An Introduction to Automated Testing Tools
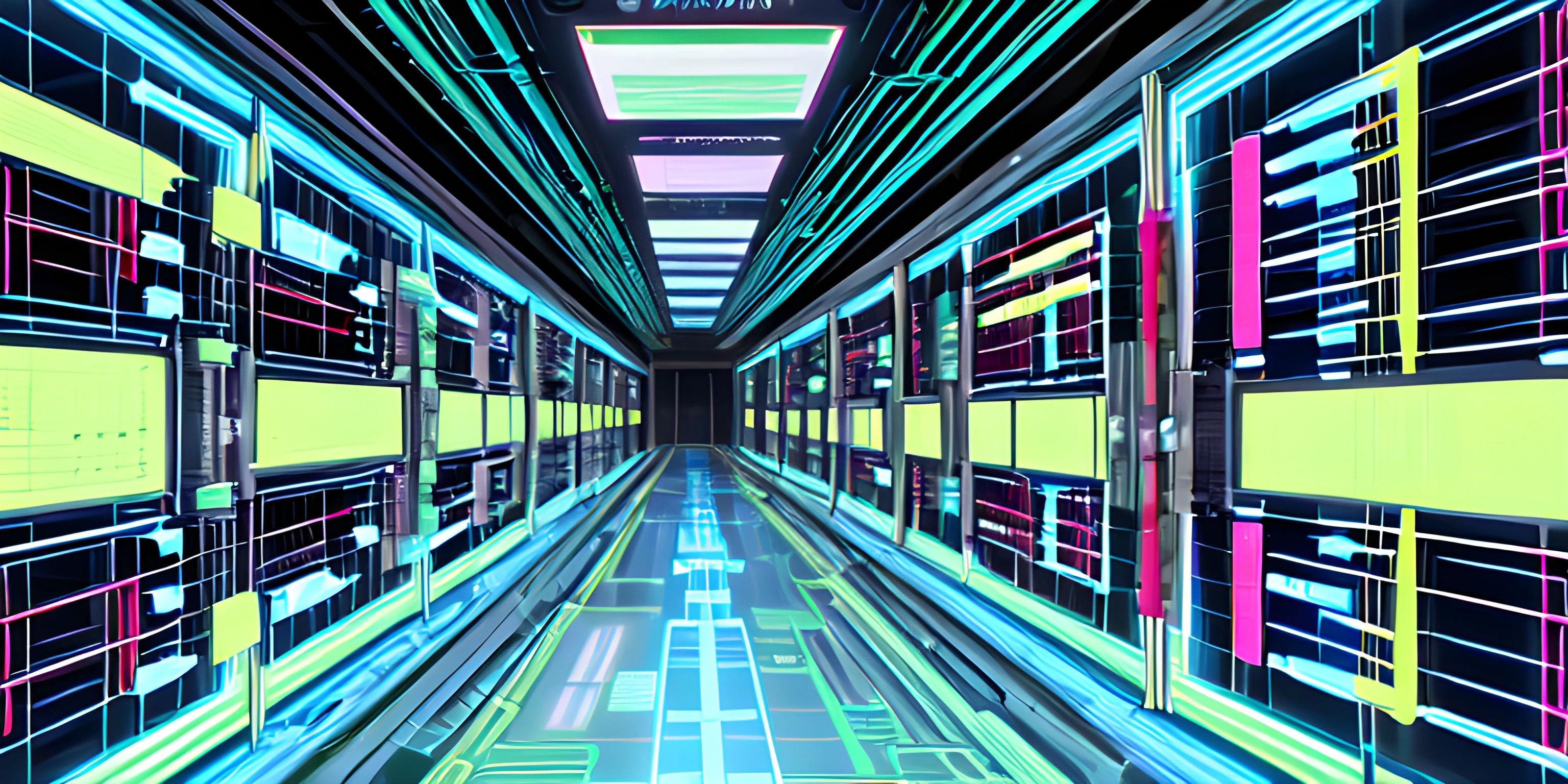
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Imagine you're building a massive Lego set without the instruction manual. You might get the castle's turret right, but the drawbridge could end up inside the moat. Now, imagine having a robot that checks each step you make, ensuring every piece is where it should be. That's what automated testing tools do for your code – they ensure every line fits perfectly into the grand structure of your software.
Automated testing tools are like these diligent robots. They help you catch mistakes early, save time, and ensure that your code works as expected. Let's dive into the different types of automated testing tools and explore their benefits.
Types of Automated Testing Tools
Unit Testing Tools
Unit testing focuses on individual components of the software, such as functions or methods. Think of it as checking each Lego block for defects before placing it onto your castle.
Some popular unit testing tools include:
- JUnit for Java
- NUnit for .NET languages
- pytest for Python
In unit testing, you write tests that verify the behavior of small sections of code. For example, if you have a function that adds two numbers, a unit test would ensure that add(2, 3)
returns 5
.
# Example using pytest def add(a, b): return a + b def test_add(): assert add(2, 3) == 5
Integration Testing Tools
Integration testing examines how different components of the software work together. It's like ensuring the drawbridge fits snugly with the turret on your Lego castle.
Popular integration testing tools include:
- Selenium for web applications
- Postman for API testing
- Apache JMeter for load testing
These tools help verify that your software's various parts interact smoothly. For instance, in a web application, you might want to ensure that the login module correctly communicates with the user database.
# Example using Selenium for a login test from selenium import webdriver driver = webdriver.Chrome() driver.get("http://example.com/login") username = driver.find_element_by_name("username") password = driver.find_element_by_name("password") login_button = driver.find_element_by_name("login") username.send_keys("user") password.send_keys("password") login_button.click() assert "Welcome" in driver.page_source driver.quit()
Functional Testing Tools
Functional testing evaluates the software against its functional requirements. It's like checking whether your Lego castle has a functioning drawbridge and can withstand a mini dragon attack.
Some functional testing tools are:
- QTP (QuickTest Professional) for a wide range of applications
- SoapUI for API testing
- FitNesse for acceptance testing
Functional testing ensures that the software behaves as expected. For example, in an e-commerce application, a functional test might verify that the "Add to Cart" button works correctly.
# Example using SoapUI for an API test import requests response = requests.get("http://example.com/api/product/1") assert response.status_code == 200 assert response.json()["name"] == "Product Name"
Performance Testing Tools
Performance testing assesses how well the software performs under specific conditions, such as heavy load or stress. It's like seeing if your Lego castle can withstand a stampede of mini knights.
Popular performance testing tools include:
- LoadRunner for performance and load testing
- Apache JMeter for performance testing
- Gatling for load testing
These tools measure response times, throughput, and resource usage. For example, you might want to test how your web application performs when 1,000 users try to log in simultaneously.
// Example using Apache JMeter for a load test import org.apache.jmeter.engine.StandardJMeterEngine; import org.apache.jmeter.save.SaveService; import org.apache.jmeter.util.JMeterUtils; import org.apache.jmeter.visualizers.Summariser; import org.apache.jorphan.collections.HashTree; public class LoadTest { public static void main(String[] args) throws Exception { // JMeter initialization (properties, log levels, locale, etc) JMeterUtils.loadJMeterProperties("jmeter.properties"); JMeterUtils.setJMeterHome("/path/to/jmeter"); JMeterUtils.initLocale(); // Load existing .jmx Test Plan SaveService.loadTree(new File("test-plan.jmx")); // Run JMeter Test StandardJMeterEngine jmeter = new StandardJMeterEngine(); jmeter.configure(testPlanTree); jmeter.run(); } }
Benefits of Automated Testing Tools
Speed and Efficiency
Automated testing tools can execute tests much faster than humans. Imagine having a robot build your Lego castle while you relax with a cup of tea. Automated tests can run overnight, covering hundreds or thousands of test cases, ensuring that you wake up to a bug-free morning.
Consistency
Human testers might miss bugs or make mistakes. Automated testing tools, on the other hand, perform the same steps accurately every time. It's like having a Lego-building robot that never gets tired or distracted by shiny things.
Coverage
Automated tests can cover more scenarios and edge cases than manual testing. Think of it as having a robot that checks every nook and cranny of your Lego castle, ensuring no piece is out of place.
Early Bug Detection
Catching bugs early in the development process saves time and money. Automated tests can run continuously, providing instant feedback. It's like having a robot that spots a misplaced Lego piece before you build the entire castle on top of it.
Reusability
Automated tests can be reused across different projects and revisions. Once you create a test suite, you can use it to verify future updates. It's like having a blueprint that can be used to build multiple Lego castles.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Full-stack Web Frameworks (Next.js) (psst, it's free!).
FAQ
What are some common types of automated testing tools?
Common types of automated testing tools include unit testing tools (e.g., JUnit, pytest), integration testing tools (e.g., Selenium, Postman), functional testing tools (e.g., QTP, SoapUI), and performance testing tools (e.g., LoadRunner, Apache JMeter).
Why is early bug detection important in software development?
Early bug detection saves time and money by catching issues before they become more significant problems. Automated tests provide instant feedback, allowing developers to address bugs promptly and maintain a higher quality of code.
How do automated testing tools improve consistency in testing?
Automated testing tools perform the same steps accurately every time, reducing the risk of human error. This consistency ensures that tests are reliable and that bugs are consistently identified.
Can automated tests cover more scenarios than manual testing?
Yes, automated tests can cover more scenarios and edge cases than manual testing. Automated tools can quickly execute a large number of tests, ensuring comprehensive coverage and identifying issues that might be missed in manual testing.
What is the advantage of reusing automated tests?
Reusing automated tests saves time and effort by allowing the same test suite to be used across different projects and revisions. This reusability ensures that new updates are verified against existing tests, maintaining the quality and reliability of the software.