JavaScript is a versatile and widely-used programming language that allows web developers to add interactivity and dynamic content to websites. It's popular for web development because it runs directly in the browser, making it easy for developers to create user-friendly and engaging web experiences without the need for additional plugins or software.
Can I use JavaScript alongside HTML and CSS?
Absolutely! In fact, JavaScript is often used in combination with HTML and CSS to create fully-featured web applications. HTML provides the structure of the webpage, CSS controls the appearance, and JavaScript adds interactivity and dynamic content. By using these three technologies together, developers can create rich and engaging web experiences.
How do I include JavaScript in my HTML file?
There are two common ways to include JavaScript in your HTML file: inline and external. To use inline JavaScript, you can include your code directly within the HTML file using the <script>
tag:
<script> console.log("Hello, World!"); </script>
For external JavaScript, you can save your code in a separate file with a .js
extension and link to it in your HTML file using the <script>
tag's src
attribute:
<script src="myScript.js"></script>
What are some basic JavaScript concepts that I should know?
As a beginner, it's important to understand some fundamental concepts of JavaScript, such as:
- Variables: Used to store data, like numbers, strings, and objects.
- Data types: Including strings, numbers, booleans, objects, and more.
- Functions: Reusable pieces of code that perform specific tasks.
- Conditional statements: Allow your code to make decisions based on conditions.
- Loops: Enable you to repeatedly execute code blocks.
- Events: Actions or occurrences, like a user clicking a button, that your code can respond to.
Can I test and debug JavaScript code in my web browser?
Yes! Most modern web browsers have built-in tools to help you test and debug JavaScript code. For example, in Google Chrome, you can open the Developer Tools by pressing Ctrl + Shift + J
(Windows/Linux) or Cmd + Opt + J
(Mac). This will open the Console, where you can write and execute JavaScript code, as well as view any error messages or output generated by your code.