Introduction to the Document Object Model
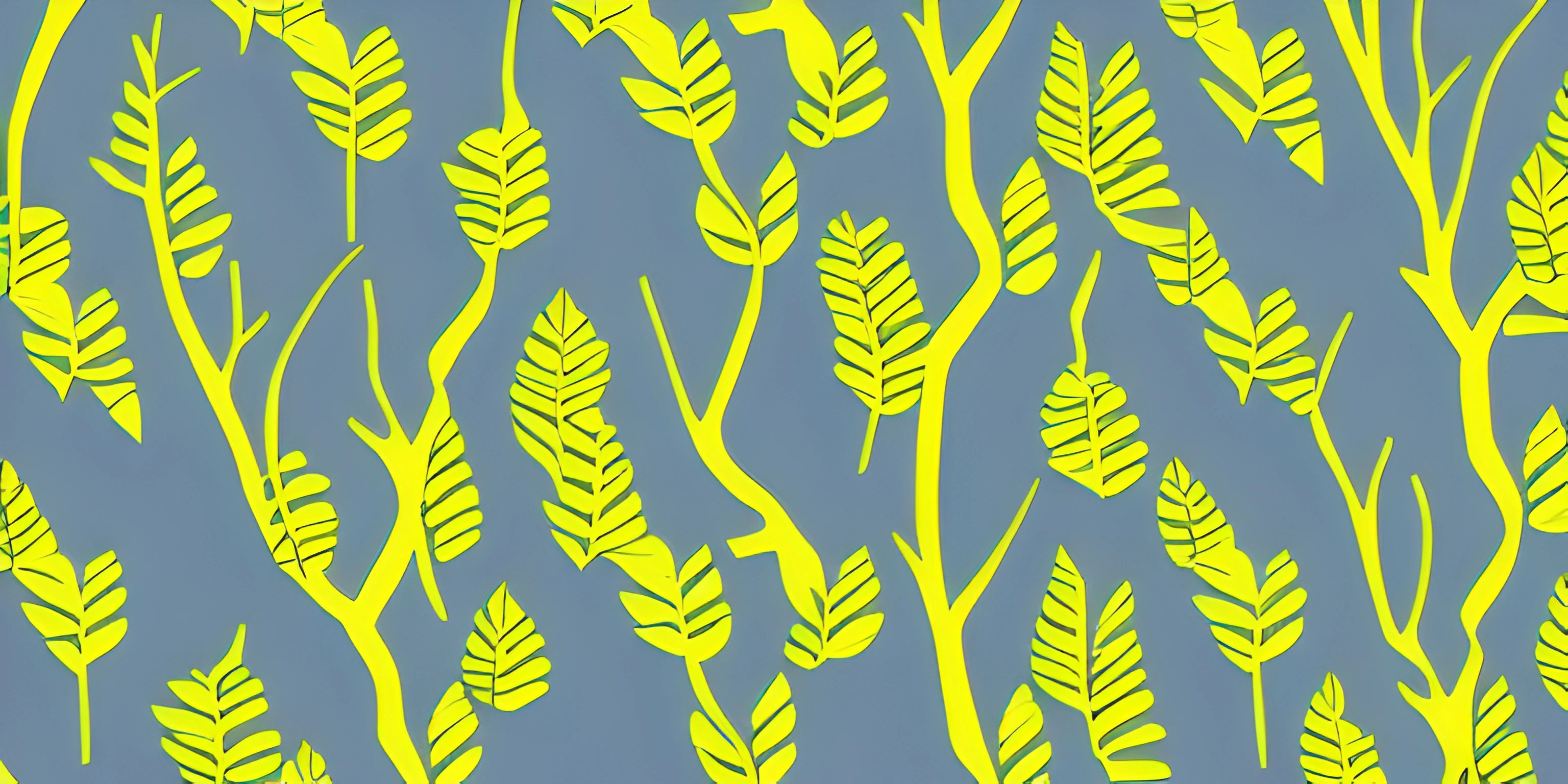
Note: this page has been created with the use of AI. Please take caution, and note that the content of this page does not necessarily reflect the opinion of Cratecode.
The Document Object Model (DOM) is a fundamental concept in web development, acting as a bridge between your HTML, CSS, and JavaScript. Think of it as a tree-like structure, with the webpage as its trunk, and each element branching out like a limb. This tree-like representation allows you to easily access, modify, and interact with the elements of a webpage using JavaScript.
What is the DOM?
The DOM is an in-memory representation of the structure of a webpage, created by the web browser when it loads an HTML document. It provides a way for JavaScript to access and manipulate the content, structure, and style of a website.
Each element on the page, such as headings, paragraphs, and images, is represented as a node in the DOM tree. Nodes can have parent-child relationships, just like in a family tree.
Let's look at a simple HTML example:
<!DOCTYPE html> <html> <head> <title>My First Webpage</title> </head> <body> <h1>Welcome to my site!</h1> <p>Here is some interesting content.</p> </body> </html>
In this example, the DOM tree would look something like this:
document
html
head
title
body
h1
p
The document
node is the root of the tree, with html
as its child. The html
node has two children, head
and body
, and so on.
Why is the DOM Important?
The DOM is crucial for web development for several reasons:
-
Accessing Elements: The DOM allows JavaScript to access and read elements on a webpage, giving you the power to retrieve values and data from user inputs, such as forms and buttons.
-
Manipulating Content: With the DOM, you can modify the content of a webpage dynamically. For instance, you can insert, update, or delete elements, change text, and even add or remove classes and styles.
-
Responding to Events: The DOM enables JavaScript to listen for and react to user interactions, like clicks, hovers, and keyboard input. These event listeners help create interactive and engaging user experiences on websites.
Getting Started with the DOM
To start working with the DOM, you'll need to learn how to access elements using JavaScript. Some of the most common methods include getElementById
, getElementsByClassName
, and querySelector
:
// Access an element by its ID attribute var myElement = document.getElementById("myId"); // Access elements by their class name var myElements = document.getElementsByClassName("myClass"); // Access an element using a CSS selector var myElement = document.querySelector("#myId .myClass");
Once you've accessed an element, you can manipulate its properties and methods to modify content, attributes, and styles, or add event listeners:
// Change the text content of an element myElement.textContent = "New content!"; // Add a CSS class to an element myElement.classList.add("newClass"); // Add an event listener to an element myElement.addEventListener("click", function() { alert("Element clicked!"); });
Now that you have a basic understanding of the Document Object Model and its importance, you're ready to dive deeper into the world of web development and create dynamic, interactive websites.
Hey there! Want to learn more? Cratecode is an online learning platform that lets you forge your own path. Click here to check out a lesson: Full-stack Web Frameworks (Next.js) (psst, it's free!).
FAQ
What is the Document Object Model (DOM)?
The DOM is an in-memory representation of the structure of a webpage, created by the web browser when it loads an HTML document. It provides a way for JavaScript to access and manipulate the content, structure, and style of a website. The DOM represents the webpage as a tree-like structure, with each element acting as a node in the tree.
Why is the DOM important?
The DOM is crucial for web development as it allows JavaScript to access and read elements on a webpage, modify the content of a webpage dynamically, and listen for and react to user interactions. This enables developers to create interactive and engaging user experiences on websites.
How can I access elements using JavaScript?
You can access elements using JavaScript with methods such as getElementById
, getElementsByClassName
, and querySelector
. These methods allow you to access elements by their ID attribute, class name, or using a CSS selector, respectively.