Cross-Site Scripting (XSS) Explained
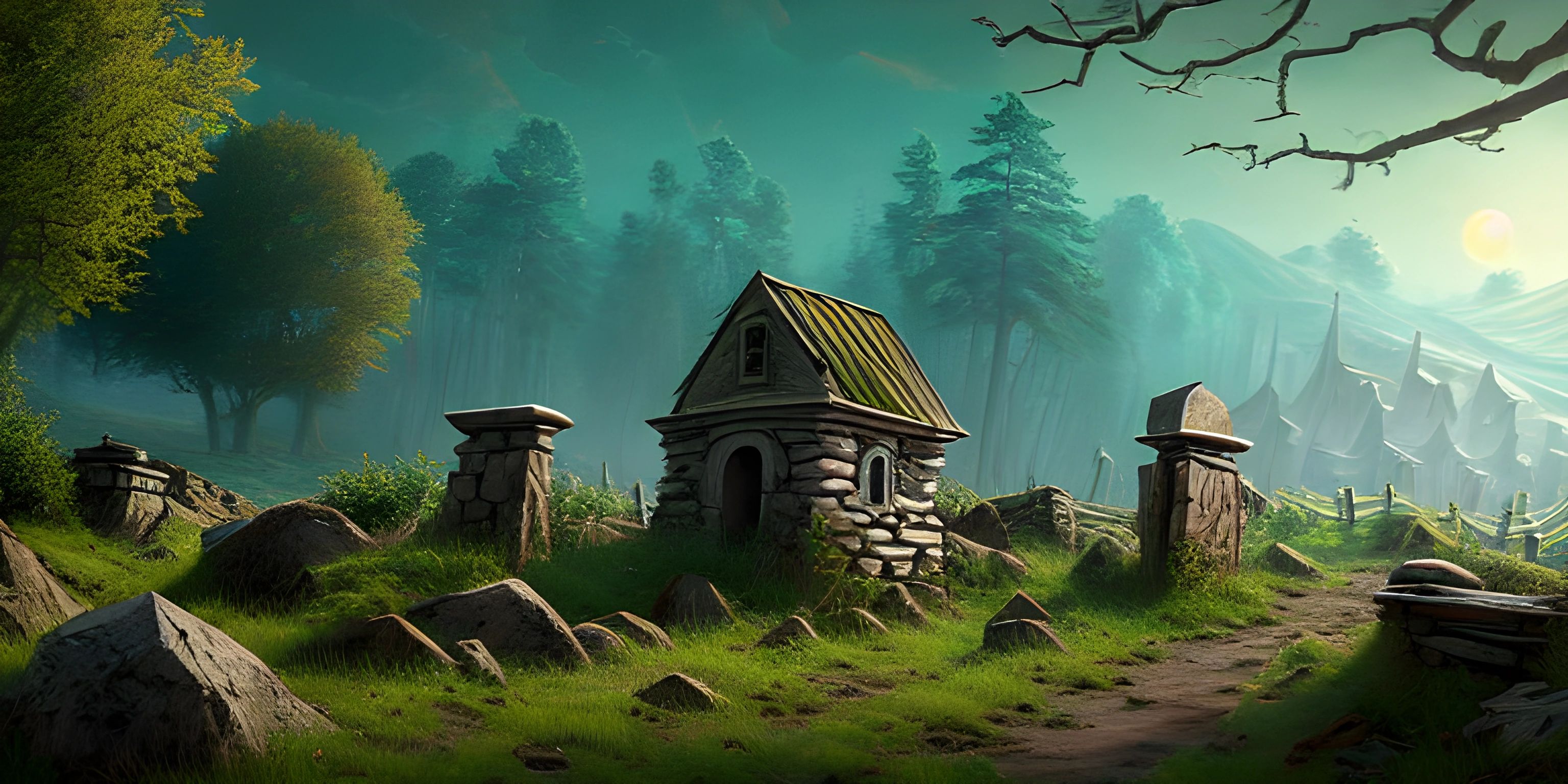
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Imagine you're at a potluck, excited to try everyone's dishes, when suddenly you realize someone has mixed poison into the food. That's essentially what happens with Cross-Site Scripting (XSS) attacks in web applications. Intruders inject malicious scripts into web pages that unsuspecting users then interact with, leading to all sorts of chaos.
What is Cross-Site Scripting (XSS)?
Cross-Site Scripting (XSS) is a type of security vulnerability found in web applications. It allows attackers to inject malicious scripts into web pages viewed by other users. The scripts execute in the context of the user's browser, potentially leading to data theft, session hijacking, or defacement of the website.
There are three main types of XSS attacks:
- Stored XSS
- Reflected XSS
- DOM-based XSS
Let's dive into each of these.
Stored XSS
Stored XSS, also known as Persistent XSS, occurs when malicious input is permanently stored on the target server, such as in a database, comment field, or message forum. When a user retrieves the stored information, the malicious script is executed in their browser.
Imagine a guestbook on a website where users can leave comments. An attacker might leave a comment that includes a script tag:
<script>alert("Hacked!");</script>
When another user views the guestbook, the script runs, displaying an alert box saying "Hacked!".
Reflected XSS
Reflected XSS happens when a malicious script is reflected off a web server and executed in the user's browser immediately. This often occurs through URL parameters or form submissions.
For example, an attacker might craft a URL like this:
http://example.com/search?q=<script>alert("Hacked!");</script>
When the victim clicks the link, the script runs in their browser, displaying the alert message.
DOM-based XSS
DOM-based XSS is a bit different. It occurs when the vulnerability is in the client-side code rather than the server-side code. The browser's Document Object Model (DOM) is manipulated to include malicious scripts.
For instance, JavaScript that takes user input and directly manipulates the DOM without sanitizing it can be exploited:
document.write(location.hash.substring(1));
If an attacker sets the URL to:
http://example.com/#<script>alert("Hacked!");</script>
The script executes in the victim's browser.
Identifying XSS Vulnerabilities
Identifying XSS vulnerabilities involves looking for places where user input is incorporated into the output. These can be in the form of query parameters, form fields, HTTP headers, or even cookies.
Manual Testing
Manual testing involves looking at the source code and identifying points where user input is not properly sanitized or encoded. This can include HTML elements, JavaScript functions, and AJAX calls.
Automated Tools
Several tools can help identify XSS vulnerabilities, such as:
- OWASP ZAP
- Burp Suite
- Netsparker
These tools can scan your application and highlight potential vulnerabilities.
Preventing XSS Attacks
Preventing XSS attacks involves proper input validation, output encoding, and using security headers.
Input Validation
Always validate and sanitize user input. This can include stripping out or escaping special characters that could be used to inject scripts. For example, using a library like DOMPurify to sanitize HTML input.
var clean = DOMPurify.sanitize(dirty);
Output Encoding
Always encode user input when displaying it. This ensures that characters like <
, >
, and &
are shown as their HTML entity equivalents (<
, >
, &
), preventing them from being interpreted as code.
For example, in a JavaScript context:
<script> var userInput = "<script>alert('Hacked!');</script>"; document.getElementById("output").innerText = userInput; </script>
Content Security Policy (CSP)
Implementing a Content Security Policy (CSP) can help mitigate the impact of XSS attacks by restricting the sources from which scripts can be loaded.
Here's an example of a CSP header:
Content-Security-Policy: script-src 'self' https://trustedscripts.example.com
This policy only allows scripts from the same origin ('self'
) and trustedscripts.example.com
.
HTTPOnly and Secure Cookies
Using HTTPOnly and Secure flags for cookies can help protect against certain types of XSS attacks, especially those aimed at stealing session cookies.
Set-Cookie: sessionId=abc123; HttpOnly; Secure
The HttpOnly
flag ensures the cookie is not accessible via JavaScript, and the Secure
flag ensures it is only sent over HTTPS.
Sanitization Libraries
Use libraries designed for sanitizing input. For example, in PHP:
$clean = htmlspecialchars($userInput, ENT_QUOTES, 'UTF-8');
Real-World Example
Let's look at a real-world example of preventing XSS in a comment section of a website. Assume we have an HTML form for users to submit comments:
<form method="post" action="/submit-comment"> <textarea name="comment"></textarea> <button type="submit">Submit</button> </form>
On the server side, we need to sanitize the input before storing it:
if ($_SERVER["REQUEST_METHOD"] == "POST") { $comment = htmlspecialchars($_POST["comment"], ENT_QUOTES, 'UTF-8'); // Store $comment in the database }
When displaying the comments, ensure they are properly encoded:
echo "<p>" . $comment . "</p>";
This way, even if an attacker tries to inject a script, it will be displayed as text rather than executed.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Story Builder (psst, it's free!).
FAQ
What are the different types of XSS attacks?
There are three main types of Cross-Site Scripting (XSS) attacks: Stored XSS, Reflected XSS, and DOM-based XSS. Stored XSS involves storing malicious input on the server, Reflected XSS reflects malicious scripts off the server, and DOM-based XSS exploits vulnerabilities in client-side code.
How can I identify XSS vulnerabilities?
Identifying XSS vulnerabilities involves looking for points where user input is included in the output, such as query parameters, form fields, and HTTP headers. Manual testing and automated tools like OWASP ZAP, Burp Suite, and Netsparker can help in identifying these vulnerabilities.
What is a Content Security Policy (CSP)?
A Content Security Policy (CSP) is a security measure that helps prevent XSS attacks by restricting the sources from which scripts and other resources can be loaded. It is configured via HTTP headers and can significantly reduce the risk of malicious script execution.
How does output encoding prevent XSS attacks?
Output encoding prevents XSS attacks by converting special characters like <
, >
, and &
into their HTML entity equivalents (<
, >
, &
). This ensures that user input is displayed as text rather than interpreted as code.
What are HTTPOnly and Secure cookies?
HTTPOnly and Secure cookies are flags that enhance cookie security. The HttpOnly
flag prevents cookies from being accessed via JavaScript, and the Secure
flag ensures cookies are only sent over HTTPS connections. These flags help protect against certain types of XSS attacks, especially those aimed at stealing session cookies.