HTML & JavaScript OnClick Event
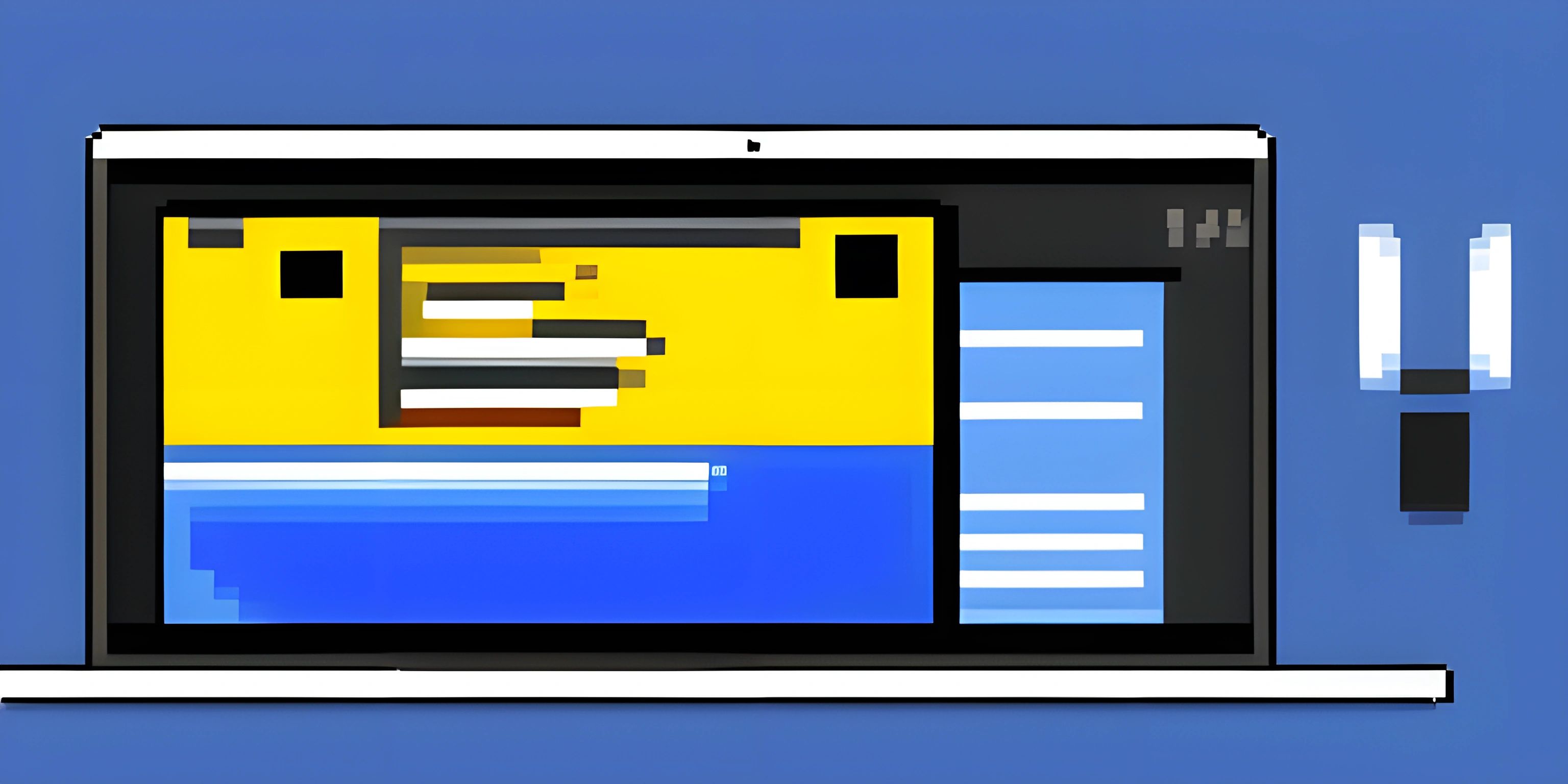
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
Programming interactive web pages often involves responding to user actions, such as clicks. The onclick
event is one of the most common ways to handle such interactions. In this example, we'll show you how to use the onclick
event to update the text of a button when it's clicked.
The OnClick Event
The onclick
event is an attribute that can be added to HTML elements, particularly buttons and links. It listens for a click event, and when it occurs, it executes the specified JavaScript function or code snippet.
Adding OnClick to an HTML Button
First, let's create an HTML button element and add the onclick
attribute to it:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>OnClick Event Example</title> </head> <body> <button id="myButton" onclick="updateButtonText()">Click me!</button> <script src="script.js"></script> </body> </html>
In the example above, we have a button with the text "Click me!". When the button is clicked, the JavaScript function updateButtonText()
will be called.
Creating the JavaScript Function
Now let's create the JavaScript function that will update the button text. Add the following code to a separate JavaScript file (e.g., "script.js"):
function updateButtonText() { const button = document.getElementById("myButton"); button.innerHTML = "You clicked me!"; }
In this function, we first use document.getElementById()
to get a reference to the button element. Then we update the innerHTML
property of the button element to change its text to "You clicked me!".
With the HTML and JavaScript in place, the button text will be updated when the button is clicked.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Using JavaScript (psst, it's free!).
FAQ
Can I use the `onclick` event with other HTML elements besides buttons?
Yes, you can use the onclick
event with various HTML elements, such as links, images, and even divs. However, it's most commonly used with buttons and links, as they are designed for user interaction.
Is `onclick` the only way to handle click events in JavaScript?
No, onclick
is just one way to handle click events. You can also use addEventListener()
to attach a click event listener to an element. This approach is more flexible and recommended for handling multiple event listeners on a single element.
Can I use inline JavaScript instead of a separate function for the `onclick` attribute?
Yes, you can use inline JavaScript code instead of a separate function. However, it's generally recommended to keep your JavaScript code separate from your HTML markup for better organization and maintainability.